Avoid Case-Sensitive Errors with touppercase JavaScript Techniques

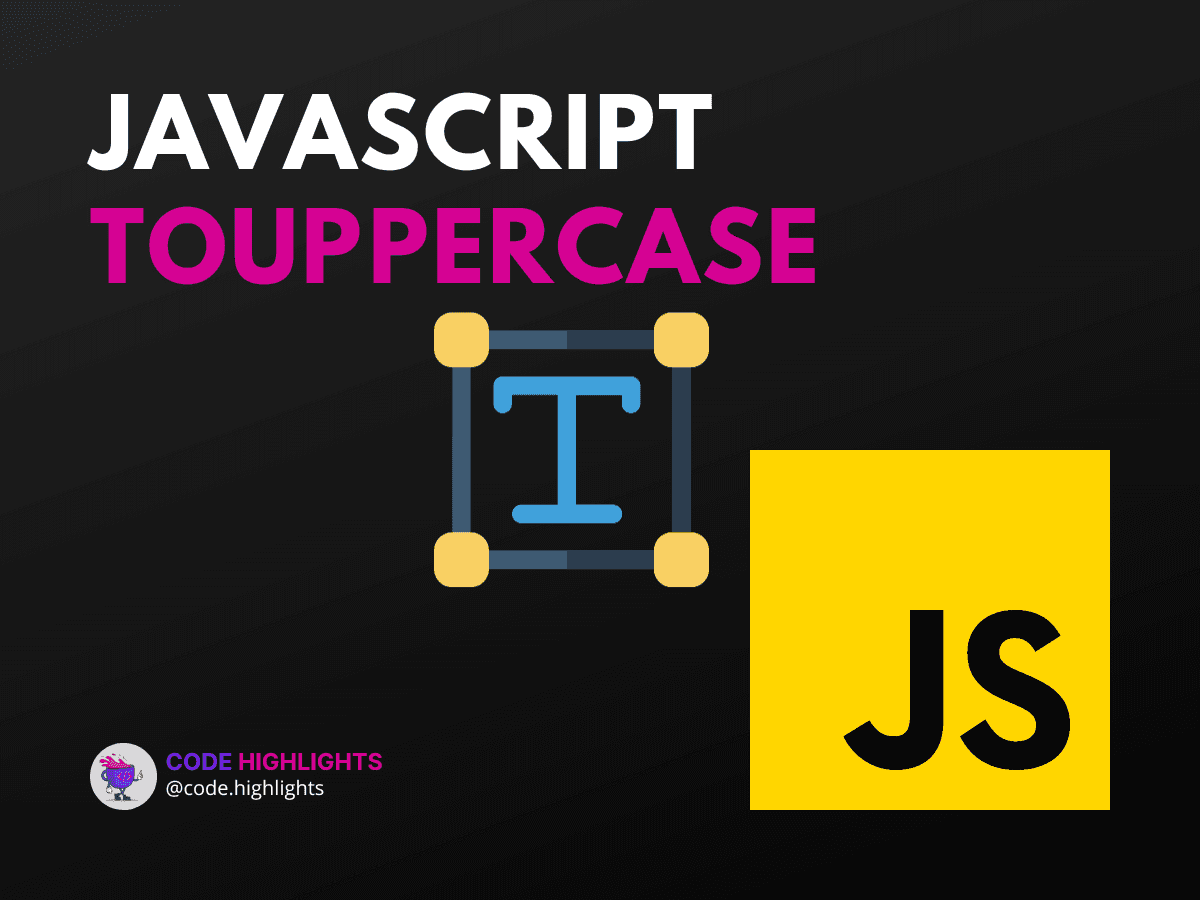
- Syntax: The Building Blocks of touppercase in JavaScript
- Examples: Practical touppercase JavaScript Usage
- Transforming User Input for Consistency
- Capitalizing First Letters (Capitalize in JavaScript)
- Uppercasing and Lowercasing for Data Normalization
- Browser Compatibility: Ensuring Cross-Browser Support
- Conclusion: The Power of touppercase in Your Codebase
Are you ready to take your JavaScript skills to the next level? Understanding how to manipulate text is a fundamental skill in web development. Let's dive into the world of strings and explore how the toUpperCase
function can transform your code. Here's a quick peek at how simple it can be:
This tutorial will guide you through using toUpperCase
in JavaScript, ensuring your applications handle text consistently and professionally.
