Master Git Version Control: 5 Essential Beginner's Tips

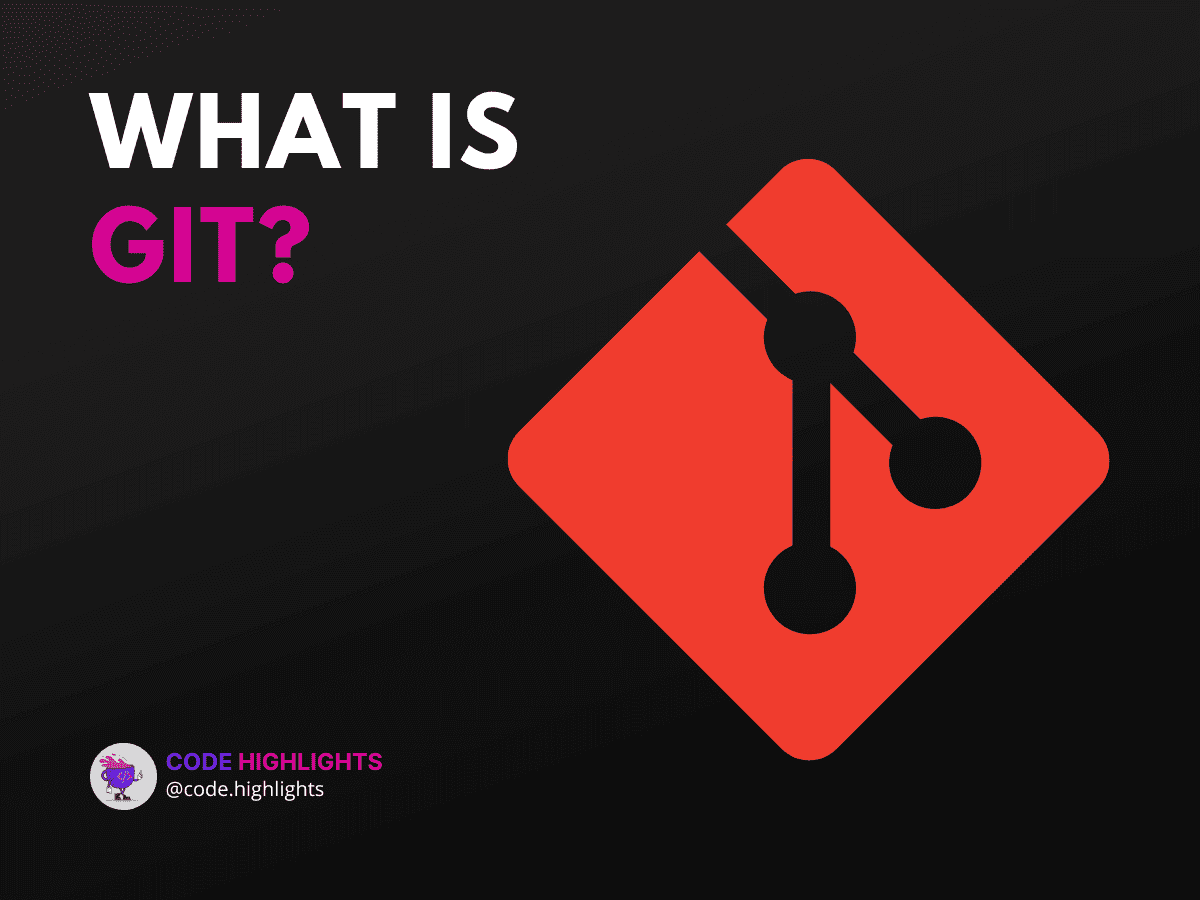
- Introduction
- Definition of Version Control Systems (VCS)
- Overview of Git as a VCS
- Importance of using a VCS
- What is Git and Why Should You Use it?
