Mastering Javascript Remove Hash from URL in Seconds

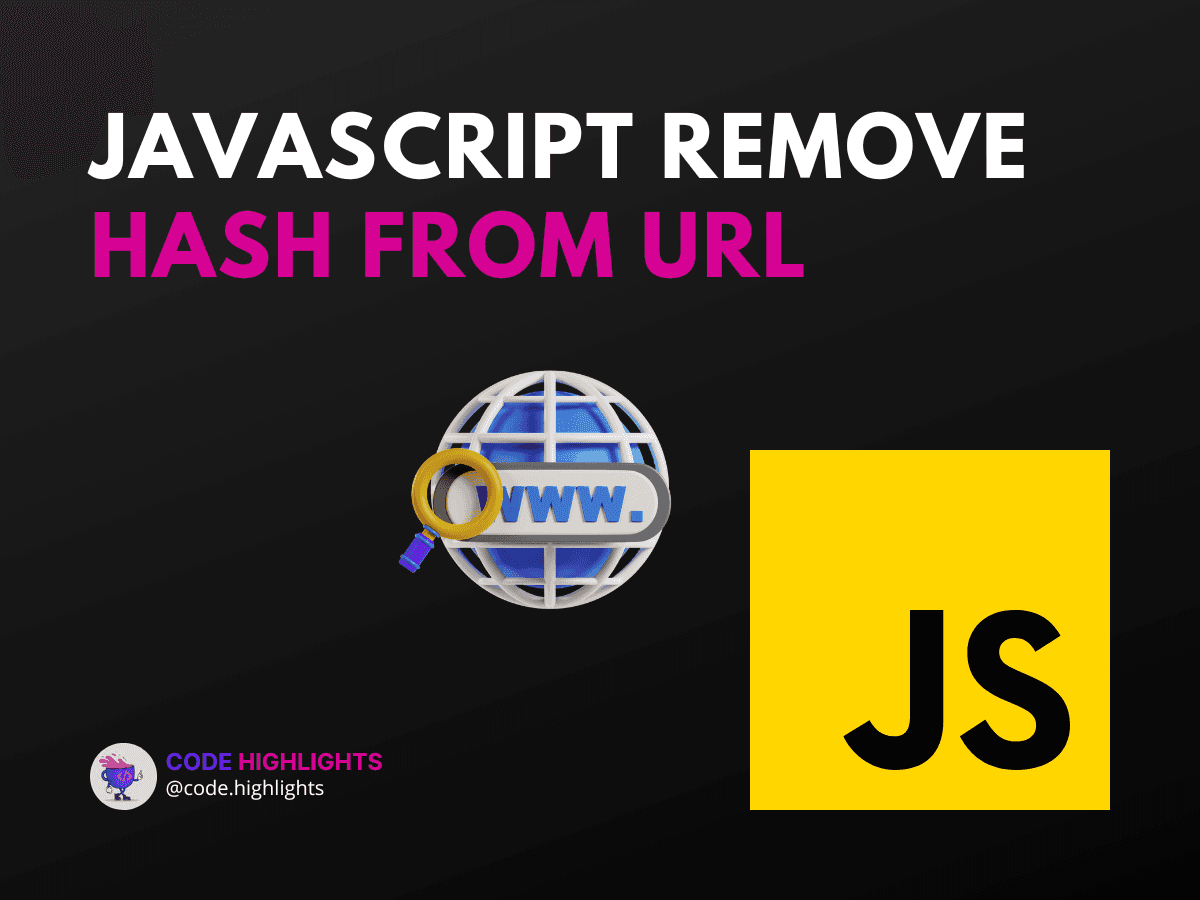
- Understanding the URL Hash
- Step-by-Step Guide to Remove the Hash
- Best Practices and Tips
- External Resources for Further Learning
Welcome to a practical guide where we'll demystify a common task in web development—removing the hash from a URL using JavaScript. Whether you're refining navigation, enhancing user experience, or just tidying up URLs for sharing, mastering this technique is a valuable skill. Before we dive into the code, let's briefly understand what a URL hash is. A hash, or fragment identifier, is often used for navigating to specific sections within a page. However, there are times when you want to remove it—say, after the user has navigated to the desired section, and you want to clean up the address bar.
Here's a sneak peek at how simple it can be:
This line of code is like a magic wand that makes the hash disappear without reloading the page. Excited to learn more? Let's get started!
Understanding the URL Hash
A URL hash looks like this: http://example.com/page.html#section1
. The #section1
part is the hash. It's client-side, meaning it doesn't affect the server—it's purely for the browser's consumption. If you're curious about the basics of web development, consider exploring Introduction to Web Development.
Step-by-Step Guide to Remove the Hash
- Access the Current URL: First, grab the current URL using
window.location.href
. - Identify the Hash: The hash is everything after the
#
symbol. JavaScript provideswindow.location.hash
for easy access. - Modify the URL: To remove the hash, we use the
history
API'spushState
method.
Here's the code in action:
1// Step 1: Access the current URL
2var currentURL = window.location.href;
3
4// Step 2: Check if there is a hash
5if (window.location.hash) {
6 // Step 3: Remove the hash
7 window.history.pushState("", document.title, currentURL.split('#')[0]);
8}
After executing this script, the URL will no longer display the hash. But what if you're working with dynamic content? Learn more about handling such scenarios with Learn JavaScript.
Best Practices and Tips
- Browser Support: Ensure compatibility across browsers. The
history
API is widely supported, but older browsers may require a fallback. - SEO Implications: Changing URLs client-side can affect SEO. Use this method judiciously, keeping in mind the HTML fundamentals course.
- User Experience: Consider the user journey. Don't disrupt their experience by removing hashes they might rely on for navigation.
External Resources for Further Learning
Removing the hash from a URL using JavaScript is straightforward once you understand the underlying concepts. With this guide, you're now equipped to handle this task with confidence. For styling your pages once the hash is gone, check out Learn CSS: Introduction. Keep experimenting, keep learning, and most importantly, keep coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
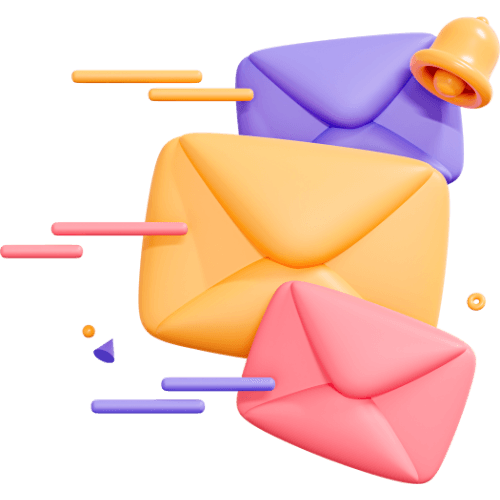
Related articles
9 Articles
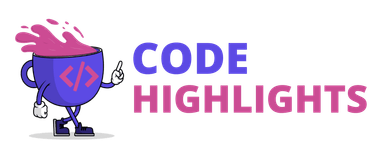
Copyright © Code Highlights 2025.