How to Convert HTML to JavaScript for Better Performance

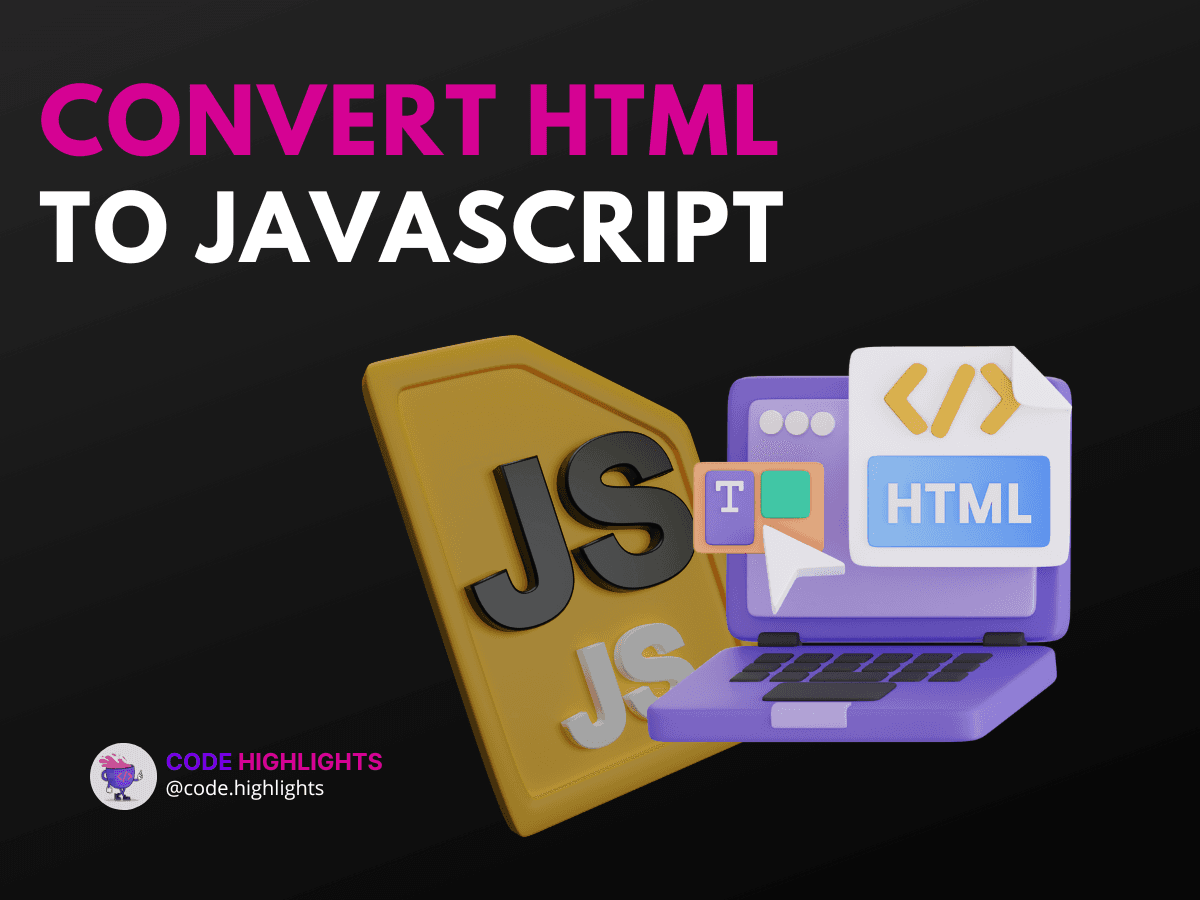
- A Quick Code Example
- Understanding the Syntax
- Example 1: Dynamic Content Creation
- Example 2: Replacing HTML with JavaScript
- Example 3: Converting HTML to Normal Text
- Example 4: Embedding HTML in JavaScript
- Browser Compatibility
- Summary
Converting HTML to JavaScript can significantly improve your web application's performance and flexibility. By using JavaScript to manipulate HTML elements dynamically, you can create more interactive user experiences. In this tutorial, we will explore how to convert HTML to JavaScript, along with practical examples to illustrate different scenarios.
A Quick Code Example
Here's a simple example to get started:
In this snippet, we create a string of HTML and insert it into the document body using JavaScript.
Understanding the Syntax
When you convert HTML to JavaScript, you typically use methods like innerHTML
, createElement
, or template literals. Here’s a breakdown of the syntax:
- Parameters: The HTML string you want to convert.
- Return Values: The updated DOM elements that reflect the new HTML structure.
Basic Syntax Example
This code replaces the content of the element with the ID "myDiv" with the new paragraph.
Example 1: Dynamic Content Creation
Creating Elements with JavaScript
You can create HTML elements dynamically using JavaScript. This is useful for applications where content changes frequently.
1const newElement = document.createElement("div");
2newElement.innerHTML = "<p>This is a new paragraph.</p>";
3document.body.appendChild(newElement);
In this example, we create a new div
element and add it to the body of the document.
Example 2: Replacing HTML with JavaScript
Using JavaScript to Update Existing Content
You can replace existing HTML content with JavaScript easily.
This replaces the content of the element with the ID "content" with a new heading.
Example 3: Converting HTML to Normal Text
Removing HTML Tags
If you need to convert HTML to plain text, you can use the following method:
1const htmlString = "<p>This is <strong>bold</strong> text.</p>";
2const plainText = htmlString.replace(/<[^>]*>/g, "");
3console.log(plainText); // Output: This is bold text.
This code removes all HTML tags, leaving just the text.
Example 4: Embedding HTML in JavaScript
Including HTML in JavaScript Variables
You can store HTML in JavaScript variables for later use.
1const htmlContent = `<div><h1>Welcome</h1><p>This is a sample page.</p></div>`;
2document.body.innerHTML += htmlContent;
This adds a new section to the existing body content.
Browser Compatibility
Most modern browsers support these JavaScript methods, including Chrome, Firefox, Safari, and Edge. Ensure to test your code across different browsers to maintain consistent behavior.
Summary
In this tutorial, we explored how to convert HTML to JavaScript for better performance. We covered various methods to manipulate HTML dynamically, including creating elements, replacing content, and converting HTML to plain text. These techniques are essential for building interactive web applications. For more foundational knowledge, consider checking out our courses on HTML Fundamentals and JavaScript.
By understanding how to convert HTML to JavaScript, you can enhance user experience and optimize your web applications effectively. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
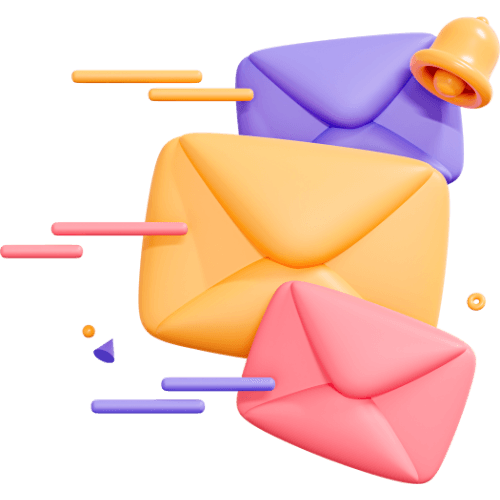
Related articles
9 Articles
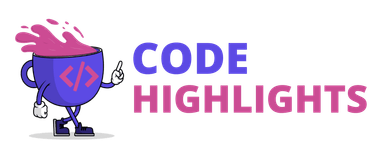
Copyright © Code Highlights 2025.