- How Git works
- Key concepts in Git
- Benefits of using Git as a VCS
- Setting up Git
- Installing Git
- Creating a repository
- Configuring Git
- Setting up a remote repository
- Basic Git Commands
- Git init
- Git clone
- Git add
- Git commit
- Git push
- Git pull
- Branching in Git
- Understanding branches in Git
- Creating and switching between branches
- Merging branches
- Resolving merge conflicts
- Collaborating with Git
- Sharing your repository
- Working with other people on a repository
- Reviewing changes and accepting or rejecting pull requests
- Advanced Git Features
- Git Hooks
- Git Rebasing
- Git Stash
- Git Bisect
- Conclusion
- References and Additional Resources
- Official Git Documentation
- Online tutorials and courses
- Books and Articles on Git and Version Control
- Further Reading
Introduction
Definition of Version Control Systems (VCS)
Several versions of source code, documentation, or any other digital content can be managed and tracked with the use of version control systems (VCS), which are computer programs. The basic goal of a VCS is to facilitate collaboration and long-term preservation of the material by enabling numerous contributors to work on the same piece of content and keeping track of all changes made.
Overview of Git as a VCS
Linus Torvalds developed Git, a well-liked and frequently used version management system, in 2005. It is a distributed version control system (VCS), which means that each contributor has a copy of the repository on their local PC. This makes it possible to work offline and increases efficiency. Git is a great choice for big, complicated projects because it is known to be fast, scalable, and reliable.
Importance of using a VCS
There are various benefits to using a VCS, including:
- Collaboration is better because more than one person can work on the same piece of content at the same time. Other contributors can just look over what they’re doing and add their changes.
- Enhanced productivity: A VCS makes it simple to compare different versions of the content to see what changes have been made and to roll back to a prior version of the content if something goes wrong.
- Improved security: VCS keeps track of every change made to the content and lets you go back to a previous version if you need to.
- Improved organization: VCS offers a central repository where all changes are tracked, which makes it simpler to track down material and its history.
What is Git and Why Should You Use it?
How Git works
Git functions by recording changes made to a collection of files that are kept in either a local or remote repository. Git creates a snapshot of the file in its current state when a change is made to it, together with a note describing the change, and stores this information as a commit. Commits are kept in chronological order and can be connected to create a branch, which is a series of modifications. Git only has one branch by default, called “master“, but more branches can be made for various features, bug fixes, or other uses.
Key concepts in Git
- Repository: A repository is a collection of files that also has a complete record of all file modifications. It is Git’s fundamental unit.
- Branch: Branches enable many streams of development to take place concurrently.
- Commit: A commit is a snapshot of the repository that shows a number of modifications that have been made to its files. Each commit has its own identifier and a message that describes the changes that were made.
- HEAD: The term “HEAD” refers to the most recent commit made to the active branch. It serves as a tool for determining the repository’s current state.
- Merge: Merging involves incorporating changes made in one branch into another branch. One branch is created by combining several branches into one.
Benefits of using Git as a VCS
- Distributed nature: Git makes it easier to work together and speeds things up by letting you work offline and keep a copy of the repository on your computer.
- Branching and merging: Git’s ability to branch and merge makes it easier to work on and manage many features at once.
- Ease of use: Even those with little technical knowledge may use Git easily thanks to its clear and straightforward user interface.
- Integration with other tools: Many tools, such as issue trackers, continuous integration platforms, and code review tools, can be connected with Git.
Setting up Git
Installing Git
Installing Git on your own computer is a prerequisite for using it. Installation steps will be different depending on your operating system, but the process is usually easy and quick.
Creating a repository
By going to the directory where your files are located and using the following command in your terminal after installing Git, you can create a new repository:
git init
This will create a new Git repository in the current directory.
Configuring Git
Git should be set up using your name and email address before you use it to ensure that your commits are appropriately credited:
$ git config --global user.name "Your Name"
$ git config --global user.email "your.email@example.com"
Setting up a remote repository
A remote repository is a Git repository that is stored on a server and can be accessed through the internet. GitHub is the most widely used site for hosting remote Git repositories, but there are a lot of other choices as well. You must first register for a GitHub account, then build a new repository, and finally use Git to push your local repository to the remote repository.
An example of how to push your local repository to a remote repository on GitHub is shown below:
$ git remote add origin https://github.com/user/repo.git $ git push -u origin master
Replace “user” with your GitHub username and “repo” with the name of your repository.
Basic Git Commands
Git is a command-line tool, and you can access the majority of its capabilities by using different commands. The most fundamental Git commands you’ll use frequently are listed below:
Git init
The git init command creates a new Git repository. You will execute it as the first command when creating a new repository.
git init
Git clone
It is possible to copy an existing repository using the git clone command. When you want to work on a project that has already been created and stored on a distant server, this is helpful.
git clone [https://github.com/user/repo.git](https://github.com/user/repo.git)
Replace “user” with the GitHub username of the repository owner and “repo” with the name of the repository.
