7 Clever Combinations JavaScript Techniques for Efficient Coding

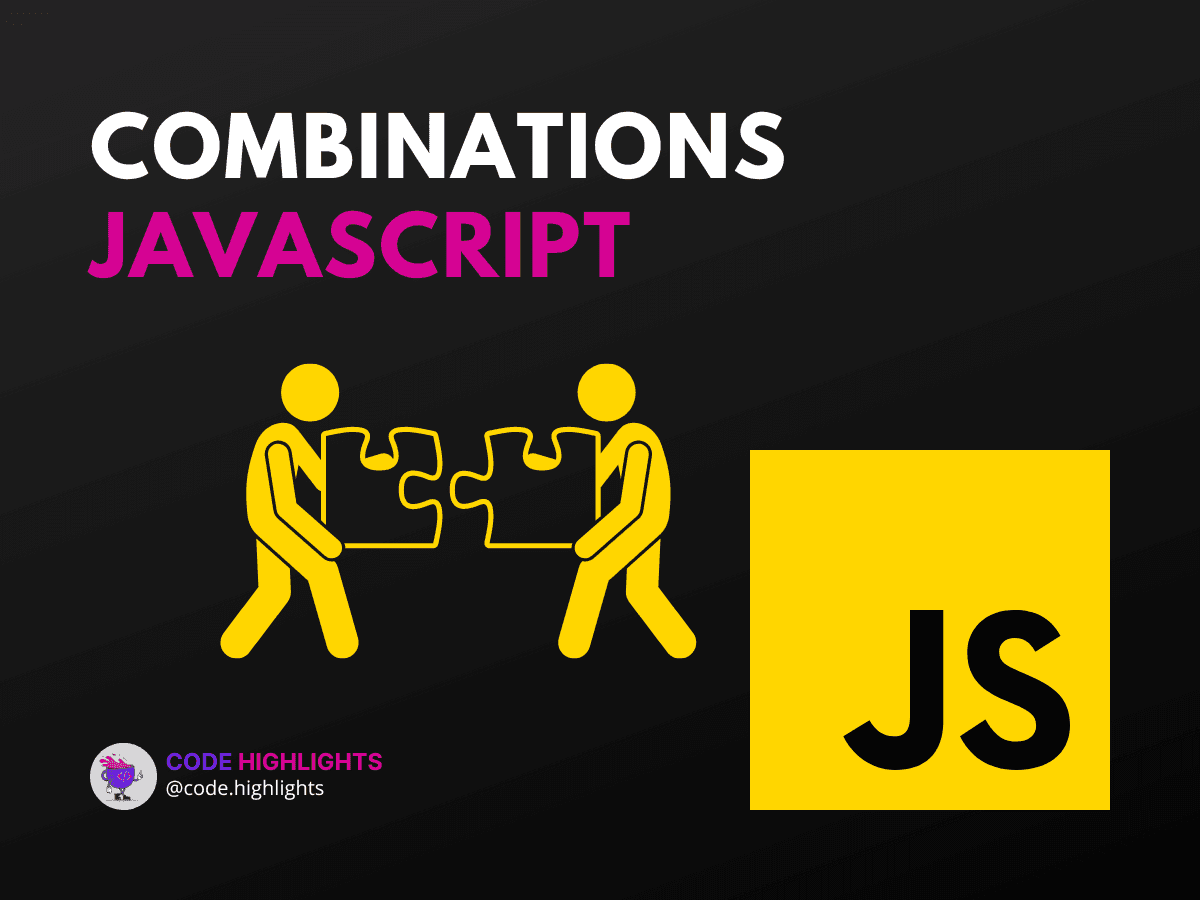
- Understanding Combinations in JavaScript
- Finding All Combinations of a List in JavaScript
- Merging Two Elements in JavaScript
- Finding Permutations of a Number in JavaScript
When it comes to coding in JavaScript, mastery of combinations can unlock a world of possibilities. Whether you're a seasoned developer or just starting, understanding how to efficiently create combinations in JavaScript can save time and enhance the functionality of your applications. In this tutorial, we'll explore various techniques to work with combinations in JavaScript, ensuring that you can tackle any related task with confidence.
