How to Leverage Closure in JS for Better Code

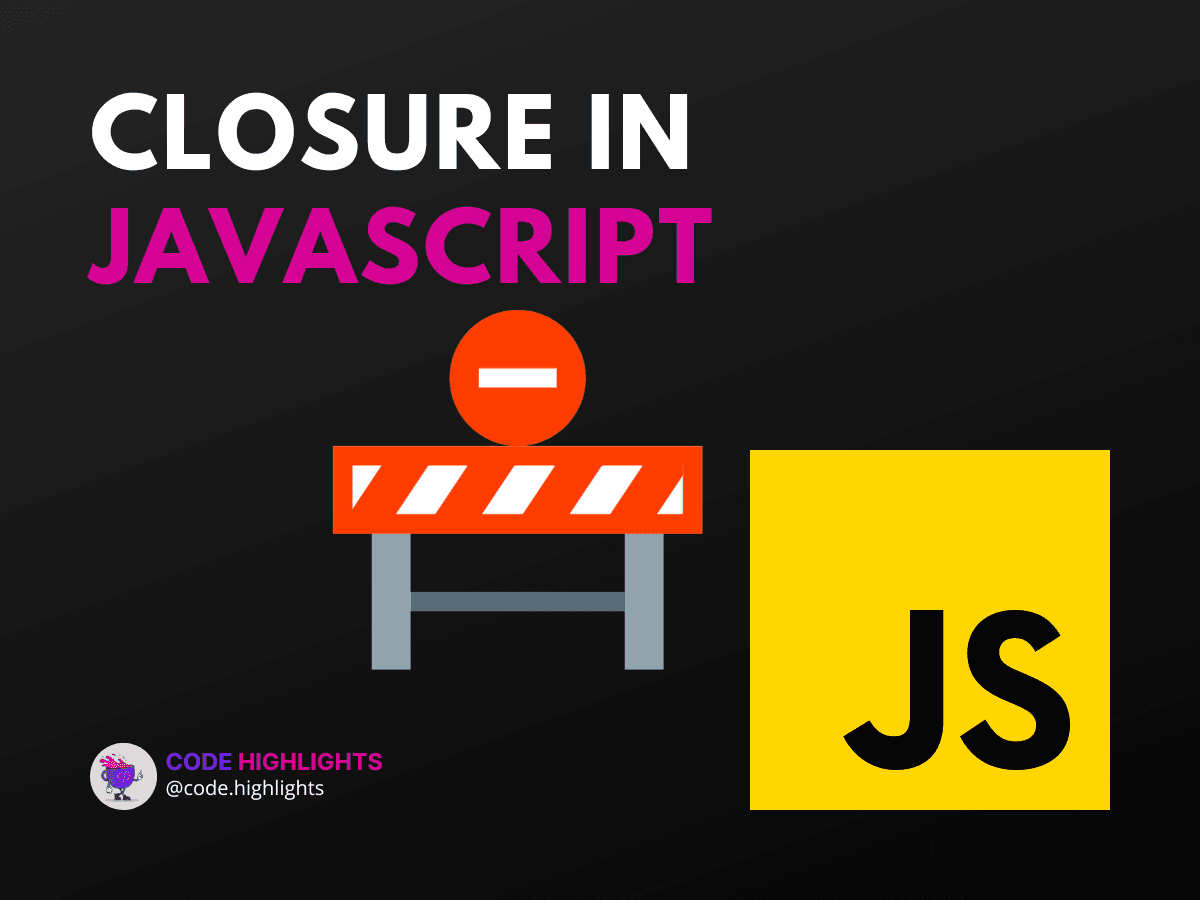
- Understanding Closures in JavaScript
- How Do You Create a Closure?
