7 Proven Strategies to Master Call in JavaScript

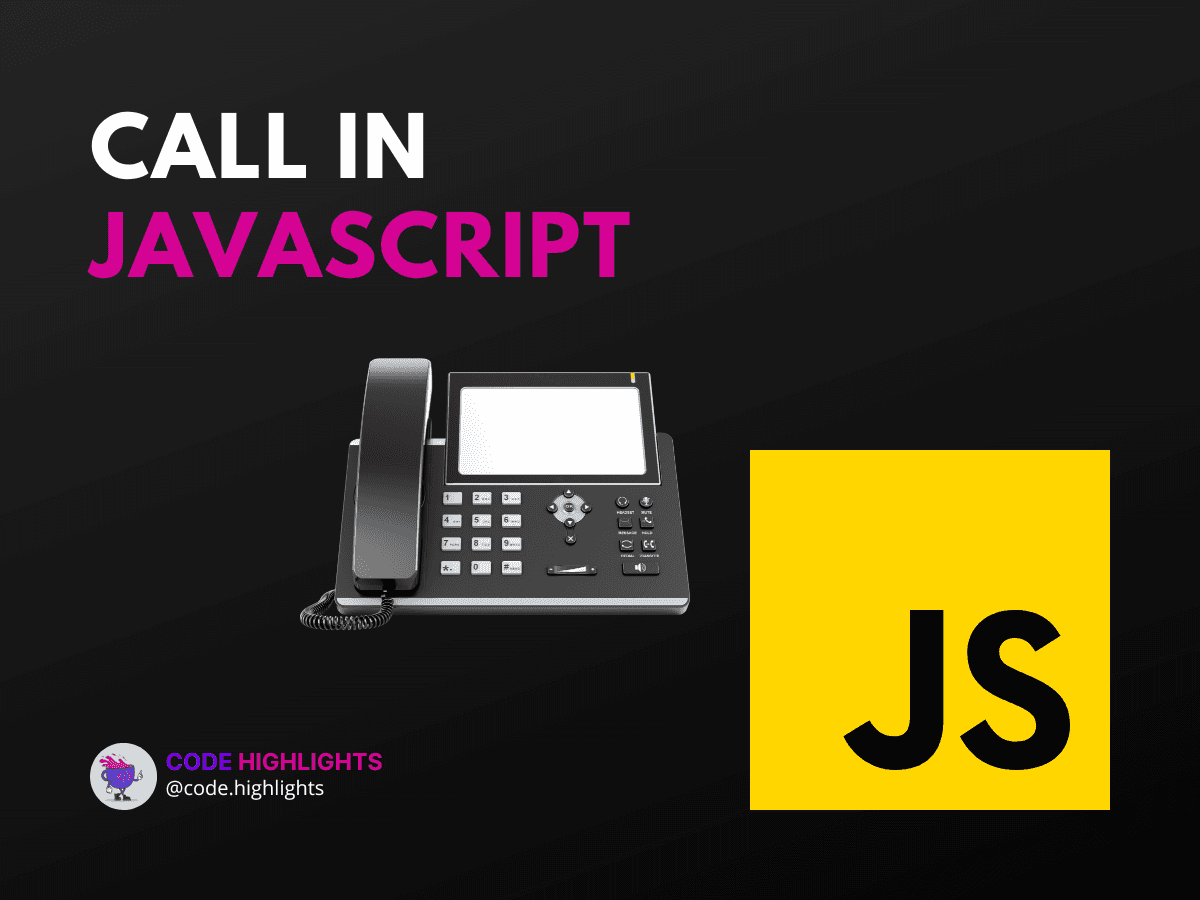
- Understanding the Basics
JavaScript is a language teeming with powerful features and nuances, one of which is the call
method. This intriguing function invocation capability can be a game-changer for your coding projects. Let's dive into the world of call
in JavaScript, where we'll unlock its potential through practical strategies.
First, here's a sneak peek at the syntax of call
:
1function display() {
2 console.log(this.message);
3}
4
5var obj = {
6 message: 'Hello, World!'
