Blob to File JavaScript: Top 7 Mistakes Developers Make

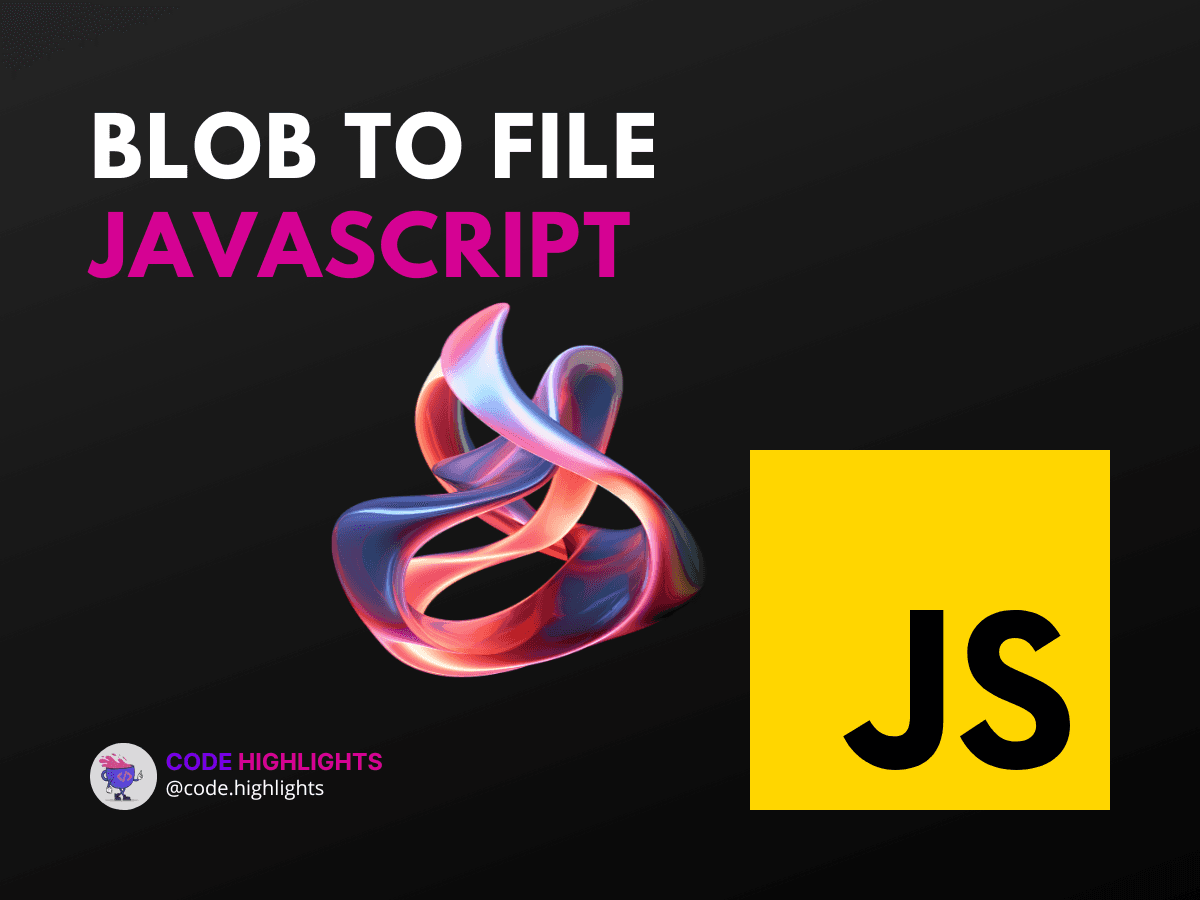
- How to Get File Blob in JavaScript?
- What is the Difference Between File and Blob in JavaScript?
- What is Blob() in JS?
- How to Convert Blob to PDF in Node.js?
- Top 7 Mistakes When Converting Blob to File in JavaScript
When diving into the world of web development, understanding how to handle different data types is crucial. In JavaScript, Blob
and File
objects are often sources of confusion and mistakes. This tutorial will illuminate the common pitfalls developers face when converting a blob to file JavaScript
, ensuring you can navigate these waters with confidence.
Let's start with a sneak peek at what we're dealing with. Here's a basic example of converting a Blob
to a File
in JavaScript:
1// Create a Blob object
2const blob = new Blob(["Hello, world!"], { type: 'text/plain' });
3
4// Convert Blob to File
5const file = new File([blob], "example.txt", {
6 type: blob.type,
7 lastModified: new Date().getTime()
8});
This snippet is just the beginning. Let's dive deeper into the nuances of blob to file JavaScript
and ensure you're equipped to avoid the top mistakes developers make.
