How to Check if String is Number JavaScript for Accurate Validation

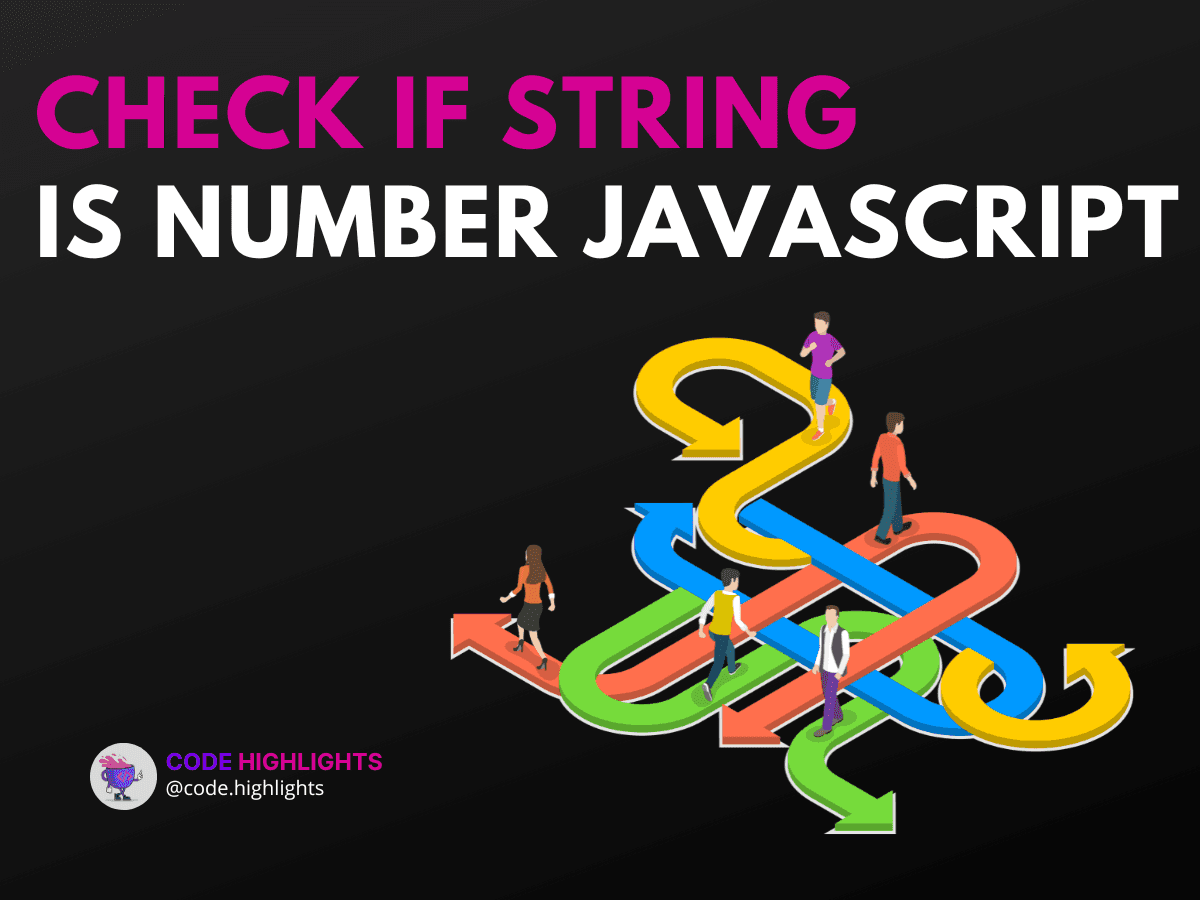
- Syntax and Methods
- Example 1: Using isNaN()
- Example 2: Using Number()
- Example 3: Using parseFloat()
- Example 4: Using Regular Expressions
- Example 5: Combining Methods
- Browser Compatibility
- Summary
In programming, it's often necessary to determine if a string represents a number. This can help prevent errors in calculations or data processing. In JavaScript, there are several ways to check if a string is a number. Understanding these methods will enhance your coding skills and ensure your applications run smoothly.
Here's a quick code example to kick things off:
Now, let's dive deeper into the syntax and various methods available for this task.
Syntax and Methods
In JavaScript, you can use several approaches to check if a string is a number. The most common methods include:
- isNaN(): This function checks if a value is NaN (Not-a-Number).
- Number(): This function converts a string to a number.
- parseFloat() and parseInt(): These functions parse a string and return a floating-point or integer number, respectively.
Example 1: Using isNaN()
In this example, isNaN()
checks if the string can be converted to a number. If it can, the result is false
, so we use the negation operator !
to get true
.
Example 2: Using Number()
1let str = "100";
2let num = Number(str);
3console.log(num); // Output: 100
4console.log(!isNaN(num)); // Output: true
Here, we convert the string to a number using Number()
. Then, we check if the result is not NaN.
Example 3: Using parseFloat()
1let str = "3.14";
2let num = parseFloat(str);
3console.log(num); // Output: 3.14
4console.log(!isNaN(num)); // Output: true
In this case, parseFloat()
converts the string to a floating-point number. We again check for NaN to confirm it's a valid number.
Example 4: Using Regular Expressions
1let str = "123abc";
2let isValidNumber = /^[+-]?\d+(\.\d+)?$/.test(str);
3console.log(isValidNumber); // Output: false
This example uses a regular expression to validate the string format. It checks if the string is a valid number, allowing for optional signs and decimals.
Example 5: Combining Methods
1function checkIfStringIsNumber(str) {
2 return !isNaN(str) && !isNaN(Number(str));
3}
4
5console.log(checkIfStringIsNumber("456")); // Output: true
6console.log(checkIfStringIsNumber("abc")); // Output: false
In this function, we combine isNaN()
and Number()
to ensure that the string is a valid number.
Browser Compatibility
Most modern web browsers support these methods. You can confidently use them across major browsers like Chrome, Firefox, Safari, and Edge. However, always test your code to ensure compatibility with older browser versions.
Summary
In this tutorial, we covered how to check if a string is a number in JavaScript using various methods such as isNaN()
, Number()
, parseFloat()
, and regular expressions. Each method has its unique use cases, making them valuable tools in your programming toolbox.
For more information on JavaScript basics, consider checking out our JavaScript course. If you're interested in web development, our Introduction to Web Development course might also be helpful.
By mastering these techniques, you can ensure accurate validation of user input, leading to more robust and error-free applications. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
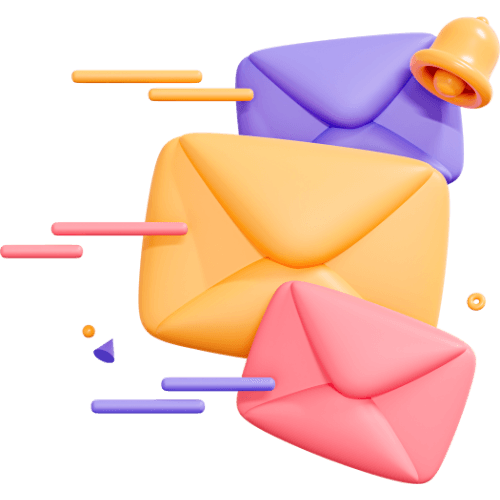
Related articles
9 Articles
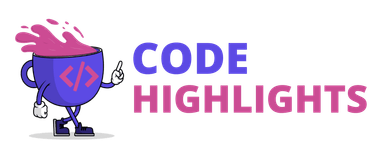
Copyright © Code Highlights 2025.