How to Check if Uppercase JavaScript: Boost Your Coding Skills

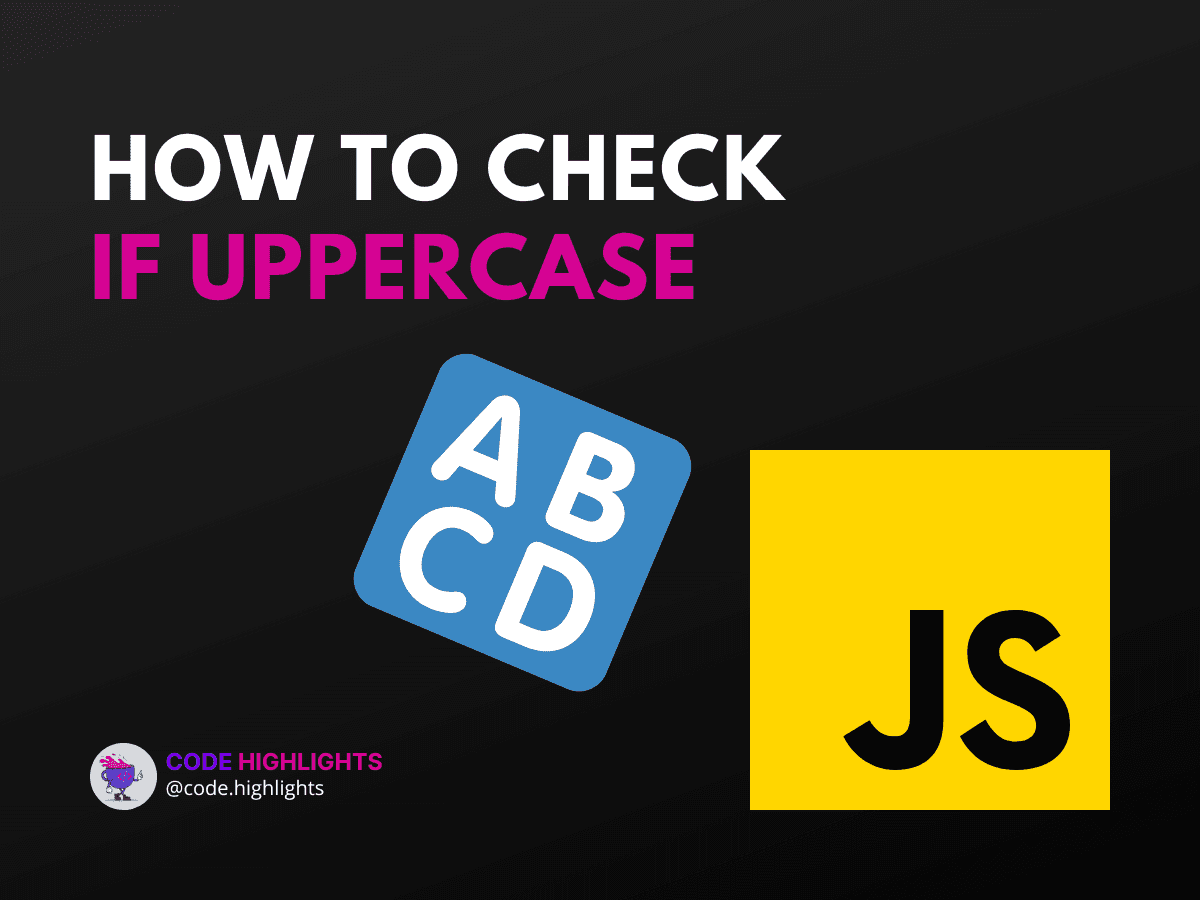
- Checking if a Character is Uppercase in JavaScript
- Checking if a String is in Uppercase
- Checking if a String Starts with an Uppercase Letter in JS
- Checking if a Character is Uppercase or Lowercase
Welcome to this tutorial where we'll dive into the practical world of JavaScript, exploring how to determine whether a character or string is uppercase. This skill is essential for various applications, such as form validation, text analysis, and user input processing. Let's kick things off with a quick code snippet that highlights the syntax you'll become familiar with:
This example sets the stage for what we'll accomplish together. Now, let's enhance your coding expertise by tackling some common questions related to uppercase checks in JavaScript.