- Closure vs Hoisting
- Function vs Closure
- Practical Uses of Closures
Welcome to this step-by-step tutorial where we will explore the concept of closure in JS, a powerful feature that can significantly enhance your coding capabilities. Whether you're just starting out or looking to refine your JavaScript skills, understanding closures is key to writing more efficient and maintainable code. Before we dive deep into closures, let's take a quick look at a basic example:
1function createGreeting(message) {
2 return function(name) {
3 console.log(message + ', ' + name);
4 };
5}
6const greetHello = createGreeting('Hello');
7greetHello('Alice'); // Output: Hello, Alice
In this snippet, createGreeting
is a function that generates another function, capable of remembering the message
passed to it even after the outer function has finished executing. This is closure in action!
Understanding Closures in JavaScript
A closure in JS is essentially a function bundled together (enclosed) with references to its surrounding state (lexical environment). In other words, a closure gives you access to an outer function's scope from an inner function. To learn more about JavaScript and its features, consider checking out this comprehensive course.
How Do You Create a Closure?
To create a closure, simply define a function inside another function and expose it. To expose a function, return it or pass it to another function. The inner function will have access to the variables in the outer function scope, even after the outer function has completed execution.
1function outerFunction(outerVariable) {
2 return function innerFunction(innerVariable) {
3 console.log('Outer Variable: ' + outerVariable);
4 console.log('Inner Variable: ' + innerVariable);
5 };
6}
7const newFunction = outerFunction('outside');
8newFunction('inside');
Closure vs Hoisting
Closures and hoisting are two distinct concepts in JavaScript. While closure deals with the scope of variables, hoisting is about how variable and function declarations are moved to the top of their containing scope before code execution. Unlike hoisting, closures do not move code physically; they capture the local variables at the point where a function is defined.
Function vs Closure
The difference between a function and a closure in JavaScript lies in the environment they carry. All functions have access to the global scope and their own local scope. However, a closure retains access to the outer function's scope even after the outer function has executed.
Practical Uses of Closures
Closures are not just theoretical constructs; they have practical uses in real-world programming. They enable private data encapsulation, currying, event handling, and much more. For those interested in web development basics, including HTML and CSS, which often interact with JavaScript, you can find foundational courses here and here.
Let's look at an example where closures can manage private data:
1function createCounter() {
2 let count = 0;
3 return {
4 increment() {
5 count++;
6 },
7 getCount() {
8 return count;
9 },
10 };
11}
12
13const counter = createCounter();
14counter.increment();
15console.log(counter.getCount()); // Output: 1
In the above example, count
is private. Only the increment
and getCount
methods exposed through the closure have access to it.
To sum up, closures are a fundamental concept in JavaScript that allows for more dynamic and flexible code. By understanding and leveraging closures, you can create private variables, partially apply functions, and much more, leading to better-structured and more robust applications. If you're keen on starting your journey into web development, don't miss out on this introductory course.
Remember, practice makes perfect. Implement closures in your projects, experiment with them, and watch your JavaScript skills grow!
For further reading on closures and advanced JavaScript topics, be sure to check out resources from MDN Web Docs, JavaScript Info, and W3Schools. These external links offer in-depth explanations and examples that can solidify your understanding of closures in JavaScript.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
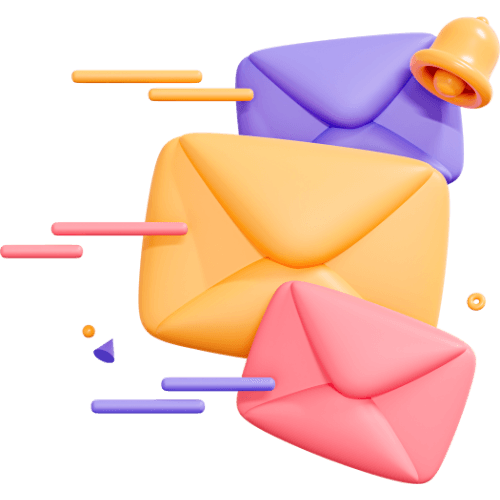
Related articles
9 Articles
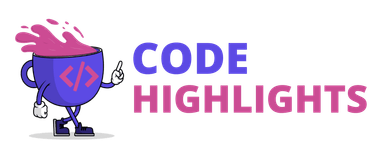
Copyright © Code Highlights 2025.