5 Proven Techniques for Mastering Chaining in JavaScript

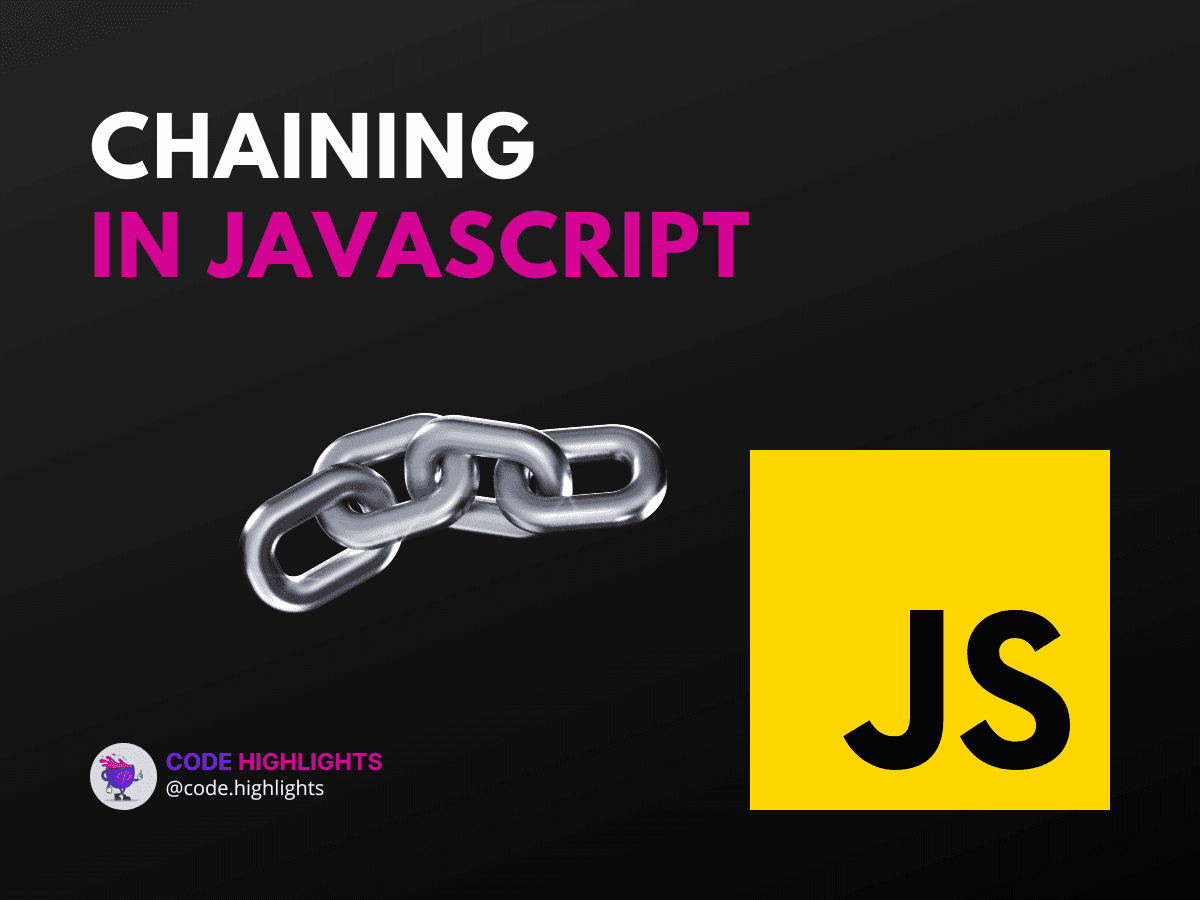
- What is chaining in programming?
- How to Chain Two Functions in JavaScript
- What is JavaScript Optional Chaining?
- What is chaining in Node.js?
- Technique 1: Method Chaining
- Technique 2: Prototype Chaining
- Technique 3: Promise Chaining
- Technique 4: Function Composition
- Technique 5: Async/Await Chaining
Are you looking to streamline your JavaScript code and make it more readable? Chaining in JavaScript is a powerful technique that can help you do just that. By the end of this tutorial, you'll be able to chain methods like a pro, creating sleek and efficient code that's easy to understand.
Let's start with a simple example of chaining:
In this line, we're filtering an array for numbers greater than 10 and then mapping those numbers to double their value, all in one smooth operation.
What is chaining in programming?
Chaining, in the context of programming, refers to the process of connecting multiple methods or functions together in a single line of code. Each method in the chain acts on the result of the preceding one without the need for intermediary variables. This pattern is not only elegant but also reduces the verbosity of the code.
