JavaScript String charAt() Method (With Examples)

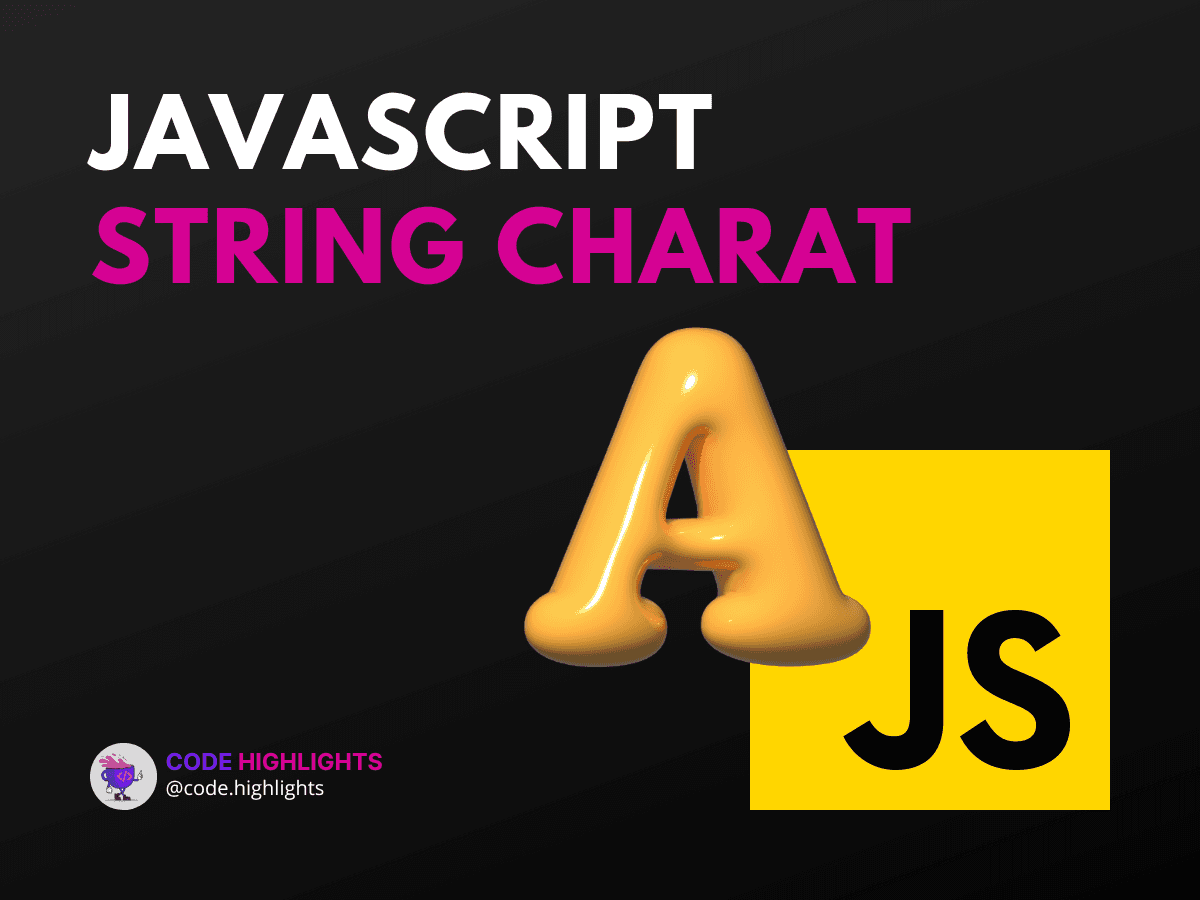
- Understanding charAt()
- Practical Examples
- Example 1: Accessing the First Character
- Example 2: Using charAt() in a Loop
- Example 3: Handling No Index Found
- When to Use charAt()
- Further Learning
- Conclusion
The charAt()
function allows you to access specific characters within a string. This method can come in handy in various coding scenarios, from data validation to parsing input values.
Before we delve deeper, let's quickly see the charAt()
method in action:
1let greeting = "Hello, World!";
2let letter = greeting.charAt(7);
3console.log(letter); // Outputs: W
In the example above, we used charAt()
to get the character at index 7 of the string greeting
, which is 'W'. Now, let's explore this method in detail.
Understanding charAt()
The charAt()
method is a built-in function in JavaScript that returns the character at a specified index within a string. If you're wondering, "Was macht charAt?" or "What does charAt do?" in English, it simply retrieves the character at the position you ask for. The index starts from 0, meaning charAt(0)
will give you the first character of the string.
Here's a basic syntax of the charAt()
method:
string
: This is the string from which you want to retrieve the character.index
: This is the position of the character you want to get. If this parameter is not a number, it will be converted to an integer.
If you're curious about "Was ist String JavaScript?" or "What is String in JavaScript?"—a String is a sequence of characters used to represent text. It is one of the primitive data types in JavaScript and is used for manipulating text.
Practical Examples
Now, let's explore some practical examples where charAt()
becomes useful:
Example 1: Accessing the First Character
Example 2: Using charAt()
in a Loop
This loop logs each character of the word "Iteration" to the console.
Example 3: Handling No Index Found
If you try to access an index that doesn't exist, charAt()
will return an empty string:
1let name = "Alice";
2let nonExistentChar = name.charAt(10);
3console.log(nonExistentChar === ""); // Outputs: true
When to Use charAt()
Understanding when to use charAt()
is crucial. You might need to use it when:
- You're interested in retrieving a single character from a string.
- You're performing operations like string manipulation or validation.
- You're iterating through a string and need to examine each character.
Further Learning
If you're looking to expand your JavaScript knowledge, consider exploring our JavaScript course. For those who are just starting out with web development, our Introduction to Web Development course covers the basics, including HTML and CSS, which you can learn more about in our HTML Fundamentals Course and CSS Introduction Course.
Conclusion
The charAt()
method is a simple yet powerful string function in JavaScript. It's used to access a character at a particular index, which can be incredibly useful in various programming tasks. By mastering charAt()
, you're one step closer to becoming proficient in string manipulation in JavaScript.
For further reading on JavaScript strings and the charAt()
method, check out these resources from reputable sources:
- Mozilla Developer Network (MDN) on charAt()
- W3Schools JavaScript String charAt() Method
- JavaScript.info String
Remember, practice makes perfect. Try implementing the charAt()
method in your projects to see how it can help you achieve your coding goals. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
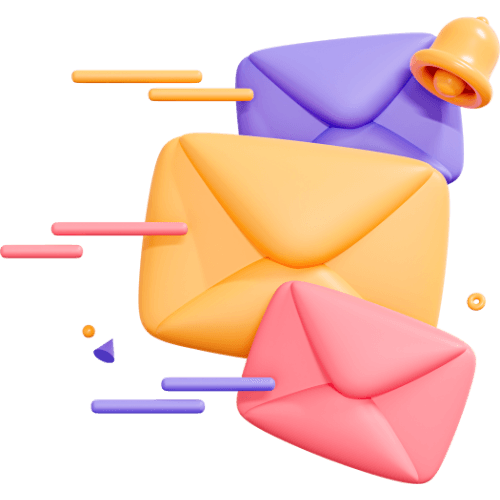
Related articles
9 Articles
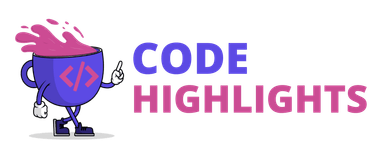
Copyright © Code Highlights 2025.