1// An introductory code snippet to demonstrate a simple combination
2function combineElements(array) {
3 if (array.length === 0) return [[]];
4 const firstElement = array[0];
5 const rest = array.slice(1);
6 const withoutFirst = combineElements(rest);
7 const withFirst = withoutFirst.map(el => [firstElement, ...el]);
8 return [...withoutFirst, ...withFirst];
9}
10
11console.log(combineElements(['a', 'b', 'c']));
This snippet is just a teaser of what's to come. Stick with me, and by the end of this guide, you'll be adept at using combinations in your JavaScript projects.
Understanding Combinations in JavaScript
Before diving into the techniques, let's answer an essential question: How do you use combinations in JavaScript? Combinations are ways to select items from a collection such that the order does not matter. In JavaScript, this often involves writing functions that generate all possible subsets of an array.
Finding All Combinations of a List in JavaScript
To find all combinations of a list in JavaScript, you need to understand recursion. Recursion allows a function to call itself, which is perfect for breaking down the problem into smaller, more manageable chunks.
1// Recursive function to find all combinations of a list
2function findAllCombinations(list) {
3 if (list.length === 0) return [[]];
4 let firstElem = list[0];
5 let restCombs = findAllCombinations(list.slice(1));
6 let combsWithFirst = restCombs.map(comb => [firstElem, ...comb]);
7 return restCombs.concat(combsWithFirst);
8}
This function elegantly demonstrates how to generate every combination possible from a given list. For a deeper dive into JavaScript, consider enrolling in this comprehensive course.
Merging Two Elements in JavaScript
Sometimes, you might want to merge two elements or arrays in JavaScript. The concat()
method is commonly used for this purpose:
1// Merging two arrays in JavaScript
2let array1 = ['a', 'b'];
3let array2 = ['c', 'd'];
4let mergedArray = array1.concat(array2);
This method creates a new array containing the elements of both array1
and array2
. If you're interested in the building blocks of web development, including HTML and CSS, check out this fundamentals course and this introduction to CSS.
Finding Permutations of a Number in JavaScript
Permutations are similar to combinations but with a key difference: order matters. To find permutations of a number in JavaScript, you can write a function that generates all possible orders of an array's elements.
1// Function to find permutations of a number
2function findPermutations(array) {
3 if (array.length <= 1) return [array];
4 let perms = [];
5 for (let i = 0; i < array.length; i++) {
6 let currentNum = array[i];
7 let remainingNums = array.slice(0, i).concat(array.slice(i + 1));
8 let remainingNumsPerms = findPermutations(remainingNums);
9 for (let perm of remainingNumsPerms) {
10 perms.push([currentNum].concat(perm));
11 }
12 }
13 return perms;
14}
This function uses a recursive approach to generate permutations. For a more general understanding of web development, you might find this introductory course helpful.
As you continue to practice these techniques, remember that there are many resources available to help you. Consider exploring reputable sources like Mozilla Developer Network (MDN), Stack Overflow, and W3Schools for additional insights and support.
In conclusion, mastering combinations and permutations in JavaScript is a valuable skill that can significantly improve your coding efficiency. By understanding the concepts and techniques covered in this tutorial, you'll be well-equipped to solve complex problems and build sophisticated features in your applications. Keep experimenting and refining your approach, and you'll soon see the power of combinations in JavaScript come to life in your projects.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
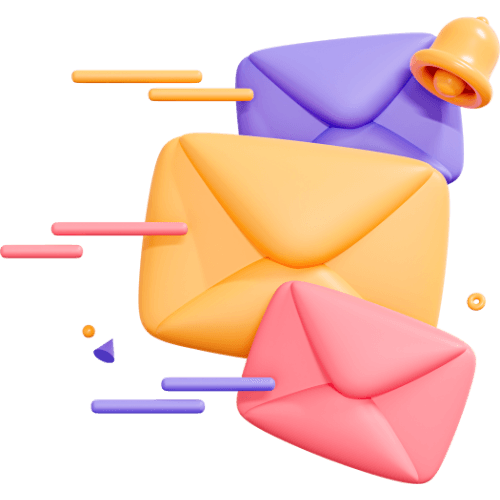
Related articles
9 Articles
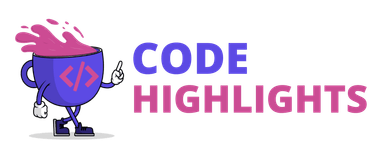
Copyright © Code Highlights 2025.