10 Essential Data Types You Need to Know in Programming

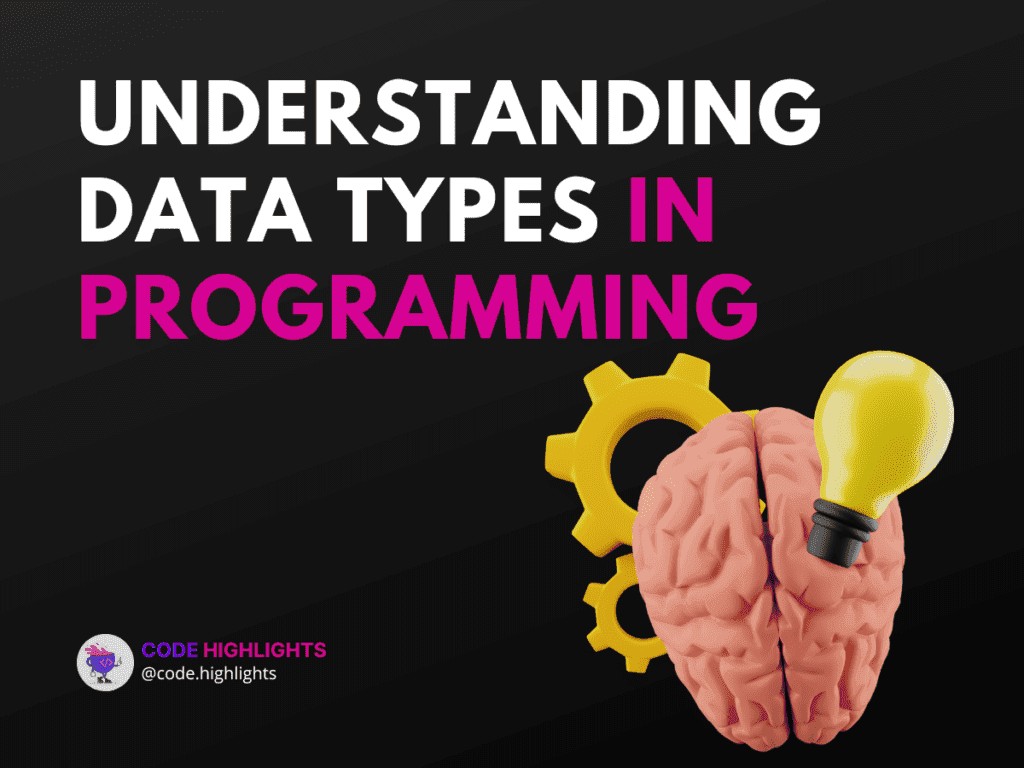
- Introduction
- Explanation of what data types are
- Importance of understanding data types in programming
- Basic Data Types
- Numbers (integer, float, double)
- Characters and Strings
- Boolean
- Examples of how each data type is used in programming
- Complex Data Types
- Arrays
- Structures
- Classes
- Explanation of how complex data types are used in programming
- Data Type Conversion
- Explanation of data type conversion
- Importance of data type conversion in programming
- Examples of data type conversion in different programming languages
- Conclusion
- Summary of key points
- Importance of understanding data types in programming
- Encouragement to continue learning about data types and other programming concepts
Introduction
Explanation of what data types are
Data types are a way to classify different types of data that can be stored and processed by a computer program. They serve as a way to identify the type of data being used and the operations that can be performed on it. Different programming languages may have different data types, but some common examples include numbers, characters, strings, and booleans.
Importance of understanding data types in programming
Understanding data types is crucial in programming as it allows developers to choose the appropriate type of data to store and manipulate. Using the correct data type ensures that the code will run efficiently and effectively. It also helps to prevent errors by ensuring that the data being stored and processed is of the correct type. Additionally, understanding data types are important when working with different programming languages, as the data types and their usage may vary between them.
Basic Data Types
Numbers (integer, float, double)
- Integers: Whole numbers, positive or negative, without a decimal point. Examples include -3, 0, and 100. They are used for counting and for representing discrete quantities.
- Floats: Numbers with decimal points. Examples include 3.14, -0.5, and 0.0. They are used for representing continuous quantities and for calculations that require a high degree of precision.
- Doubles: Similar to floats but with more precision. Often used for complex mathematical operations.
Characters and Strings
- Characters: Single letters or symbols, enclosed in single quotes. Examples include: ‘a’, ‘#’, ‘$’. They are used to represent individual characters in a program.
- Strings: A series of characters, enclosed in double quotes. Examples include: “Hello World”, and “abc123”. They are used to represent a sequence of characters and are commonly used for text data.
Boolean
A data type that can only have two values, true or false. Used for logical operations and decision-making in the code.
Examples of how each data type is used in programming
- Integers are used in calculations, such as addition, subtraction, multiplication, and division.
- Floats are used for representing decimal numbers, such as currency values or measurements.
- Characters and Strings are used for handling text data, such as user input or text displayed on a screen.
