Debugging Common Coding Errors and Mistakes

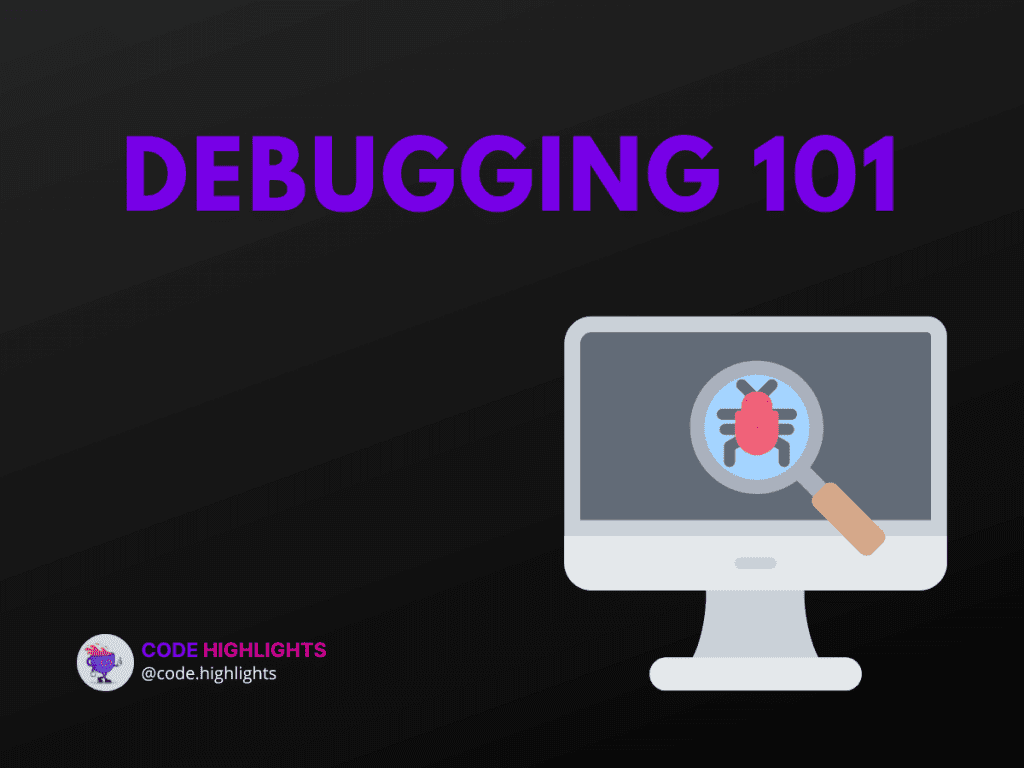
- Syntax Errors
- Logic Errors
- Logic Errors examples
- Tips for identifying logic Errors
- Runtime Errors
- Runtime Errors examples
- Tips for identifying Runtime Errors
- Best Practices for Debugging Common Coding Errors and Mistakes
- Using debugging tools
- Collaboration and seeking help from others
- Conclusion
In this blog, we will be talking about debugging Common Coding Errors and Mistakes: Best Practices and Techniques. Debugging is a crucial step in the software development process. It allows developers to identify and fix code errors for smooth program execution. Without debugging, a program may contain hidden bugs that can cause it to crash or produce inaccurate results.
Different types of coding errors: syntax, logic, runtime errors.
Syntax errors: computer cannot understand code. Logic errors: correct code but thewrong outcome. Runtime errors occur when the program is run, they can include problems such as memory leaks and infinite loops.
Understanding and being able to identify these types of errors is important for debugging and improving one’s coding skills.
Syntax Errors
They occur when the code is written in a way that the computer cannot understand. Syntax errors are caused by typing mistakes, incorrect punctuation, data types mismatch, and nonconformity to language rules.
Examples of syntax errors include:
- Forgetting a semicolon at the end of a line of code
- Using the wrong type of quotes around a string, such as using single quotes instead of double quotes
- Forgetting to close a bracket or parenthesis
- Using a variable without initializing it
Tips for identifying and correcting syntax errors:
- Use a code editor with syntax highlighting: Many code editors have built-in syntax highlighting that can help identify syntax errors by color-coding different parts of the code. This can make it easier to spot mistakes, such as missing punctuation or mismatched data types.
- Carefully review your code and compare it to similar examples: Sometimes, the best way to find syntax errors is to simply read through your code line by line and compare it to similar examples. This can help you identify errors that may have been overlooked by syntax highlighting.
- Check console or compiler output for error messages to identify and correct syntax errors. Error messages provide location and cause, making it easier to fix the problem.
- Check language documentation for understanding language rules and conventions.
- Use linting tools to automatically check code for syntax errors and common mistakes.
Logic Errors
Logic errors occur when the code is written correctly but produces the wrong output. These errors can be more difficult to identify and correct than syntax errors because the code will not produce error messages or crash. Instead, the program will run but produce unexpected or incorrect results. Logic errors are usually caused by mistakes in the program’s design or implementation, such as using the wrong mathematical formula or using an incorrect conditional statement.
Logic Errors examples
Examples of logic errors include:
- Using the wrong mathematical operator in a calculation
- Using an incorrect comparison operator in a conditional statement
- Using the wrong variable in a calculation or comparison
- Using an infinite loop when a finite loop is intended
Tips for identifying logic Errors
Tips for identifying and correcting logic errors:
- Understand the problem and the expected output: Before you start coding, make sure you understand the problem you are trying to solve and what the expected output should be. This will make it easier to identify when the program’s output is not what you expect.