Syntax: The Building Blocks of touppercase in JavaScript
The toUpperCase
method is straightforward, with no parameters to worry about. It's a string method that converts all characters in a string to uppercase and returns a new string:
1let text = "JavaScript is fun!";
2let upperText = text.toUpperCase();
3console.log(upperText); // JAVASCRIPT IS FUN!
While toUpperCase
does not modify the original string, it provides a new string with all letters converted to their uppercase form. Let's look at some variations and how they behave in different scenarios.
Examples: Practical touppercase JavaScript Usage
Transforming User Input for Consistency
Imagine you're creating a search feature where user input needs to be case-insensitive:
1let userInput = "Search Query";
2let searchKey = userInput.toUpperCase();
3// Use searchKey for comparison
Capitalizing First Letters (Capitalize in JavaScript)
While JavaScript doesn't have a built-in capitalize
function, you can create one combining toUpperCase
with toLowerCase
:
1function capitalize(str) {
2 return str.charAt(0).toUpperCase() + str.slice(1).toLowerCase();
3}
4console.log(capitalize("javaScript")); // JavaScript
Uppercasing and Lowercasing for Data Normalization
When storing data, you might want to normalize text to prevent case-sensitive errors:
1let rawData = "Email@example.com";
2let normalizedData = rawData.toLowerCase();
3console.log(normalizedData); // email@example.com
Browser Compatibility: Ensuring Cross-Browser Support
The toUpperCase
method is widely supported across all major browsers.
Conclusion: The Power of touppercase in Your Codebase
In this tutorial, we've covered the toUpperCase
method in JavaScript, which is an invaluable tool for text manipulation. It's essential for creating user-friendly interfaces and maintaining data integrity. By now, you should feel comfortable implementing toUpperCase
in various scenarios, from enhancing user input to normalizing database entries.
If you found this tutorial useful, you might also like our Javascript Fundamentals Course, which features a ton of useful methods like these.
Remember, mastering string methods like toUpperCase
and toLowerCase
can significantly impact the functionality and reliability of your JavaScript applications. Keep practicing, and don't hesitate to refer back to this guide whenever you need a refresher on using toUpperCase
in JavaScript.
For further reading on JavaScript String toUpperCase, I recommend articles from MDN Web Docs.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
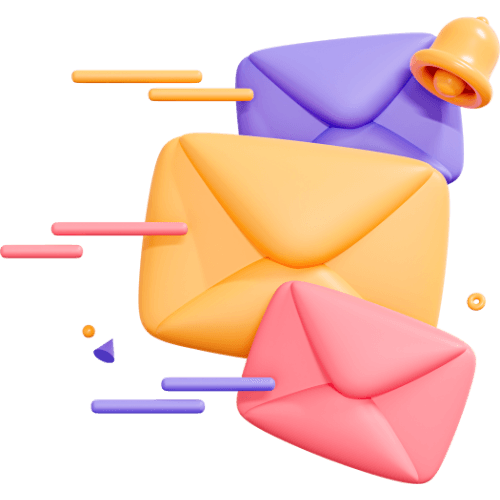
Related articles
9 Articles
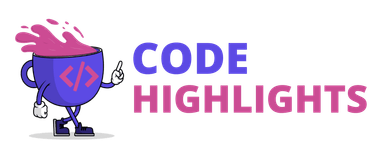
Copyright © Code Highlights 2025.