Avoid Bugs: Mastering Coercion in JavaScript Techniques

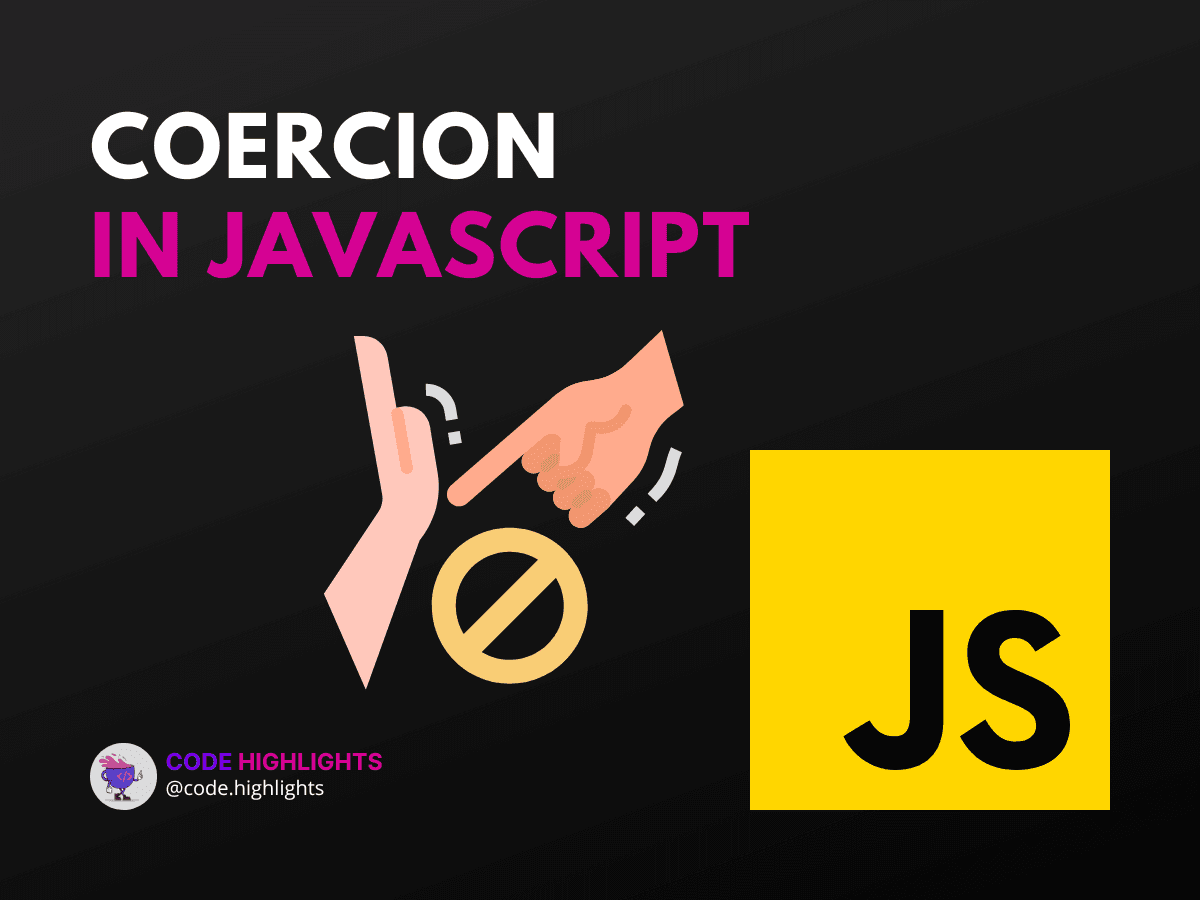
- What is Coercion in JavaScript with Example?
- What is Coercion in Programming?
- How Does Type Coercion Work?
- What is the Difference Between Casting and Coercion?
Have you ever found yourself scratching your head, wondering why your JavaScript code is behaving unpredictably? It's possible that you've encountered a quirky yet fundamental aspect of JavaScript: coercion. In this tutorial, we'll demystify this concept, so you can write cleaner, bug-free code.
Let's start with a simple code example to set the stage:
In the above snippet, JavaScript has coerced the number 2
into a string '2'
, resulting in the concatenation of '3'
and '2'
rather than their sum. This is a basic instance of coercion in action.
What is Coercion in JavaScript with Example?
Coercion in JavaScript refers to the automatic or implicit conversion of values from one data type to another. This happens because JavaScript is a loosely typed language, which means variables are not directly bound to a single data type. For example:
In this case, JavaScript interprets the subtraction operation and coerces both strings into numbers before performing the calculation.
What is Coercion in Programming?
Coercion in programming is not unique to JavaScript. It's a concept where a value of one data type is converted into another data type automatically. This process helps in operations involving multiple data types, ensuring compatibility between them.
How Does Type Coercion Work?
JavaScript employs type coercion in three main contexts: string, number, and boolean. When an operation involves mixed types, JavaScript converts the values to an expected type. For example:
Understanding how JavaScript handles type coercion is crucial to avoid unexpected results in your code.
What is the Difference Between Casting and Coercion?
Casting is when the programmer explicitly converts a value from one type to another, whereas coercion is an implicit conversion by the JavaScript engine. For instance:
1let explicitCast = String(123); // Casting: number to string
2let implicitCoerce = '6' * '2'; // Coercion: strings to numbers for multiplication
Casting is a controlled form of type conversion, while coercion can sometimes lead to bugs if not properly understood.
To further strengthen your understanding of coercion and other JavaScript fundamentals, consider enrolling in a JavaScript course.
Remember to use reliable sources like MDN Web Docs or W3Schools to dive deeper into JavaScript's intricacies. Also, familiarize yourself with HTML and CSS through HTML Fundamentals and CSS Introduction courses to build a strong foundation in web development.
In conclusion, mastering coercion in JavaScript can help you write more predictable and error-free code. By understanding the nuances of implicit type conversion and knowing when to use explicit casting, you'll be able to tackle complex programming challenges with confidence. Keep practicing and exploring, and don't hesitate to refer back to this guide whenever you need a refresher on coercion techniques in JavaScript.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
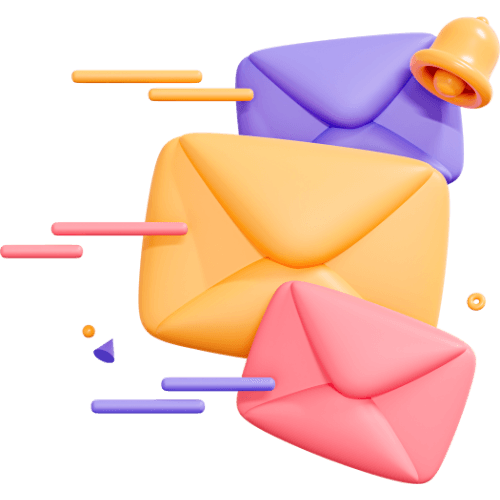
Related articles
9 Articles
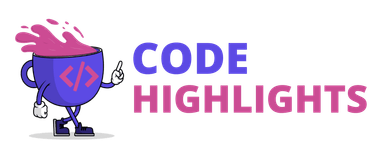
Copyright © Code Highlights 2025.