How to Chain Two Functions in JavaScript
Chaining two functions in JavaScript is straightforward. Consider the following scenario:
1function double(x) {
2 return x * 2;
3}
4
5function addFive(x) {
6 return x + 5;
7}
8
9let result = addFive(double(10)); // Outputs 25
Here, double(10)
is evaluated first, returning 20
, which is then passed to addFive
, resulting in 25
. It's a simple yet effective way to combine operations.
What is JavaScript Optional Chaining?
Optional chaining (introduced in ES2020) is a game-changer in JavaScript. It allows you to safely access deeply nested object properties without an explicit check for null or undefined values. The syntax looks like this:
If obj
or prop
is undefined or null, the expression short-circuits and returns undefined instead of throwing an error.
What is chaining in Node.js?
In Node.js, chaining is often used in the context of streams, allowing you to pipe output from one stream directly into another. For instance:
1fs.createReadStream('input.txt')
2 .pipe(zlib.createGzip())
3 .pipe(fs.createWriteStream('output.txt.gz'));
This snippet chains file reading, gzipping, and writing operations seamlessly.
Technique 1: Method Chaining
Method chaining is commonly used in libraries like jQuery and Lodash, which return the original object (or a modified copy) from their methods, enabling multiple actions to be performed in a single line. Here's an example using jQuery:
Each method operates on $('#myElement')
, altering it or its display without breaking the chain.
Technique 2: Prototype Chaining
By extending prototypes, you can implement your own method chaining in custom objects. Just ensure each method returns this
:
1class Calculator {
2 constructor(value = 0) {
3 this.value = value;
4 }
5
6 add(n) {
7 this.value += n;
8 return this;
9 }
10
11 multiply(n) {
12 this.value *= n;
13 return this;
14 }
15}
16
17let calc = new Calculator(10).add(5).multiply(2);
18console.log(calc.value); // Outputs 30
Technique 3: Promise Chaining
Promise chaining is crucial for handling asynchronous operations in sequence:
1fetch('/data.json')
2 .then(response => response.json())
3 .then(data => console.log(data))
4 .catch(error => console.error(error));
Each .then()
waits for the previous Promise to resolve before executing.
Technique 4: Function Composition
Function composition is a functional programming concept where you combine multiple functions to create a new one:
1const compose = (f, g) => x => f(g(x));
2const addSixAndDouble = compose(double, addFive);
3console.log(addSixAndDouble(10)); // Outputs 30
Technique 5: Async/Await Chaining
With async/await, you can write asynchronous code that looks synchronous:
1async function fetchData() {
2 try {
3 let response = await fetch('/data.json');
4 let data = await response.json();
5 console.log(data);
6 } catch (error) {
7 console.error(error);
8 }
9}
Each await
pauses execution until the Promise resolves, allowing for a clear sequence of operations.
In conclusion, mastering chaining in JavaScript can significantly improve the way you write and understand code. Whether you're working with arrays, promises, or your own custom objects, these five techniques will equip you with the skills to write concise and maintainable code.
For further learning, consider checking out our JavaScript course, diving into our HTML fundamentals course, exploring the world of CSS, or starting from scratch with our introduction to web development.
Now that you're familiar with chaining, why not take your JavaScript knowledge to the next level? Remember, practice makes perfect, so start implementing these techniques in your next project!
Note: The examples provided in this tutorial are simplified to demonstrate the concepts clearly. Real-world scenarios may require additional error handling and considerations.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
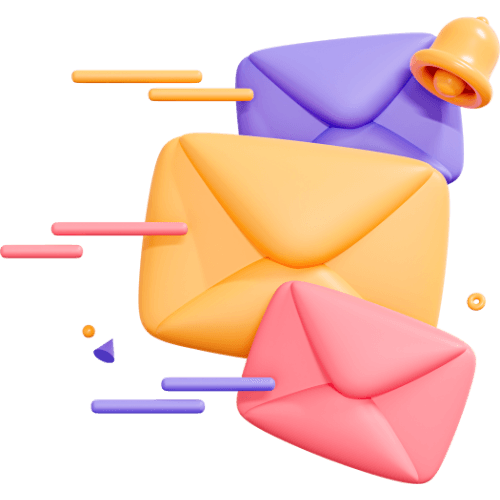
Related articles
9 Articles
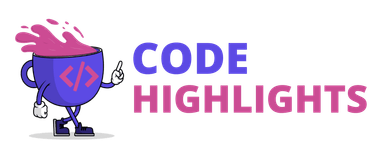
Copyright © Code Highlights 2025.