How to Check If String Number in JavaScript for Accurate Results

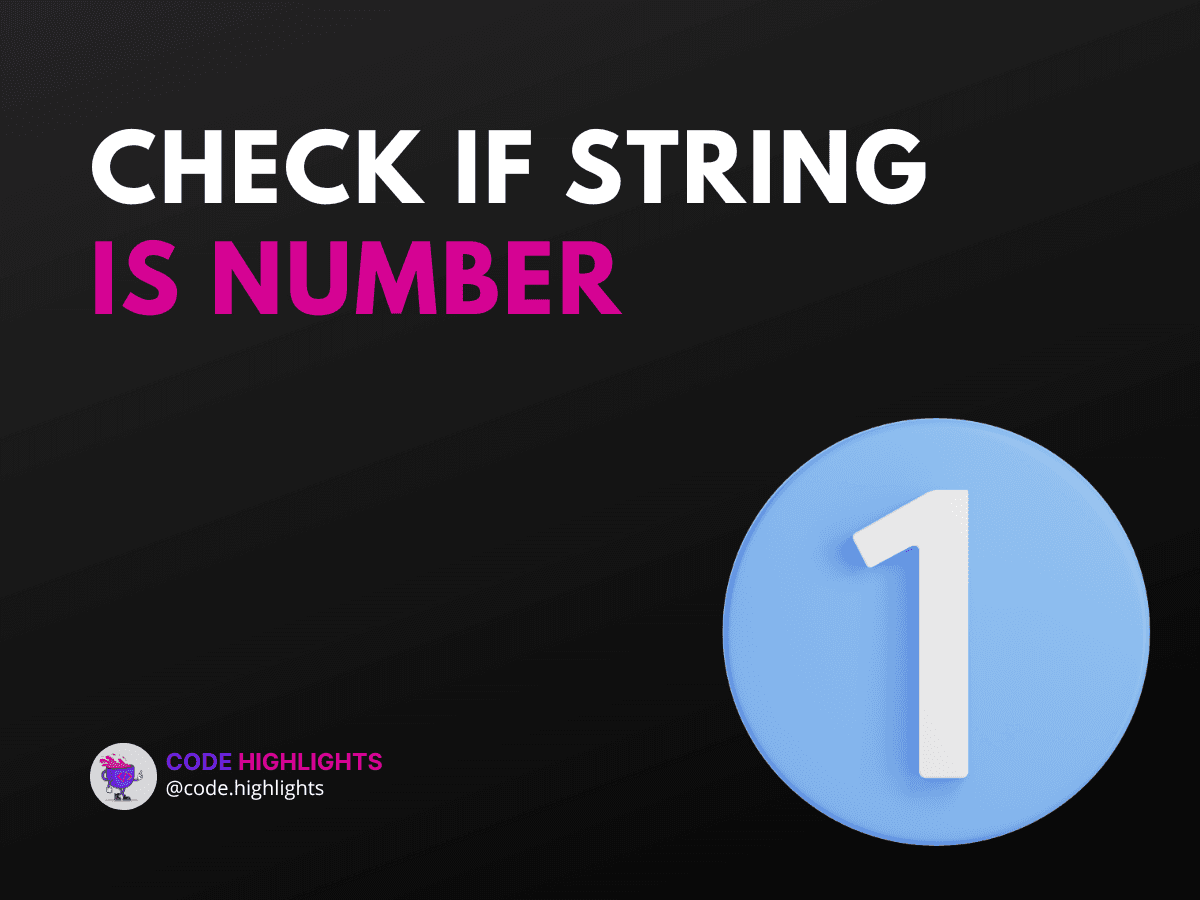
- Quick Code Example
- Understanding the Syntax
- Key Parameters and Return Values
- Example 1: Basic Check for Number
- Using isNaN
- Example 2: Check for Numeric Characters
- Using Regular Expressions
- Example 3: Validate User Input
- Combining Checks
- Compatibility with Major Web Browsers
- Summary
When working with JavaScript, you often need to determine if a string represents a number. This is crucial for ensuring your code behaves as expected, especially when performing mathematical operations. In this tutorial, we will explore different methods to check if a string is a number in JavaScript.