- Boolean values are used in decision-making, such as in an if statement to check if a certain condition is true or false.
Please let me know if you need me to explain anything further or if there is something else you would like me to add.
Complex Data Types
Arrays
An array is a collection of data of the same type, stored in a single variable. Arrays are used to store a large number of similar elements and can be accessed and manipulated using an index. The size of an array is fixed and determined when it is created. They can be one-dimensional, such as a list of integers, or multi-dimensional, such as a two-dimensional grid.
Structures
A structure is a collection of different data types, grouped together to form a single unit. Structures are used to store related information that belongs together and can be accessed using dot notation. They are similar to classes in that they can have properties and methods, but unlike classes, structures are value types and are stored on the stack rather than the heap.
Classes
A class is a blueprint for creating objects, which are instances of a class. Classes define a template for creating objects with specific properties and methods. Objects can be created from a class, and each object created has its own set of properties and methods. Classes are used to represent real-world entities, such as a bank account or a student.
Explanation of how complex data types are used in programming
- Arrays are commonly used in programs that need to store and manipulate large amounts of data of the same type, such as a list of items in a shopping cart or a list of student names in a class. They are also used for iterating over a set of data, such as displaying all the items in a list.
- Structures are used in programs that need to store related data together, such as the personal information of an employee including name, age, and address. They can also be used for storing data with a specific format, such as a date or a color.
- Classes are used in programs that need to represent real-world entities, such as a bank account or a student. They are used to create objects, which can have properties and methods that represent the characteristics and behavior of the entity they represent.
Data Type Conversion
Explanation of data type conversion
Data type conversion, also known as type casting, is the process of converting one data type to another. This can be done explicitly by the programmer or implicitly by the programming language. The conversion process ensures that the data being stored and processed is of the correct type.
Importance of data type conversion in programming
It is important in programming because it allows for flexibility and interoperability between different data types. For example, a variable of one data type may need to be used in a calculation or a comparison that requires a different data type. Data type conversion also allows for converting user input to a specific data type, ensuring that the input is valid before it is processed.
Examples of data type conversion in different programming languages
- In C#, data type conversion can be done using the (data type) notation, such as int x = (int) 3.14;
- In Python, data type conversion can be done using built-in functions like int(), float(), and str(). For example, int(“42”) will convert the string “42” to the integer 42.
- In Java, data type conversion can be done using methods of the corresponding wrapper classes like Integer.parseInt() and Double.parseDouble(). For example, Integer.parseInt(“42”) will convert the string “42” to the integer 42.
It’s also worth noting that in some cases, data type conversion may lead to loss of data, especially when converting from a higher precision data type to a lower precision one. For example, converting a float with a value of 1.23 to an integer will result in the value 1.
Javascript, for example, even if it’s one of the most popular modern web languages, doesn’t require writing data type.
Conclusion
Summary of key points
In this blog post, we discussed the concept of data types and their importance in programming. We covered the basic data types such as numbers, characters, and strings, and the complex data types such as arrays, structures, and classes. We also covered data type conversion and its importance in ensuring the correct data type is used for the desired operation.
The data type can also help you in bug debugging.
Importance of understanding data types in programming
The key points we covered include:
- Data types are a way to classify different types of data that can be stored and processed by a computer program.
- Understanding data types is crucial in programming as it allows developers to choose the appropriate type of data to store and manipulate, leading to more efficient and effective code.
- Complex data types such as arrays, structures, and classes are used for storing and manipulating more complex data in a program.
- Data type conversion is the process of converting one data type to another, it allows for flexibility and interoperability between different data types.
Encouragement to continue learning about data types and other programming concepts
Understanding data types is an essential part of programming, and it’s important to continue learning about different data types, their usage, and how they can be used effectively in different programming languages. It’s also important to continue learning about other programming concepts such as variables, functions, and control structures to become a proficient developer.
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
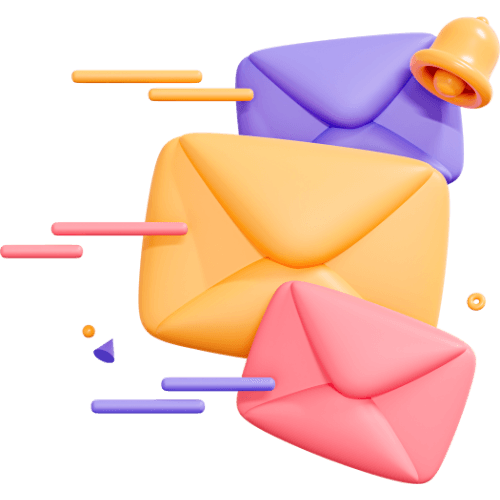
Related articles
5 Articles
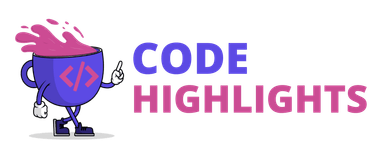
Copyright © Code Highlights 2025.