Checking if a Character is Uppercase in JavaScript
To determine if a character is uppercase, we can use the toUpperCase()
method. This method converts the entire string to uppercase and allows for a simple comparison:
1function isUppercase(char) {
2 return char.length === 1 && char === char.toUpperCase();
3}
4console.log(isUppercase('A')); // true
5console.log(isUppercase('a')); // false
This function checks for a single character and compares it to its uppercase version. If they match, the character is indeed uppercase.
For those who are just starting out with JavaScript and want to solidify their understanding, consider checking out this JavaScript course.
Checking if a String is in Uppercase
What if we have an entire string to verify? The process is quite similar:
1function isStringUppercase(str) {
2 return str === str.toUpperCase();
3}
4console.log(isStringUppercase('HELLO WORLD')); // true
5console.log(isStringUppercase('Hello World')); // false
This function ensures that every character in the string is uppercase by comparing the original string to its uppercase counterpart.
Checking if a String Starts with an Uppercase Letter in JS
Sometimes, you only need to check the first character of a string. Here's how you can do that:
1function startsWithUppercase(str) {
2 return str.charAt(0) === str.charAt(0).toUpperCase();
3}
4console.log(startsWithUppercase('JavaScript')); // true
5console.log(startsWithUppercase('javaScript')); // false
The charAt(0)
method retrieves the first character of the string, which we then compare to its uppercase form.
If you're looking to build a solid foundation in web development, our Introduction to Web Development course is a great resource to start with.
Checking if a Character is Uppercase or Lowercase
To differentiate between uppercase and lowercase characters, we can use the toLowerCase()
method in conjunction with toUpperCase()
:
1function checkCase(char) {
2 if (char === char.toUpperCase()) {
3 return 'Uppercase';
4 } else if (char === char.toLowerCase()) {
5 return 'Lowercase';
6 } else {
7 return 'Not a letter';
8 }
9}
10console.log(checkCase('A')); // Uppercase
11console.log(checkCase('b')); // Lowercase
12console.log(checkCase('3')); // Not a letter
This function not only checks for uppercase but also accounts for lowercase characters and non-letter entities.
For more foundational knowledge on HTML and CSS, which are integral to web development alongside JavaScript, take a look at our courses on HTML fundamentals and a gentle introduction to CSS.
By now, you should have a good grasp of how to check if uppercase JavaScript. Remember, practice is key to mastering these concepts, so try incorporating these methods into your projects.
For further reading and best practices, reputable sources like MDN Web Docs provide extensive documentation on JavaScript methods. Additionally, W3Schools offers examples and tutorials on toUpperCase()
and toLowerCase()
functions.
Keep experimenting with the code examples provided, and before you know it, you'll be handling text cases in JavaScript like a pro!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
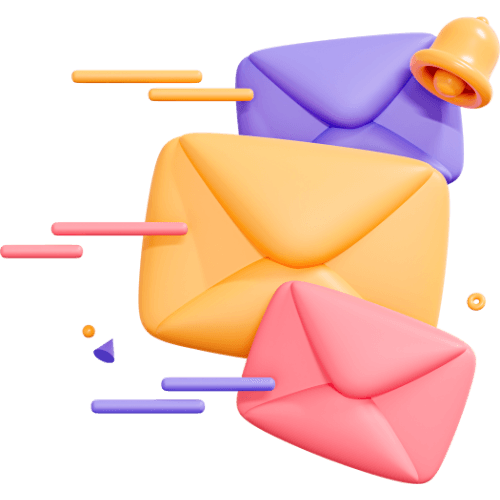
Related articles
125 Articles
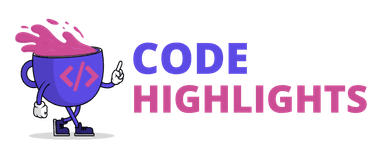
Copyright © Code Highlights 2024.