- Print statements and testing: Use print statements to check the values of variables and expressions at different points in the program. This can help you identify where the logic error is and what is causing it.
- Use a debugging tool: Many code editors and IDEs have built-in debugging tools that allow you to step through the code line by line, set breakpoints, and check the values of variables. This can help you see exactly what the program is doing and identify where the logic error is.
- Revisit the problem and the design: Sometimes, the best way to fix a logic error is to revisit the problem and the overall design of the program. This can help you identify any mistakes in the program’s logic and come up with a new approach to solving the problem.
- Seek help from others: Collaboration and seeking help from others can be extremely valuable when trying to identify and correct logic errors. Other people may be able to spot mistakes or offer suggestions that you may not have considered.
Runtime Errors
Runtime errors occur when the program is executed and can include issues such as memory leaks and infinite loops. These errors can occur even if the code contains no syntax or logic errors. They can be caused by a variety of factors, such as a lack of memory, file permission issues, or incorrect user input.
Runtime Errors examples
Examples of runtime errors include:
- Attempting to access an array out of its bounds
- Attempting to open a file that does not exist
- Attempting to divide by zero
- Attempting to access a null object
- Attempting to use an uninitialized variable
- Memory leaks, where the program keeps allocating memory but not releasing it
Tips for identifying Runtime Errors
Tips for identifying and correcting runtime errors:
- Use a debugger: Debugging tools can help you identify and correct runtime errors by allowing you to step through the code and check the values of variables at different points.
- Use log statements: Logging statements can be useful in identifying and fixing runtime errors, as they can provide information about what the program is doing at the time of the error.
- Check for error messages: When the program encounters a runtime error, it may produce error messages that can help you identify the problem.
- Verify the environment: Ensure that the environment in which the program runs is configured correctly and has all the required dependencies
- Identify memory leaks: Utilize memory profilers to identify memory leaks, and ensure that the program releases memory when it is no longer needed.
- Check for infinite loops: Make sure that the loops in your code have a way to exit, and that the conditions for exiting the loop are correct.
- Test the program: Test the program with a variety of inputs and use edge cases to identify the runtime errors.
It is important to note that debugging is an iterative process and it may take some time and effort to identify and correct runtime errors. However, with the right tools and techniques, it can be done effectively.
Best Practices for Debugging Common Coding Errors and Mistakes
Commenting and documentation are essential for debugging and maintaining code. Comments provide information about the purpose of the code and how it works, making it easier to understand and modify. Well-written comments can also help identify problems and suggest solutions. Additionally, having good documentation of the overall design and architecture of the codebase can make it easier to understand the code and identify issues.
For more information on the importance of commenting and how to write effective comments for improved code readability and maintainability, please check this post: The Importance of Commenting in Programming: How to Write Effective Comments for Improved Code Readability and Maintainability
Using debugging tools
Debugging tools are an essential part of the debugging process. Breakpoints allow you to pause the program’s execution at a specific line of code, so you can check the values of variables and expressions. Print statements (console logs) allow you to output the values of variables and expressions to the console, which can help you identify where a problem is occurring.
Collaboration and seeking help from others
This can be extremely valuable when debugging. Other people may be able to spot mistakes or offer suggestions that you may not have considered. Additionally, working with others can help you learn new debugging techniques and tools. Collaboration can also be helpful in understanding the overall design and architecture of the codebase. Pair programming is an excellent approach to this.
It’s important to keep in mind that debugging is an iterative process and it may take some time and effort to identify and correct errors. However, by utilizing appropriate tools, techniques, and best practices, it can be done efficiently. Additionally, debugging is not just about fixing errors, it’s also a great way to learn and improve your problem-solving skills.
Conclusion
In this post, we have discussed the importance of debugging, the types of coding errors and mistakes that can occur, and best practices for identifying and correcting them. We have covered syntax errors, logic errors, and runtime errors, as well as the importance of commenting and documentation, using debugging tools, collaboration, and seeking help from others.
Debugging is a crucial step in the software development process, and it is important to practice it regularly to improve your problem-solving skills. The more you debug, the more you’ll understand how to identify and correct errors, and the better you’ll get at it. Additionally, debugging can be a great way to learn new tools, techniques, and best practices.
There are many resources available to help you learn more about debugging, including online tutorials, books, and forums. Some popular resources include developer documentation for your programming language and debugging tools, online communities such as Stack Overflow, and blogs and tutorials from experienced developers. Additionally, there are many debugging tools available that can be very helpful in identifying and correcting errors.
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
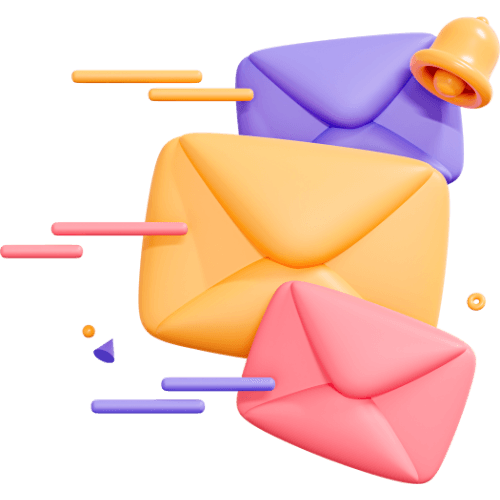
Related articles
5 Articles
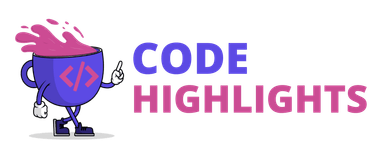
Copyright © Code Highlights 2025.