How to Avoid Common JavaScript Mistakes and Save Time

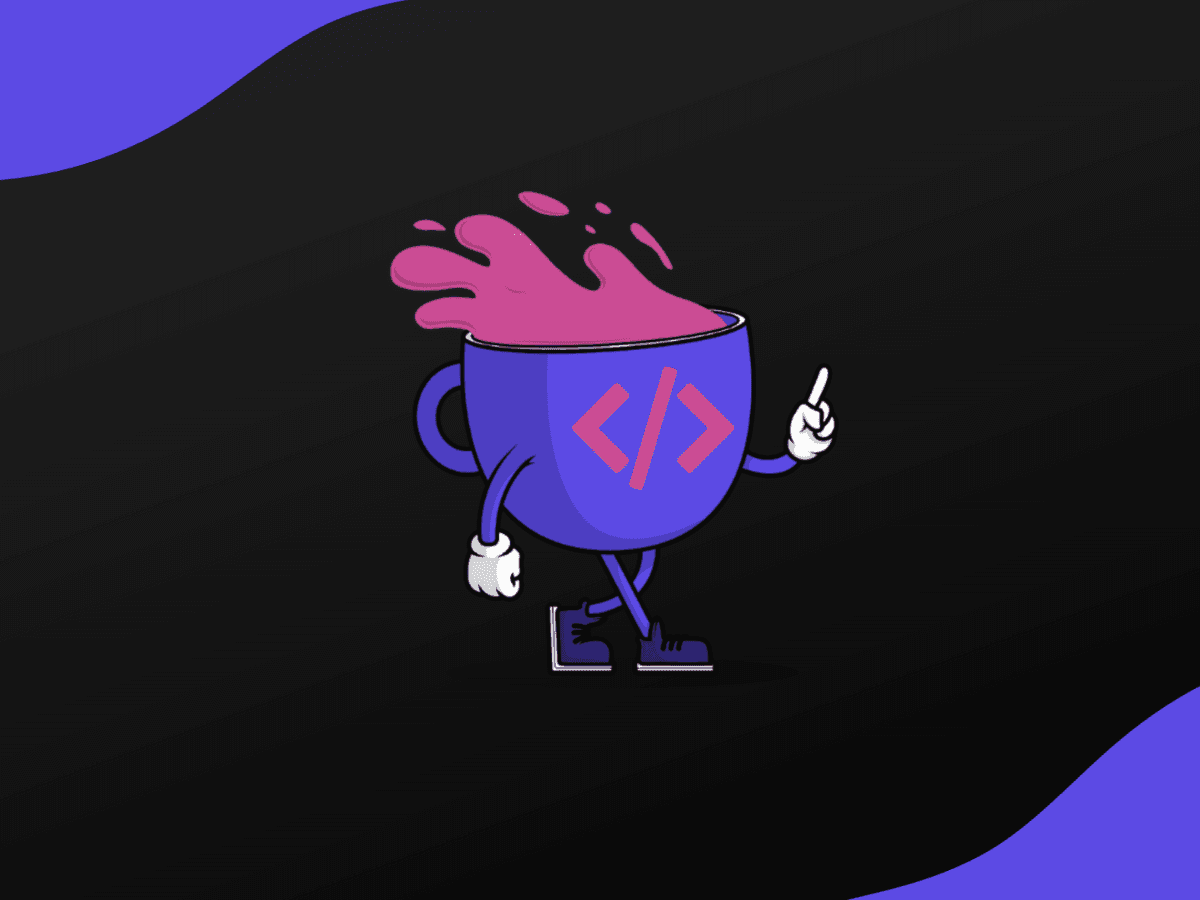
- Mistake #1: Not Declaring Variables
- Mistake #2: Overusing the Global Scope
- Mistake #3: Not Understanding Callbacks
- Mistake #4: Ignoring Error Handling
- Conclusion
JavaScript is a powerful language that is used extensively in web development. However, even experienced developers can make common mistakes that can lead to performance issues or bugs in their code. In this article, we will discuss some of the most common JavaScript mistakes and how to avoid them.
Mistake #1: Not Declaring Variables
One of the most common mistakes in JavaScript is not declaring variables before using them. This can lead to unexpected behaviors in your code and make it more difficult to read and understand.
To avoid this mistake, always declare your variables with the var
, let
, or const
keywords before using them. This will ensure that your variables are created and initialized properly.
1// Example of declaring variables
2let name = "John";
3const age = 30;
4var job = "Software Engineer";
Mistake #2: Overusing the Global Scope
Another common mistake is overusing the global scope in your code. The global scope is the outermost scope of your code and any variables or functions that are declared in it can be accessed anywhere in your code.
While this may seem convenient, it can lead to naming collisions and make your code more difficult to debug and maintain.
To avoid this mistake, try to limit the use of global variables and instead use local variables that are only accessible within their own function or block scope.
1// Example of using a local variable
2function sayHello() {
3 let message = "Hello, world!";
4 console.log(message);
5}
6
7sayHello(); // Output: "Hello, world!"
8
9// Example of overusing the global scope
10let message = "Hello, world!";
11
12function sayHello() {
13 console.log(message);
14}
15
16sayHello(); // Output: "Hello, world!"
Mistake #3: Not Understanding Callbacks
Callbacks are a common programming pattern in JavaScript that allow you to pass one function as an argument to another function. While callbacks can be powerful, they can also be difficult to understand and lead to confusing code.
To avoid this mistake, make sure you understand how callbacks work and try to use simpler alternatives like promises or async/await when possible.
1// Example of using a callback
2function processResults(data, callback) {
3 // Process data...
4 callback(data);
5}
6
7function printResults(data) {
8 console.log(data);
9}
10
11processResults([1, 2, 3], printResults); // Output: [1, 2, 3]
Mistake #4: Ignoring Error Handling
Error handling is an important aspect of programming that is often overlooked in JavaScript. Ignoring errors can lead to unexpected behaviors in your code and make it more difficult to debug.
To avoid this mistake, always include error handling in your code and make sure to test it thoroughly.
1// Example of error handling
2try {
3 // Code that may throw an exception...
4} catch (error) {
5 console.error(error);
6}
Conclusion
By avoiding these common JavaScript mistakes, you can write cleaner, more efficient, and more maintainable code. Remember to always declare your variables, limit the use of global variables, understand callbacks, and include error handling in your code. With these tips, you'll be on your way to becoming a more proficient JavaScript developer.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
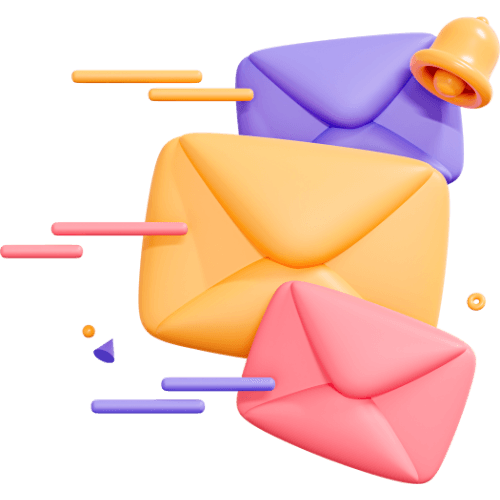
Related articles
122 Articles
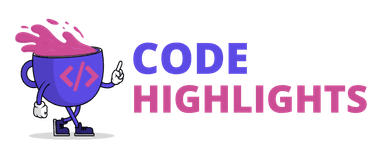
Copyright © Code Highlights 2024.