How to Use Comment in JavaScript Effectively

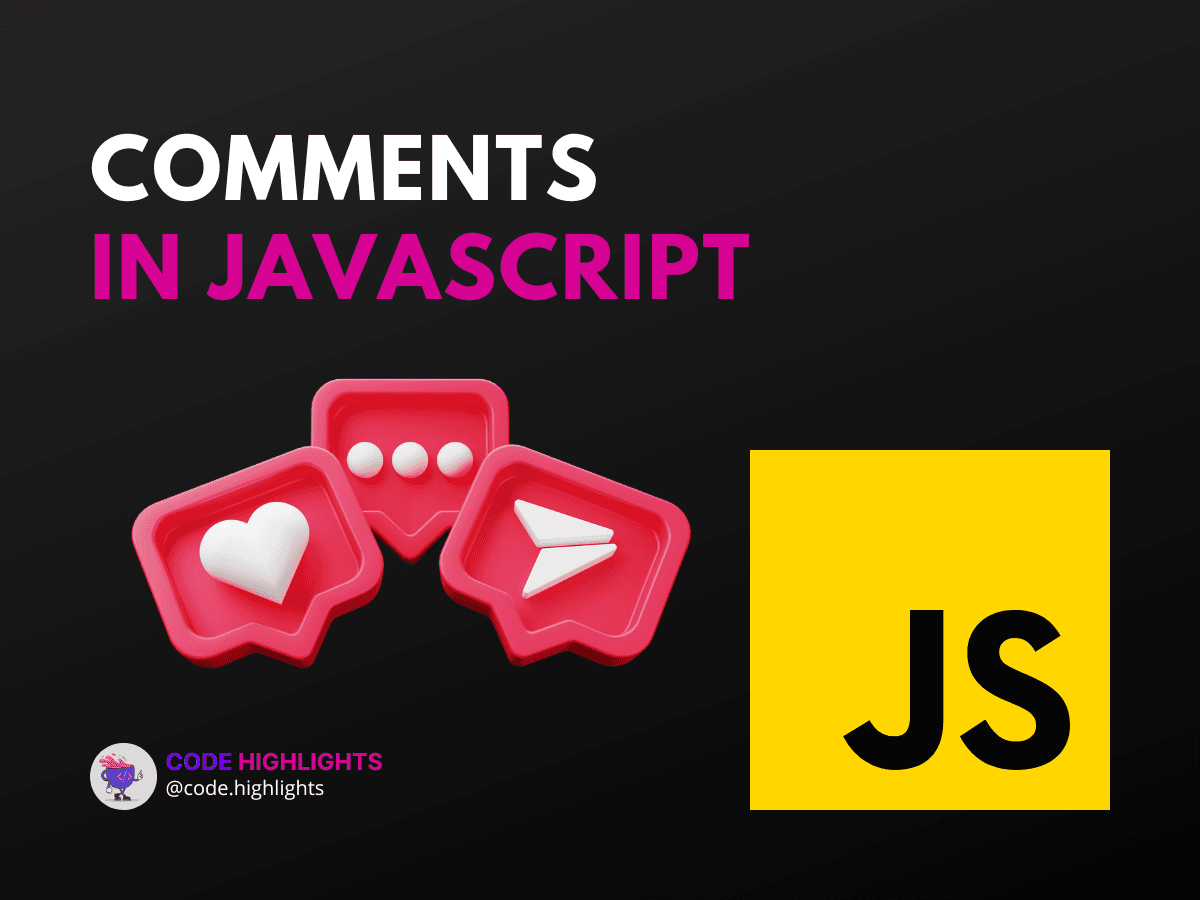
- Single-Line Comments
- Multi-Line Comments
- Commenting Best Practices
- Posting Comments in JavaScript Applications
- External Resources
Welcome to the world of JavaScript, where the art of coding is as much about writing clear, maintainable code as it is about creating powerful web applications. In this tutorial, we'll dive into how to effectively use comments in JavaScript. Whether you're a seasoned developer or just starting out, understanding how to properly comment your code is a vital skill that will help you and your team in the long run.
Let's start with a quick example of JavaScript syntax for commenting:
1// This is a single-line comment in JavaScript
2
3/*
4 And this is a multi-line comment.
5 It can span several lines!
6*/
Now that you've had a glimpse of commenting in action, let's explore the various ways to use comments and why they're so important.
Single-Line Comments
Single-line comments are created using two forward slashes //
. Anything following these slashes on the same line will be ignored by the JavaScript engine.
Single-line comments are perfect for brief explanations or annotations within your code.
Multi-Line Comments
To comment multiple lines in JavaScript, you can use the /* */
syntax. This is especially useful when you need to block out sections of code or add detailed descriptions.
1/*
2 This function calculates the area of a rectangle.
3 It takes the width and height as parameters.
4*/
5function calculateArea(width, height) {
6 return width * height;
7}
Multi-line comments can also be used to temporarily comment out a section in JavaScript during debugging or development.
Commenting Best Practices
It's important to strike a balance with comments. Over-commenting can clutter your code, while under-commenting can leave it cryptic to others and even to your future self. Here are some tips:
- Comment complex logic or algorithms that aren't immediately clear.
- Use comments to explain the "why" behind certain code decisions.
- Avoid stating the obvious; trust that readers understand basic JavaScript syntax.
- Keep your comments up-to-date. Outdated comments can be more harmful than no comments at all.
Posting Comments in JavaScript Applications
Sometimes, you might wonder how to post a comment in JavaScript within the context of user interactions. For instance, allowing users to add comments on a blog post. This is a different use case where JavaScript interacts with HTML elements to capture and display user input.
1// Function to post a user's comment
2function postComment() {
3 let userComment = document.getElementById('comment-box').value;
4 // Code to display the comment goes here
5}
For a deeper dive into how JavaScript interacts with HTML, consider taking a course on HTML fundamentals.
External Resources
To bolster your understanding of JavaScript comments and best practices, check out these resources:
- Mozilla Developer Network - JavaScript Guide
- W3Schools - JavaScript Syntax
- Codecademy - Learn JavaScript
Remember, commenting is an art that improves with practice. As you continue to write more JavaScript, you'll develop a sense for when and how to comment effectively.
By now, you should have a solid grasp of how to comment text in JavaScript and why it's such a crucial part of writing clean, understandable code. Keep honing your skills, and don't forget to check out other courses to broaden your web development knowledge!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
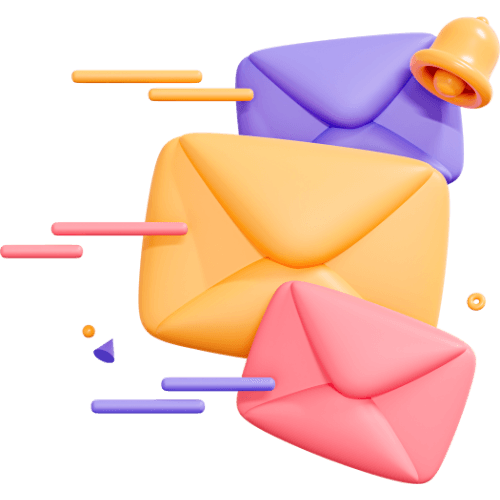
Related articles
9 Articles
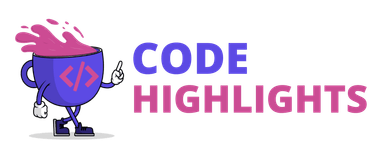
Copyright © Code Highlights 2025.