Git add
To prepare modifications for a commit, use the git add command. Before you can commit changes you make to a file in your repository, you must first stage them using git add.
git add file.txt
Git commit
A new commit is made using the git commit command. The foundation of your repository’s history is made up of commits, which stand for a collection of modifications you’ve made to your files.
git commit -m "Add new file"
The -m option gives a message that describes the changes made by the commit.
Git push
To push changes from your local repository to a remote repository, use the git push command.
git push origin master
The name of the remote repository is specified by the “origin” option, and the name of the branch you’re pushing to is “master“.
Git pull
With the git pull command, you can get changes from a remote repository and put them in your local repository.
git pull origin master
Similar to git push, “master” is the name of the branch you’re pulling from, and the “origin” option is the name of the remote repository.
Branching in Git
One of Git’s most important features is that it lets you work on multiple versions of your project at the same time.
Understanding branches in Git
A branch in Git is nothing more than a pointer to a particular commit in the repository’s history. A new repository has one branch by default called “master,” but you can add as many as you require.
You can switch between branches as necessary to work on various aspects of your project; each branch represents a distinct path of development. When you’re ready, you can combine your work by merging modifications from one branch into another.
Creating and switching between branches
The git branch command can be used to add a new branch to Git. For instance:
git branch new-feature
This will produce the “new-feature” branch as a new branch. You can use the git checkout command to change to a different branch:
git checkout new-feature
Merging branches
The git merge command is used to merge changes from one branch with changes from another. To merge the “new-feature” branch into the “master” branch, for instance:
git checkout master
git merge new-feature
Most of the time, Git can automatically merge changes from one branch into another, but there can be disagreements.
Resolving merge conflicts
Git is unable to automatically integrate the conflicting changes when a merge conflict arises. The conflict must be manually addressed by modifying the impacted files, and git add must be used to mark the issue as resolved.
Run git commit to finish the merging once all conflicts have been resolved. Include a statement outlining the conflict resolution process in your commit message.
Collaborating with Git
Git was created to encourage teamwork and make it simple for several people to work on a project at once. Here’s how to collaborate with others using Git.
Sharing your repository
Hosting your repository on a remote server, like GitHub, is one of the simplest methods to make it accessible to others. With the git push command, you can either create a new repository on GitHub or push an existing one there:
git remote add origin https://github.com/username/repo.git
git push -u origin master
When your repository is hosted on a remote server, other users can copy it to their personal computers using the git clone command:
git clone [https://github.com/username/repo.git](https://github.com/username/repo.git)
Working with other people on a repository
When working on a repository with other people, it’s important to follow some basic best practices so that everyone’s changes can be easily merged.
For any new feature or bug repair, for instance, it’s a good idea to start a new branch so that your modifications don’t conflict with other people’s work. When your modifications are complete, you can merge them into the main branch.
To keep up with what others are doing, it’s also a good idea to often pull in updates from the remote repository.
Reviewing changes and accepting or rejecting pull requests
When working together on a GitHub project, you can use pull requests to examine and incorporate changes into the main branch.
When you want someone else to examine and incorporate your modifications into the main branch, you submit a pull request. On the GitHub page for the repository, click the “Pull Request” button to start a pull request.
Those who create pull requests can review the changes and comment on them. The pull request can be merged into the main branch after everyone is happy with the changes. The pull request can be denied and the changes adjusted if there are any problems with the changes.
Advanced Git Features
Git is meant to be simple and easy to use, but it also has some advanced features that can make working with your repositories more productive and efficient.
Some of the most practical advanced Git capabilities are listed below:
Git Hooks
Scripts known as “Git hooks” are launched automatically when particular Git commands are used. They can be used to automate various tasks, such as validating that code is formatted correctly or that tests are passing before changes are committed.
Git hooks are kept in your repository’s “.git/hooks” directory. By adding executable scripts to this directory, you can add new hooks.
Git Rebasing
You can reapply a series of commits onto a separate branch using git rebasing. When you wish to organize your commit history or incorporate changes from one branch into another, this can be helpful.
When using the git rebase
command, you may specify the branch to which you want to apply your changes again. The command then incrementally applies each of your commits to the new branch.
Git Stash
Using the Git stash
, you can temporarily save changes that you haven’t yet committed so that you can switch to a new branch or finish another task.
