How to play a sound with JavaScript

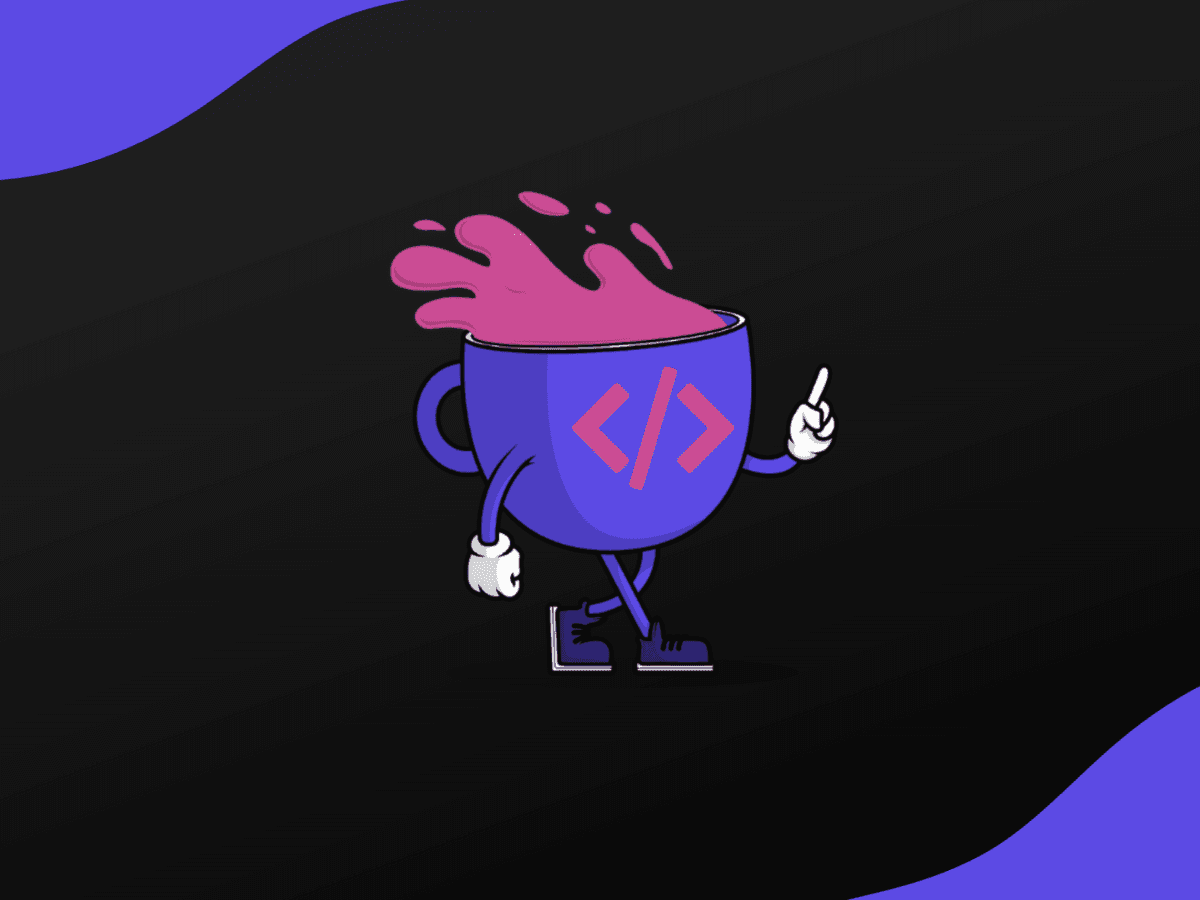
- Creating an Audio Element
- Playing a Sound with JavaScript
- Playing a Sound on Key Press
- Conclusion
In JavaScript, playing a sound can be accomplished using the HTML5 <audio>
element. This tutorial will guide you through the process of playing a sound in JavaScript and even playing a sound when a key is pressed.
Creating an Audio Element
First, we need to create an audio element. You can do this directly in your HTML or dynamically using JavaScript.
1<audio id="myAudio" src="sound.mp3"></audio>
Or
Playing a Sound with JavaScript
Once you've created an audio element, you can use JavaScript to play the sound. Here's how:
Or if you created the audio element with JavaScript:
Playing a Sound on Key Press
To play a sound when a key is pressed, we can add an event listener for the keydown
event.
1window.addEventListener("keydown", function (event) {
2 var audio = document.getElementById("myAudio");
3 audio.play();
4});
This will play the sound every time a key is pressed. If you want to play a sound when a specific key is pressed, you can check the keyCode
property of the event object.
1window.addEventListener("keydown", function (event) {
2 if (event.keyCode == 65) {
3 // 'A' key
4 var audio = document.getElementById("myAudio");
5 audio.play();
6 }
7});
This code will play the sound when the 'A' key is pressed. You can replace 65
with the keyCode of any other key you want. Here's a useful list of keyCodes.
Learn more about handling events in JavaScript
Conclusion
Playing a sound in JavaScript is as simple as creating an audio element and calling the play()
method. You can also easily play a sound when a key is pressed by adding an event listener for the keydown
event.
Start your journey with web development
Remember to replace 'sound.mp3'
with the actual path to the sound file you want to play. Also, ensure that your webpage has the necessary permissions to play audio.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
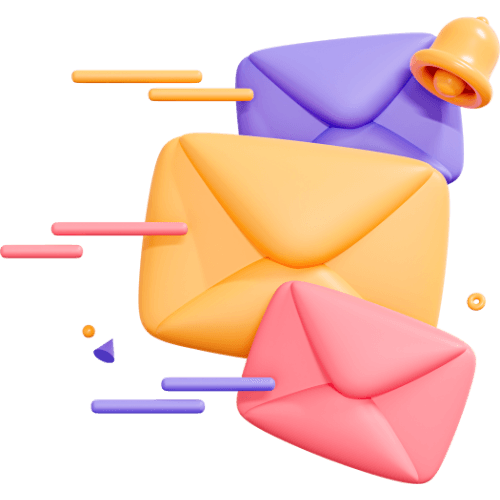
Related articles
9 Articles
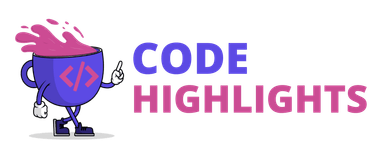
Copyright © Code Highlights 2024.