How to Console Log JavaScript Objects for Better Debugging

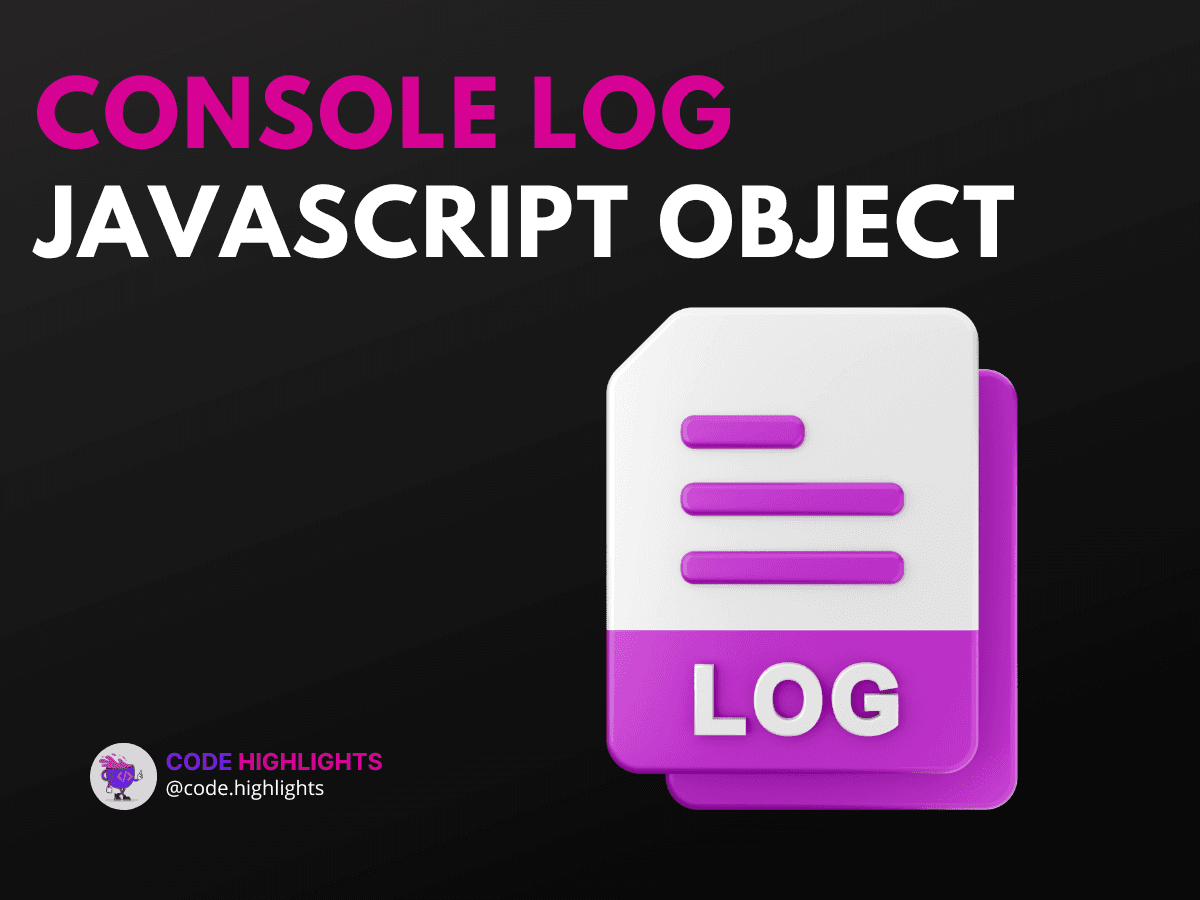
- Getting Started with console.log
- Understanding the Syntax
- Parameters
- Return Values
- Variations
- Example 1: Logging Nested Objects
- Displaying Complex Structures
- Example 2: Logging Text and Objects Together
- Combining Information
- Example 3: Logging Specific Object Values
- Accessing Properties Directly
- Example 4: Logging Elements in the DOM
- Accessing HTML Elements
- Browser Compatibility
- Summary
Debugging is a crucial part of programming, and one of the best tools for finding errors in your code is using the console. In JavaScript, logging objects to the console can provide valuable insights into your code's behavior. This tutorial will guide you through the process of effectively using console.log
to display JavaScript objects, helping you improve your debugging skills.
