Debugging JavaScript Code

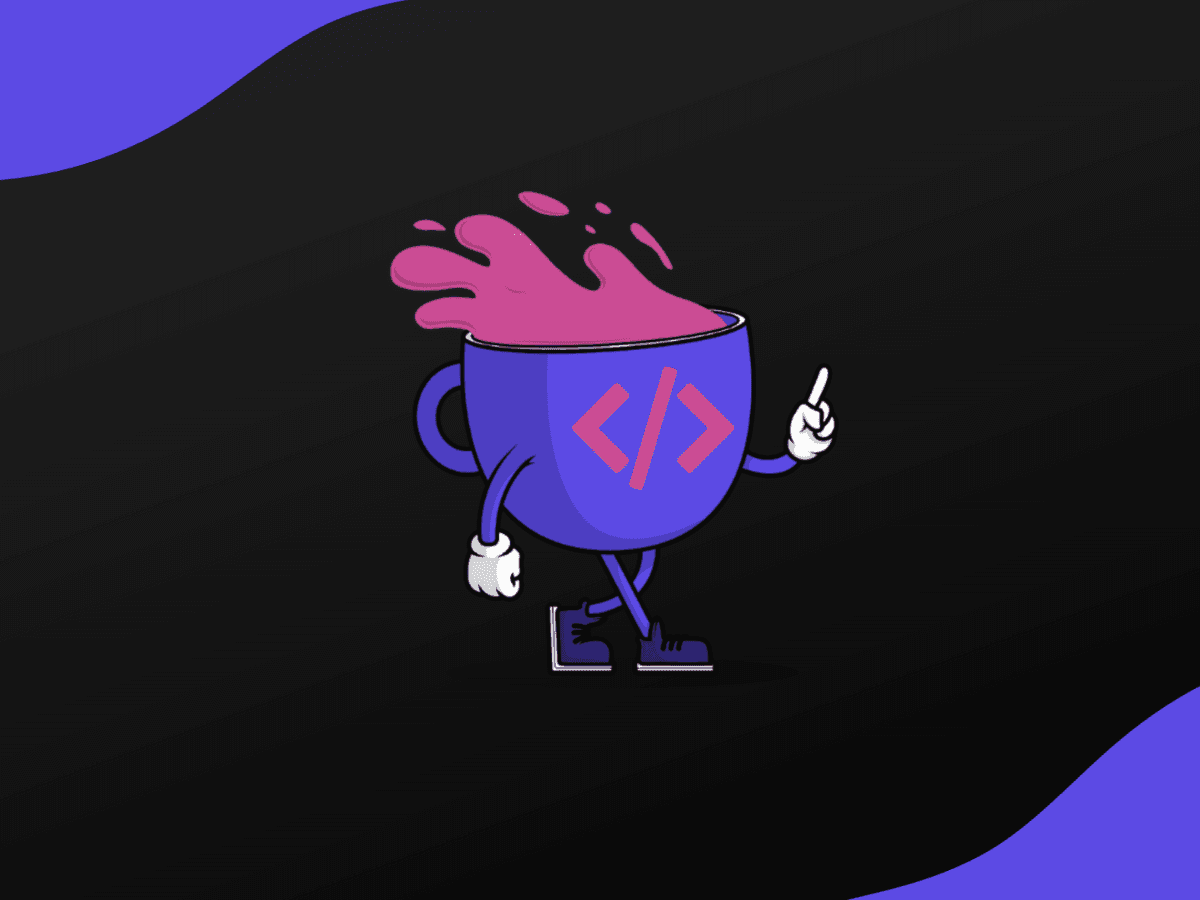
- Console Logging
- Conditional Breakpoints
- Event Listener Breakpoints
- Network Tab
- Conclusion
Debugging is an important aspect of software development. It helps developers identify and fix errors in their code to ensure that the program runs smoothly. Debugging JavaScript code can be a challenging task, especially for complex applications. In this article, we'll explore some advanced techniques for debugging JavaScript code.
Console Logging
The console.log()
method is a well-known debugging tool for JavaScript developers. It allows you to log specific values and variables in the console, providing valuable insight into the flow of your application. However, sometimes you may want to log more than just variables or values. You can log complex objects, functions, and even CSS styles using the console.log()
method in conjunction with the %c
symbol.
The %c
symbol is a special character in the console.log()
method that allows you to add CSS styles to the output. Here's an example:
The first argument is the message you want to log, while the second argument is a string of CSS styles that will be applied to the message.
Conditional Breakpoints
Conditional breakpoints are a useful feature that can be used to stop the execution of your code at specific points. This allows you to inspect the current state of your application and fix any issues. Conditional breakpoints can be set up in most modern browsers' debugging tools.
To set a conditional breakpoint, right-click on the line number where you want to stop the execution of your code and select "Add conditional breakpoint". Then, enter the condition you want to trigger the breakpoint. Here's an example:
This code will stop the execution of the JavaScript code when the value of the variable i
is equal to 5.
Event Listener Breakpoints
Event listener breakpoints are a special type of breakpoint that triggers when an event listener is fired. This is useful for debugging events such as clicks, keypresses, and form submissions. Event listener breakpoints can also be set up in most modern browsers' debugging tools.
To set an event listener breakpoint, navigate to the "Event Listener Breakpoints" tab in the debugging tool and select the type of event you want to trigger the breakpoint. Here's an example:
This code will log the details of the click event to the console each time a user clicks on the page.
Network Tab
The network tab is a powerful tool for debugging network-related issues. It shows you all the network requests that have been made by your application, including their status codes, headers, and timings. By analyzing the network tab, you can identify issues such as slow response times and failed requests.
To access the network tab, navigate to the "Network" tab in the debugging tool and start interacting with your application. All network requests made by your application will be displayed in the tab.
Conclusion
Debugging JavaScript code requires a lot of patience and perseverance. However, by using the techniques outlined in this article, you can gain valuable insight into the inner workings of your application and fix any issues. Remember to use console logging, conditional breakpoints, event listener breakpoints, and the network tab to help you debug your JavaScript code effectively.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
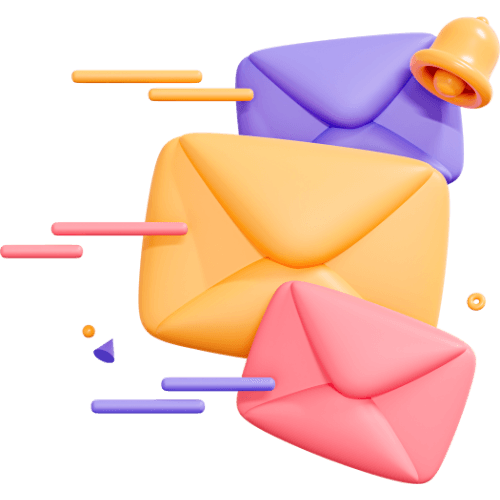
Related articles
9 Articles
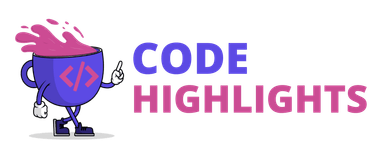
Copyright © Code Highlights 2025.