How to Manipulate 2D Array in JavaScript Effortlessly

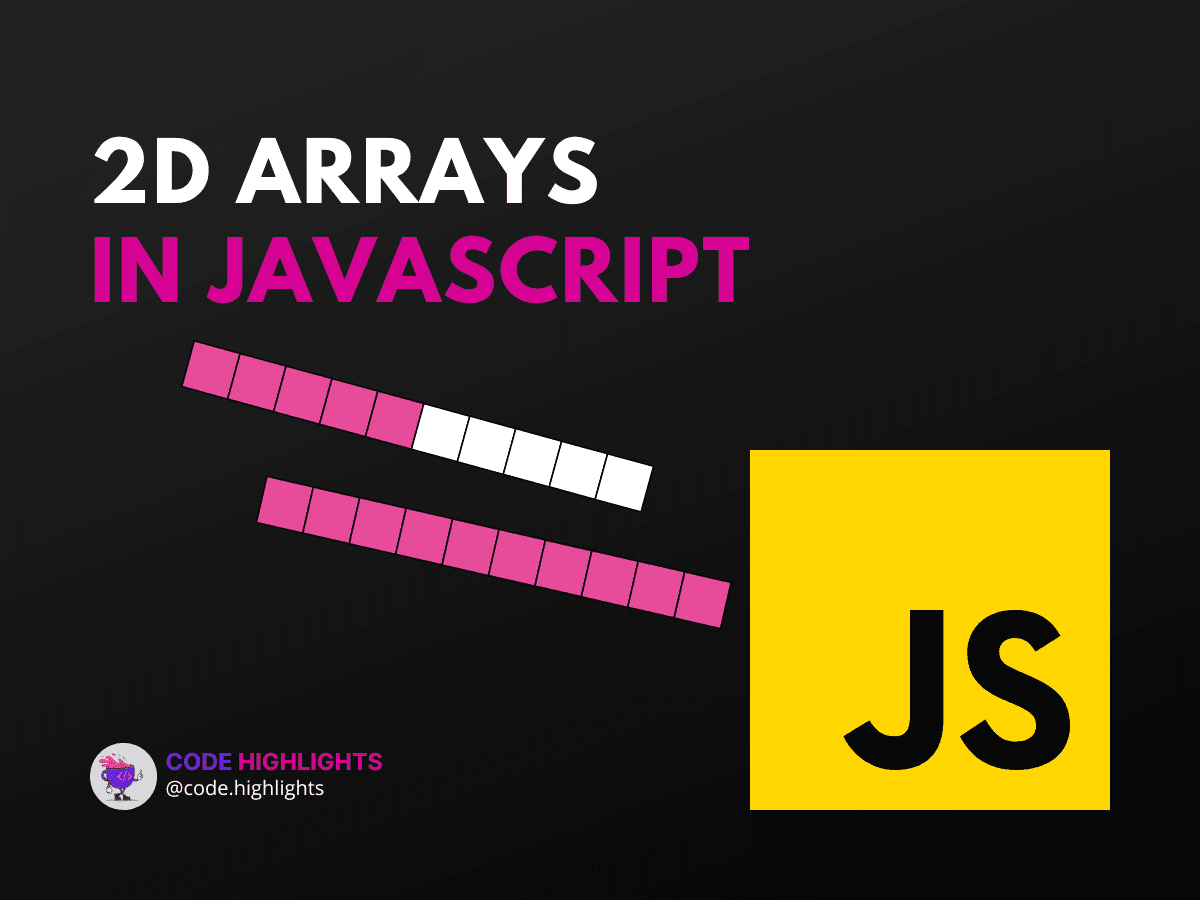
- What is a 2D Array in JavaScript?
- How to Make a 2D Array?
- How to Manipulate 2D Array in JS?
- What is a 2D Array Example?
- Conclusion
Are you looking to master the art of handling a 2d array in JavaScript
? You've come to the right place! A 2D array, also known as a matrix or a table, is an array of arrays, where each inner array represents a row of data. They are incredibly useful for storing and organizing data in a structured format, such as spreadsheet information or board game layouts.
Let's kick things off with a simple code snippet that illustrates a basic 2D array:
In this tutorial, we'll dive into how to create, access, and manipulate 2D arrays in JavaScript, ensuring you're equipped with the skills to manage them effortlessly. Ready to begin? Let's code our way through!
What is a 2D Array in JavaScript?
A 2D array in JavaScript
is essentially an array whose elements are also arrays. This structure allows us to store data in rows and columns, making it much easier to visualize and work with complex datasets. For instance, if you're interested in web development, understanding 2D arrays is crucial when dealing with tabular data (Introduction to Web Development).
How to Make a 2D Array?
Creating a 2D array in JavaScript is straightforward. You simply define an array and then fill it with more arrays, like so:
1let twoDArray = new Array(3); // Create an array with 3 slots
2for (let i = 0; i < twoDArray.length; i++) {
3 twoDArray[i] = new Array(3).fill(0); // Each slot is an array of 3 zeros
4}
This creates a 3x3 matrix filled with zeros. However, there are more ways to create 2D arrays, and understanding the basics of JavaScript (Learn JavaScript) will give you the foundation you need to explore these methods.
How to Manipulate 2D Array in JS?
Manipulating a 2D array can involve several operations, such as adding or removing rows and columns, updating values, or even rotating the matrix. Here’s how you can update a value in our earlier example:
To add a new row, you could use the push
method:
For more complex manipulations, it might be helpful to understand the fundamentals of HTML and CSS as well (HTML Fundamentals Course, Learn CSS Introduction).
What is a 2D Array Example?
Let's look at an example where we create a tic-tac-toe board using a 2D array in JavaScript:
This neatly represents the game board, where each row corresponds to a row in the game, and each element within those rows represents a cell on the board.
Conclusion
Mastering 2D arrays in JavaScript is a powerful skill that will serve you well in many programming tasks. Remember, practice is key—try creating different types of 2D arrays and manipulating them in various ways to solidify your understanding.
For further reading, check out these reputable sources that delve deeper into JavaScript arrays:
- Mozilla Developer Network's guide on Arrays
- W3Schools JavaScript Array Tutorial
- JavaScript.info's article on Arrays
With the knowledge you've gained today, you're now better equipped to tackle any challenges involving 2d arrays in JavaScript
. Keep experimenting and happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
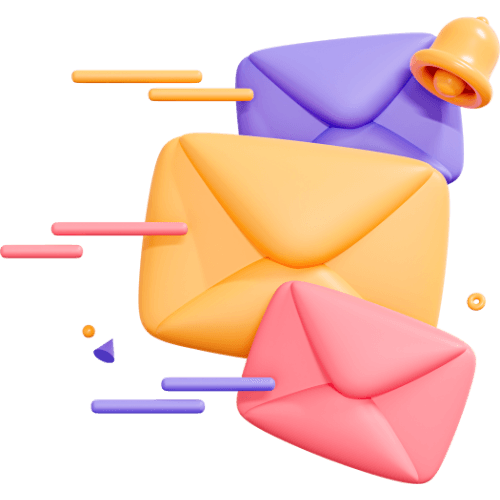
Related articles
9 Articles
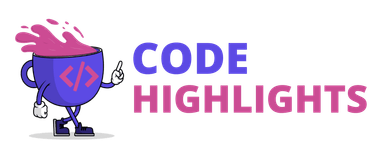
Copyright © Code Highlights 2025.