Getting Started with console.log
To kick things off, let’s look at a simple example of logging a JavaScript object. Here’s a basic code snippet:
In this example, we create an object called user
and log it to the console. When you run this code, you'll see the entire object structure printed out, which is helpful for understanding its properties.
Understanding the Syntax
The syntax for console.log
is straightforward. You simply call the function with the object you want to log as its parameter. The basic structure looks like this:
Parameters
- object: This can be any variable, including strings, numbers, arrays, or other objects.
Return Values
The console.log
function does not return any value; it outputs the data directly to the console.
Variations
You can log multiple values by separating them with commas:
This will print both the string and the object together.
Example 1: Logging Nested Objects
Displaying Complex Structures
Sometimes, your objects might contain other objects or arrays. Here’s how to log them:
1const product = {
2 id: 101,
3 name: "Laptop",
4 specs: {
5 processor: "Intel i7",
6 ram: "16GB"
7 }
8};
9
10console.log(product);
Using console.log(product)
will show the complete structure, allowing you to inspect nested properties easily.
Example 2: Logging Text and Objects Together
Combining Information
You may want to log text alongside an object to clarify what you're displaying. Here’s how:
This logs the message "Car Details:" followed by the car
object, making your console output clearer.
Example 3: Logging Specific Object Values
Accessing Properties Directly
If you want to log just specific values from an object, you can do it like this:
1const student = {
2 name: "Bob",
3 grade: "A",
4 subjects: ["Math", "Science"]
5};
6
7console.log("Student Name:", student.name);
8console.log("Student Grade:", student.grade);
Here, we log only the name and grade, which can help when you only need specific information.
Example 4: Logging Elements in the DOM
Accessing HTML Elements
You can also log DOM elements using JavaScript. Here’s an example:
This will log the HTML element with the ID myElement
, allowing you to inspect its properties and attributes.
Browser Compatibility
The console.log
function is well-supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. This means you can rely on it for debugging in virtually any environment.
Summary
In this tutorial, we've explored how to use console.log
to effectively debug JavaScript objects. We learned about the basic syntax, variations, and several practical examples, including logging nested objects, combining text with objects, and accessing specific values. Mastering these techniques can significantly enhance your debugging process. For further learning, consider exploring more about JavaScript in our JavaScript courses or check out our HTML fundamentals course.
By understanding how to console log JavaScript objects, you’ll be better equipped to troubleshoot your code. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
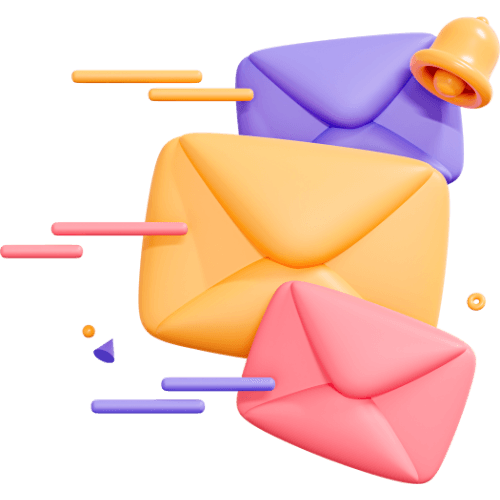
Related articles
9 Articles
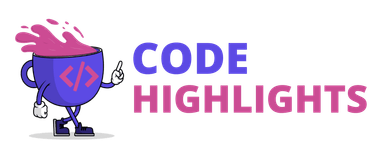
Copyright © Code Highlights 2025.