Change Element ID JavaScript: Boost Your DOM Manipulation Skills

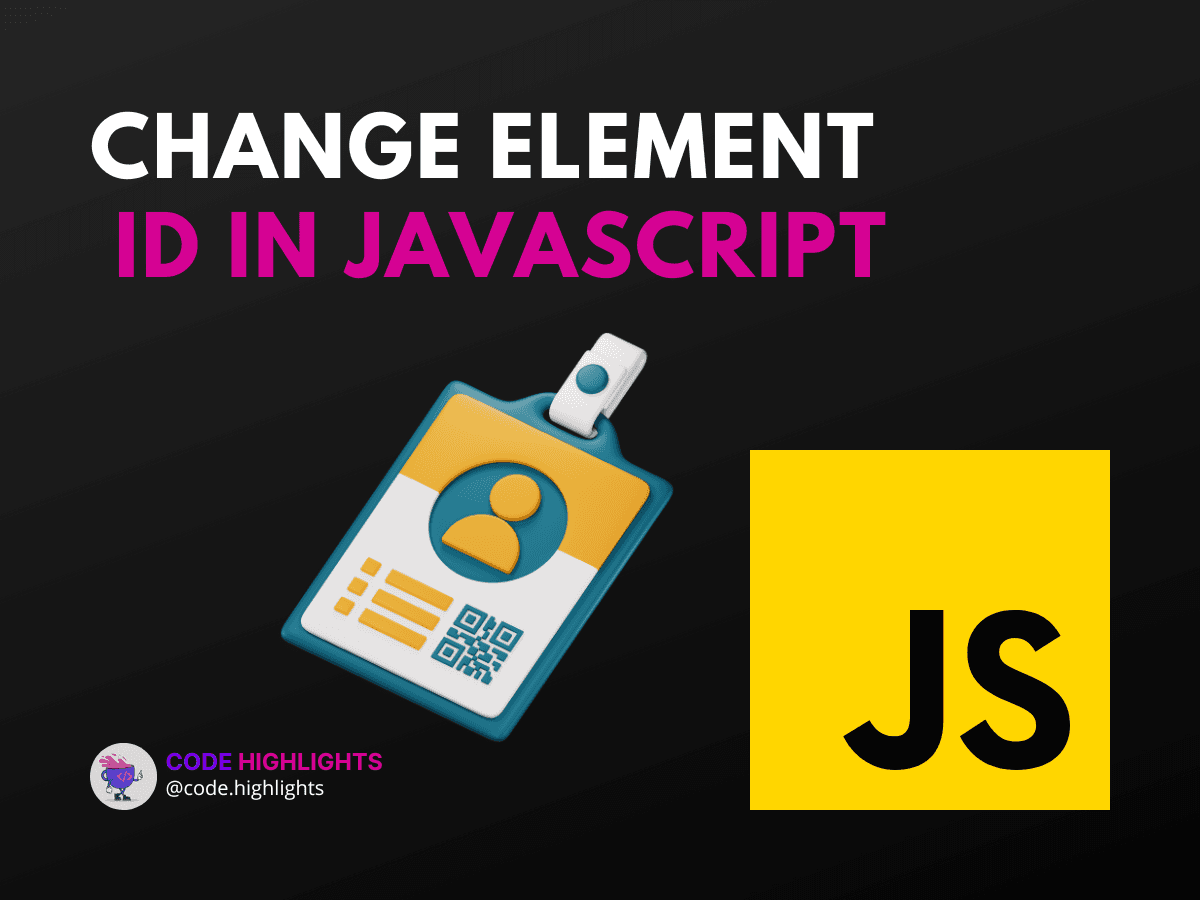
- Understanding the Basics
- Step-by-Step Guide to Change an Element's ID
- Practical Example
- Best Practices and Considerations
- Conclusion
Welcome to a practical journey into the world of JavaScript and DOM manipulation! If you've ever found yourself needing to change the ID of an HTML element on the fly, you're in the right place. This tutorial will walk you through the steps to dynamically alter element IDs using JavaScript, a skill that can greatly enhance the interactivity and responsiveness of your web pages.
In the example above, we've used JavaScript to target an element with the ID of oldId
and changed it to newId
. It might look simple, but understanding this process opens up a world of possibilities for customizing user experiences on the web.
Understanding the Basics
Before diving into the code, let's ensure we have a solid foundation. If you're new to JavaScript or need a refresher, check out this JavaScript course. For those of you who want to brush up on HTML or CSS, we've got great resources for you as well, with our HTML fundamentals course and CSS introduction.
Changing an element's ID is a common task when working with the Document Object Model (DOM), which represents the page so that programs can change the document structure, style, and content. The DOM represents the document as nodes and objects; that way, programming languages can interact with the page.
A web page is documented in HTML, and each element within the HTML is part of the DOM. To learn more about how all these technologies come together, consider taking a course on web development.
Step-by-Step Guide to Change an Element's ID
-
Identify the Element: First, you need to get a reference to the element whose ID you want to change. This is commonly done using
document.getElementById
or other selectors likedocument.querySelector
. -
Change the ID: Once you have the element, changing the ID is as simple as setting the
id
property to a new value. -
Verify the Change: It's always a good idea to ensure that your change was successful.
Practical Example
Imagine you have a list of items, and you want to highlight one by changing its ID to highlighted-item
when it's clicked. Here's how you could do it:
1document.addEventListener("DOMContentLoaded", function() {
2 var items = document.querySelectorAll(".item");
3 items.forEach(function(item) {
4 item.addEventListener("click", function() {
5 this.id = "highlighted-item";
6 });
7 });
8});
In the code above, we add an event listener to each item that listens for a click event. When an item is clicked, its ID is changed to highlighted-item
.
Best Practices and Considerations
- Unique IDs: Ensure that the new ID is unique within the page to avoid issues with styling and scripting.
- Event Handlers: Remember that changing an ID might affect event handlers attached to the original ID.
- SEO Impact: Be mindful of the SEO impact when changing IDs, especially if they are used as anchor links. Speaking of SEO, make sure your JavaScript practices are aligned with SEO best practices.
Conclusion
You now have the know-how to change an element's ID using JavaScript, a small but mighty tool in your web development arsenal. As you practice, remember to keep your code organized and always consider the broader impact of your DOM manipulations.
For further reading and to deepen your understanding, explore reputable sources such as MDN Web Docs or W3Schools for more insights into JavaScript and the DOM.
Happy coding, and may your web development journey be filled with learning and creativity!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
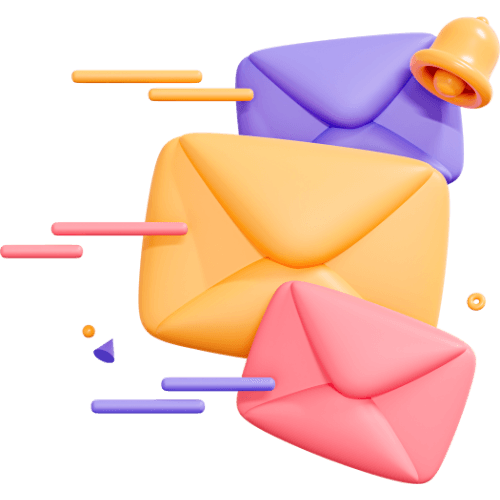
Related articles
9 Articles
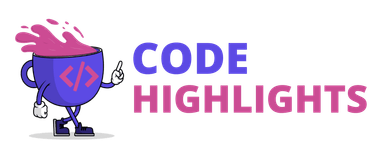
Copyright © Code Highlights 2025.