JavaScript Data Types: A Comprehensive List

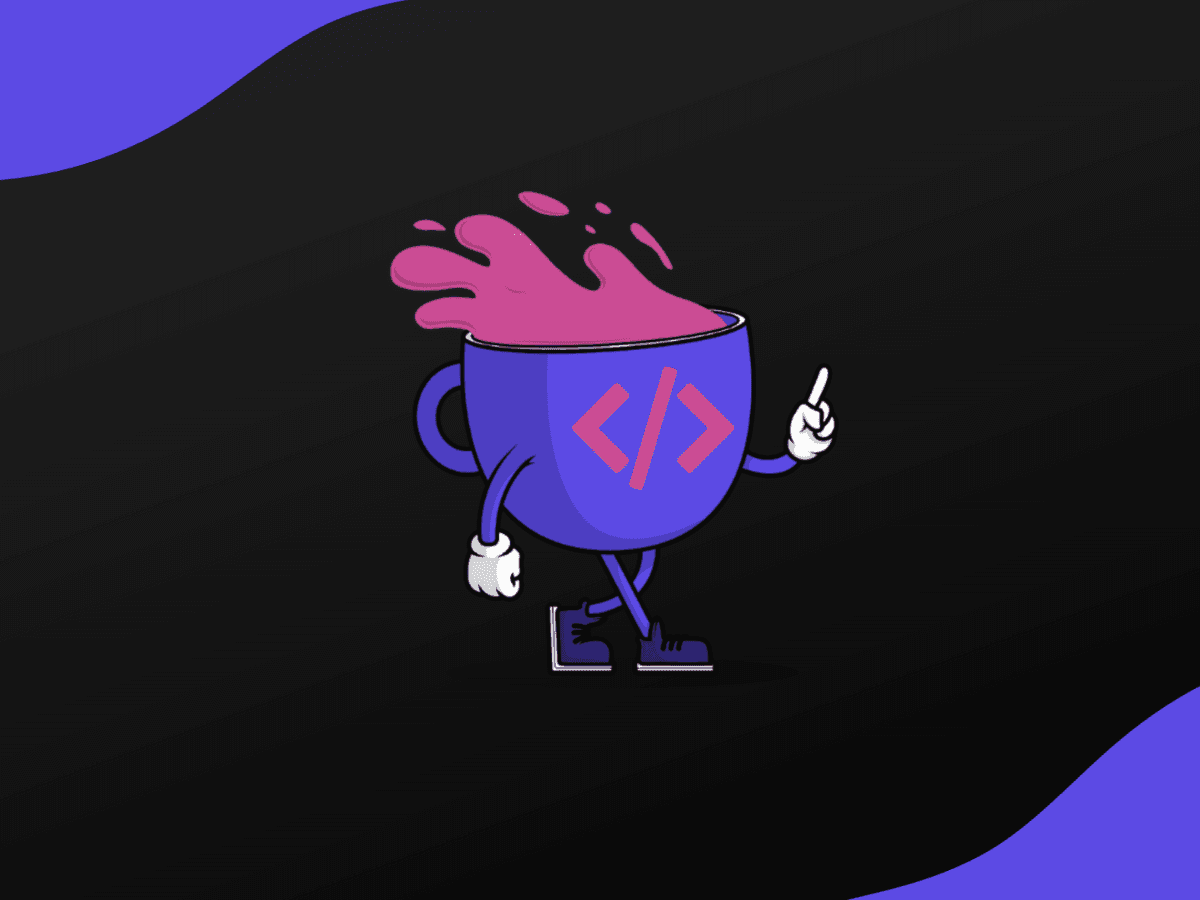
- Primitive Data Types
- 1. Numbers
- 2. Strings
- 3. Booleans
- 4. Null
- 5. Undefined
- 6. Symbols
- Object Data Types
- Conclusion
JavaScript is a dynamically typed language, meaning that data types are determined at runtime based on the assigned values. This can lead to some confusion, especially for beginners. In this article, we will cover the different data types in JavaScript and their properties.
Primitive Data Types
JavaScript has six primitive data types, which are immutable (cannot be changed). They are:
1. Numbers
Numbers represent both integer and floating-point values. They are denoted using the number
keyword.
Properties:
MAX_VALUE
: The maximum representable number in JavaScriptMIN_VALUE
: The smallest positive representable number in JavaScriptNEGATIVE_INFINITY
: Represents negative infinityPOSITIVE_INFINITY
: Represents positive infinityNaN
: Not a number
2. Strings
Strings represent text and are denoted using the string
keyword. They can be enclosed in single or double quotes.
Properties:
length
: Returns the length of the string
3. Booleans
Booleans represent the truth values true
and false
and are denoted using the boolean
keyword.
4. Null
Null represents the intentional absence of any object value and is denoted using the null
keyword.
5. Undefined
Undefined represents the absence of any value and is denoted using the undefined
keyword. It is typically used for uninitialized variables or missing object properties.
6. Symbols
Symbols represent unique identifiers and are denoted using the symbol
keyword. They are typically used as property keys in objects.
Object Data Types
The seventh data type in JavaScript is objects, which are mutable and can contain any combination of primitive and object values. They are denoted using curly braces {}
and their properties are specified using key-value pairs.
Properties:
Object.keys()
: Returns an array of object keysObject.values()
: Returns an array of object valuesObject.entries()
: Returns an array of key-value pairs
Conclusion
Knowing the different data types in JavaScript is essential for writing high-quality code. Understanding their properties and usage will help you avoid common programming errors and improve your productivity.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
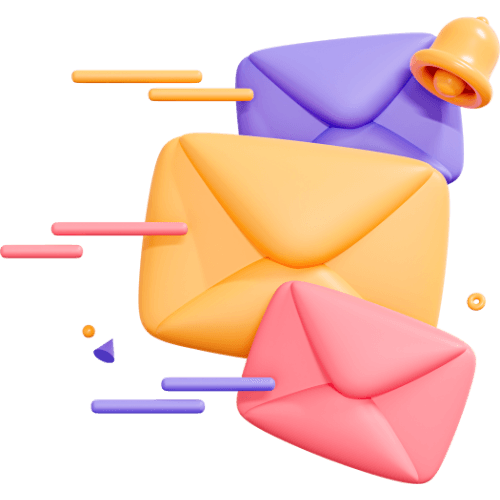
Related articles
9 Articles
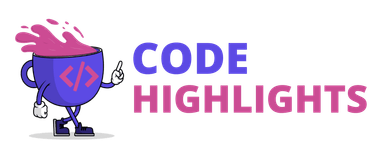
Copyright © Code Highlights 2025.