How to Get File Blob in JavaScript?
A Blob
represents binary data in an immutable, raw format. It's often used for operations like file I/O (Input/Output). You can retrieve a Blob
from various sources, for instance, from an XMLHttpRequest
response, or by creating one directly using the Blob()
constructor.
Here's how you might get a Blob
from an input element:
1document.getElementById('input').addEventListener('change', function(e) {
2 const fileBlob = e.target.files[0];
3 // Now you have a Blob from the file input
4});
For more on JavaScript basics, check out this introductory course.
What is the Difference Between File and Blob in JavaScript?
While a Blob
is a generalized object representing raw binary data, a File
object is a specialized version that inherits from Blob
. A File
object contains metadata such as name
and lastModified
, making it akin to the file concept on your computer.
Understanding the difference is essential to prevent errors when manipulating these objects. For instance, while you can create a Blob
directly, a File
requires a bit more information to be instantiated correctly.
What is Blob() in JS?
In JavaScript, Blob()
is a constructor that allows you to create a new Blob
object containing raw data. You can specify the data and its MIME type, which is useful when you need to work with files in a binary format.
Here's an example:
This creates a Blob
containing the string "Hello World" with a MIME type of text/plain
.
How to Convert Blob to PDF in Node.js?
Converting a Blob
to a PDF in Node.js requires a buffer and a library like pdfkit
. Here's a simplified process:
1const PDFDocument = require('pdfkit');
2const fs = require('fs');
3const blobToBuffer = require('blob-to-buffer');
4
5// Assuming 'myBlob' is a Blob object you've obtained
6blobToBuffer(myBlob, (err, buffer) => {
7 if (err) throw err;
8
9 const doc = new PDFDocument();
10 doc.pipe(fs.createWriteStream('output.pdf'));
11 doc.image(buffer, 0, 15);
12 doc.end();
13});
Remember, Node.js doesn't have a native Blob
object, so you'll need to use a buffer equivalent. Explore more about Node.js and file handling in this web development course.
Top 7 Mistakes When Converting Blob to File in JavaScript
-
Ignoring Metadata: Not setting the
name
andlastModified
properties when creating aFile
can lead to issues when uploading or processing files server-side. -
Mishandling MIME Types: Incorrect MIME types can result in improper handling of the file content. Always set the correct type when creating a
Blob
orFile
. -
Overlooking Error Handling: Conversion processes can fail. Always include error handling to manage exceptions gracefully.
-
Neglecting Browser Compatibility: Some methods may not be supported across all browsers. Check compatibility before implementation.
-
Using Outdated Methods: Stay updated with the latest APIs and methods for working with
Blob
andFile
objects to avoid deprecated code. -
Confusing Blob and File: Remember that a
File
is aBlob
with extra properties. Treat them accordingly in your code. -
Inefficient Data Conversion: Avoid unnecessary conversions between
Blobs
andFiles
, as they can be performance-intensive.
By sidestepping these common mistakes, you'll be well on your way to mastering file handling in JavaScript. And for those interested in HTML fundamentals, consider this HTML course to strengthen your foundational knowledge.
In conclusion, dealing with blob to file JavaScript
can be tricky, but with a clear understanding and attention to detail, you can avoid these prevalent errors. Remember to keep your code clean, your error handling robust, and always test across different environments.
For further reading and best practices, check out these resources:
Happy coding, and may your blob to file
conversions be error-free!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
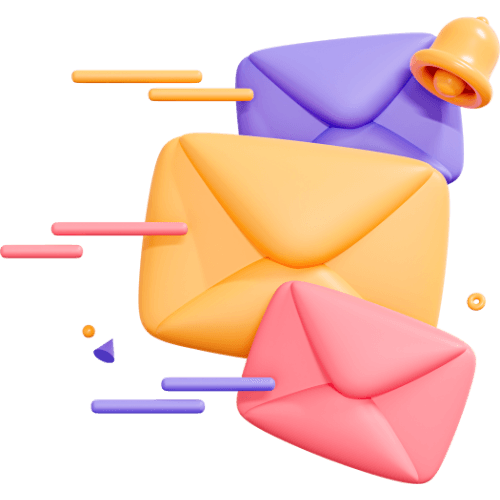
Related articles
9 Articles
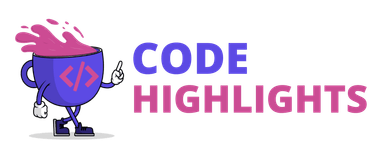
Copyright © Code Highlights 2025.