You can save your changes with the git stash command and then use the git stash apply command to reapply them.
Git Bisect
Git bisect
is a feature that makes it easy to find the change in your code that caused a bug.
The git bisect program examines each commit to see if the bug is there after splitting the history of your repository into two halves. Until the commit that caused the bug is discovered, this process is repeated.
Git bisect can be an effective debugging tool since it makes it possible to find the root of a fault even in vast and complex codebases.
Conclusion
Git, one of the most well-liked version control systems (VCS) on the market right now, has been covered in this article. The fundamentals of what Git is, how it functions, and how to use it successfully have been addressed.
One of the best things about using Git as a VCS is that you can easily work with other people, keep different code branches, and quickly go back to older versions of your code.
Overall, Git is a strong and adaptable technology that can be used to successfully and efficiently manage your code. Git is a tool that you should think about using, whether you’re working on a small-scale software development project or a large-scale one.
Online tutorials, guidelines, and video courses are just a few of the many resources accessible if you want to learn more about Git and how to use it efficiently. Also, there is a large and active community of people who use Git and are always willing to help and answer questions.
References and Additional Resources
If you want to learn more about Git and version control systems, you might want to look into some of the following:
Official Git Documentation
Git’s official website has a wealth of tutorials, reference materials, and other materials on how to use Git. If you’re new to Git and looking for a thorough reference, this is an excellent place to start.
Online tutorials and courses
You can find a wide variety of online tutorials and courses to help you learn Git, from simple introductory material to more complex subjects. Udemy, Coursera, and Pluralsight are a few well-known online educational resources that provide Git courses.
Books and Articles on Git and Version Control
There are several books and articles that discuss Git and version control if you prefer to learn through reading. “Pro Git” by Scott Chacon and Ben Straub and “Version Control with Git” by Jon Loeliger and Matthew McCullough are two well-liked books. Online, there are a ton of articles that cover different facets of Git and version control.
Further Reading
FAQs
What are the prerequisites for using Git as a VCS?
What are the prerequisites for using Git as a VCS?
The only requirement for utilizing Git as a VCS is having Git installed locally. The installation procedure is simple and quick to complete; depending on your operating system, the instructions may change.
How does Git keep track of changes made to a repository?
How does Git keep track of changes made to a repository?
Git captures the present state of the files, adds a message describing the change, and saves this information as a commit to maintain track of changes made to a repository. Commits are kept in chronological order and can be connected to create a branch, which is a series of modifications.
What is a remote repository in Git?
What is a remote repository in Git?
In Git, a “remote repository” is a Git repository that is kept on a server and can be accessed online. Although GitHub is the most widely used site for hosting remote Git repositories, there are other options.
How can I set up a remote repository in Git?
How can I set up a remote repository in Git?
You must first register for an account on a website like GitHub before setting up a remote repository in Git. You can use Git to push your local repository to the remote repository after the remote repository has been established.
What are some of the most basic Git commands that I’ll use regularly?
What are some of the most basic Git commands that I’ll use regularly?
You’ll frequently use the Git commands “git init” to create a new repository, “git clone” to duplicate an existing repository, “git add” to add changes to be committed, “git commit” to commit changes, and “git push” to push changes to a remote repository.
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
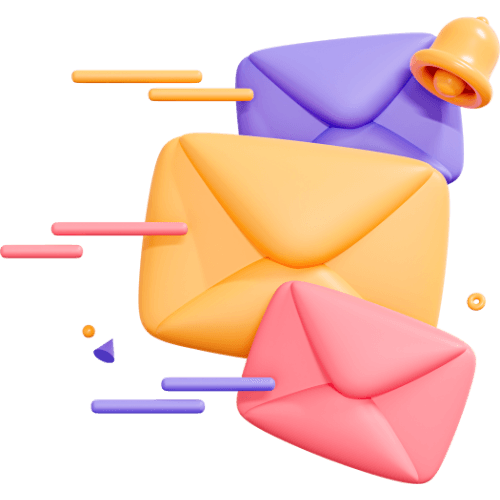
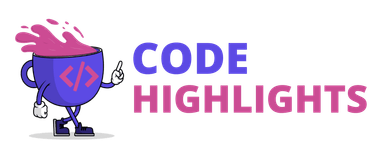
Copyright © Code Highlights 2025.