How to open a link in a new tab with HTML and JavaScript

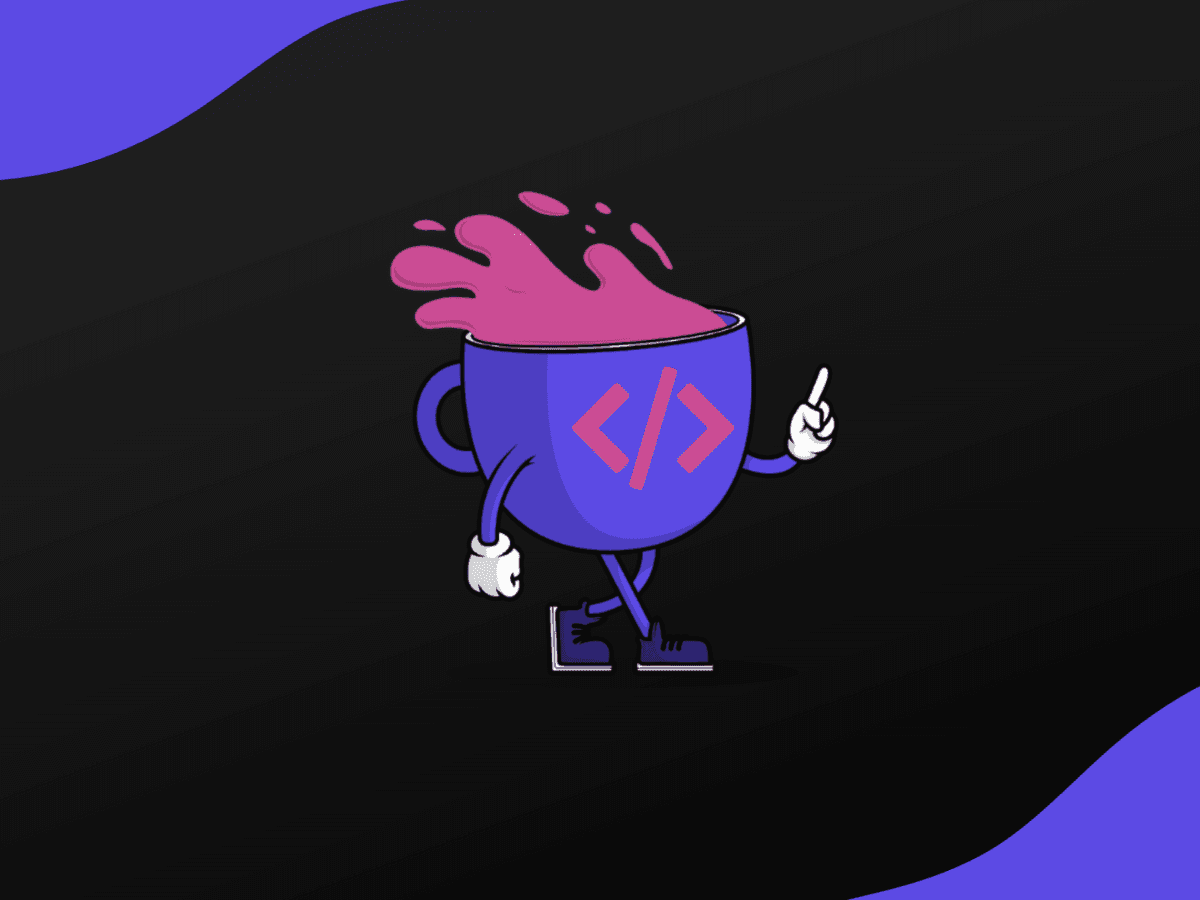
- HTML: target="_blank"
- JavaScript: window.open()
- JavaScript Event Listener
- Opening All Links in a New Tab
HTML and JavaScript provide several ways to open links in a new tab, enhancing user experience by keeping the original page open. This tutorial will guide you through these methods.
HTML: target="_blank"
In HTML, you can use the target="_blank"
attribute in your anchor tag (<a>
) to open the linked page in a new tab.
1<a href="https://www.example.com" target="_blank">Visit Example.com</a>
This method is straightforward and doesn't require any JavaScript. However, it's important to add rel="noopener noreferrer"
for security reasons.
JavaScript: window.open()
JavaScript provides the window.open()
method to open a link in a new tab. This method requires the URL as the first argument.
The second argument, '_blank'
, specifies that the URL should open in a new tab.
JavaScript Event Listener
You can also use JavaScript to add an event listener to a link. When the link is clicked, the event listener triggers the window.open()
method.
1var link = document.querySelector('#myLink');
2link.addEventListener('click', function(e) {
3 e.preventDefault();
4 window.open(this.href, '_blank');
5});
In this example, preventDefault()
stops the link from opening in the same tab, and this.href
gets the URL from the clicked link.
Opening All Links in a New Tab
To automatically open all links in a new tab, you can use a simple JavaScript loop:
1var links = document.querySelectorAll('a');
2for (var i = 0; i < links.length; i++) {
3 links[i].setAttribute('target', '_blank');
4}
This script finds all <a>
elements and sets their target
attribute to '_blank'
.
Remember, it's important to consider user experience when deciding to open links in new tabs. Not all users may appreciate this behavior. For more on HTML and JavaScript, check out our HTML fundamentals course and Learn JavaScript courses.
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
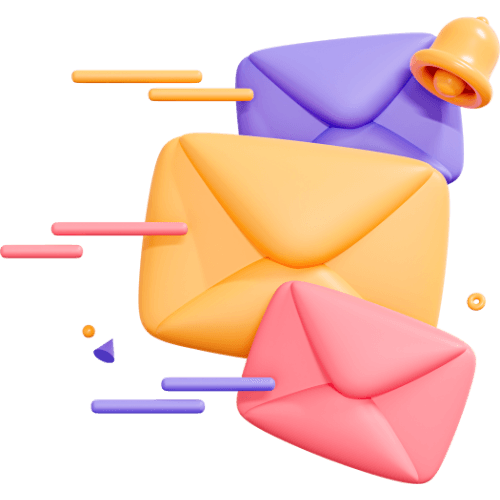
Related articles
9 Articles
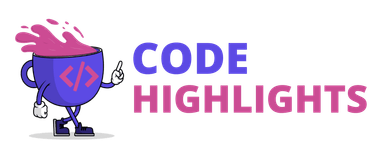
Copyright © Code Highlights 2024.