Quick Code Example
Here's a simple example to get started:
1function isStringNumber(str) {
2 return !isNaN(str) && !isNaN(parseFloat(str));
3}
4
5console.log(isStringNumber("123")); // true
6console.log(isStringNumber("abc")); // false
This function checks if the provided string can be converted into a number.
Understanding the Syntax
To check if a string is a number, you can use several methods. The most common ones include:
- isNaN(): Checks if a value is NaN (Not-a-Number).
- parseFloat(): Converts a string to a floating-point number.
- typeof: Determines the type of a variable.
Key Parameters and Return Values
-
isNaN(value):
- Parameter: A value to check.
- Return:
true
if the value is NaN; otherwise,false
.
-
parseFloat(string):
- Parameter: A string to convert.
- Return: The parsed floating-point number or NaN.
-
typeof value:
- Parameter: A variable to check.
- Return: A string indicating the type of the variable.
Example 1: Basic Check for Number
Using isNaN
1function checkNaN(str) {
2 return isNaN(str) ? "Not a number" : "Is a number";
3}
4
5console.log(checkNaN("42")); // Is a number
6console.log(checkNaN("hello")); // Not a number
In this case, isNaN() helps identify if the input is not a number.
Example 2: Check for Numeric Characters
Using Regular Expressions
1function containsNumber(str) {
2 return /\d/.test(str);
3}
4
5console.log(containsNumber("Hello123")); // true
6console.log(containsNumber("Hello")); // false
Here, we use a regular expression to check if there are any numeric characters in the string.
Example 3: Validate User Input
Combining Checks
1function validateInput(input) {
2 if (typeof input === 'string' && !isNaN(input)) {
3 return "Valid number string";
4 }
5 return "Invalid input";
6}
7
8console.log(validateInput("100")); // Valid number string
9console.log(validateInput("abc")); // Invalid input
This example combines typeof and isNaN() to ensure the input is both a string and a number.
Compatibility with Major Web Browsers
The methods discussed are widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. You can confidently use these techniques in your web applications without worrying about compatibility issues.
Summary
In this tutorial, we covered how to check if a string is a number in JavaScript using various methods like isNaN(), parseFloat(), and regular expressions. Understanding these techniques is essential for validating user inputs and ensuring accurate calculations in your applications.
For more information on JavaScript, feel free to check out our JavaScript course or explore the basics of web development in our Introduction to Web Development course.
By mastering these skills, you can enhance your programming abilities and create more robust applications. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
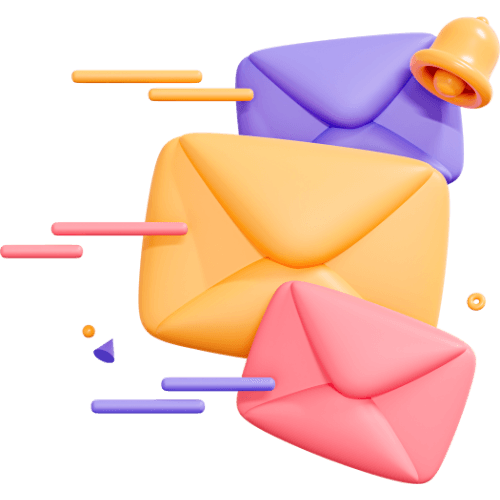
Related articles
9 Articles
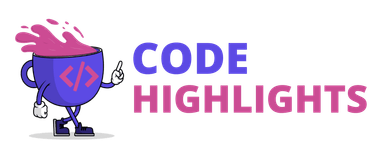
Copyright © Code Highlights 2025.