7};
8
9// Using call to invoke the function
10display.call(obj); // Outputs: Hello, World!
In this tutorial, we'll explore seven strategies to master call
in JavaScript, ensuring you have a robust understanding and can apply it confidently in your scripts.
Understanding the Basics
Before we delve into the strategies, let's ensure we're on the same page. The call
method allows you to invoke a function with an explicitly set this
value. You can also pass arguments to the function as if you were calling it directly.
Strategy 1: Binding Contexts Dynamically
When working with object-oriented JavaScript, controlling the context (this
) is crucial. Use call
to invoke methods in different contexts dynamically:
1function greet() {
2 console.log(`Hello, I'm ${this.name}`);
3}
4
5const person1 = { name: 'Alice' };
6const person2 = { name: 'Bob' };
7
8greet.call(person1); // Hello, I'm Alice
9greet.call(person2); // Hello, I'm Bob
Strategy 2: Borrowing Methods
One of the most powerful aspects of call
is method borrowing. This allows you to use methods from other objects without inheritance:
1let fruits = ['apple', 'banana'];
2
3// Borrowing the slice method from Array prototype
4let slicedFruits = Array.prototype.slice.call(fruits, 0, 1);
5
6console.log(slicedFruits); // Outputs: ['apple']
Strategy 3: Invoking Functions Immediately
Functions can be invoked immediately using call
, which is especially useful when dealing with the Function
constructor or when you need to execute a function right away with a specific context.
Strategy 4: Using call
in Event Handlers
When dealing with DOM events, you might want to invoke a function with a specific context using call
.
Strategy 5: Currying Functions
Currying is a technique in which a function doesn't take all of its arguments up front. Instead, you create a chain of functions that each take a subset of the arguments:
1function multiply(a, b) {
2 return a * b;
3}
4
5let double = multiply.bind(null, 2);
6double = double.call(null, 5); // Outputs: 10
Strategy 6: Testing and Debugging
Use call
to test functions with different contexts in your debugging process. This can help you understand how your functions behave under different conditions.
Strategy 7: Optimizing Performance
While call
can be slightly faster than apply
in some JavaScript engines due to the way arguments are handled, it's crucial to profile your code before making performance-based decisions.
Throughout this tutorial, you'll learn to wield these strategies with precision. To further expand your JavaScript knowledge, consider enrolling in this comprehensive JavaScript course.
If you're new to web development, it's also beneficial to have a solid understanding of HTML and CSS. Check out this HTML fundamentals course and this introduction to learning CSS. Together, they lay the foundation for powerful web development skills.
For a broader overview, this introduction to web development course can provide you with a holistic understanding of building websites.
As you apply these strategies, remember to consult documentation and resources from reputable sources like Mozilla Developer Network (MDN) or W3Schools to deepen your understanding of call
and other JavaScript methods.
By mastering call
in JavaScript, you'll enhance your ability to write clean, efficient, and maintainable code. Embrace these strategies, practice diligently, and watch as you transform into a more proficient JavaScript developer. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
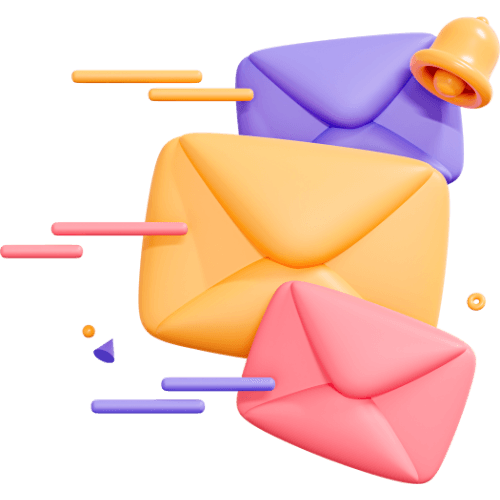
Related articles
9 Articles
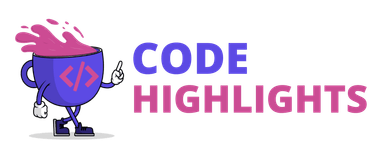
Copyright © Code Highlights 2024.