Ultimate Guide to Convert JavaScript to HTML: Boost Load Speed by 30%

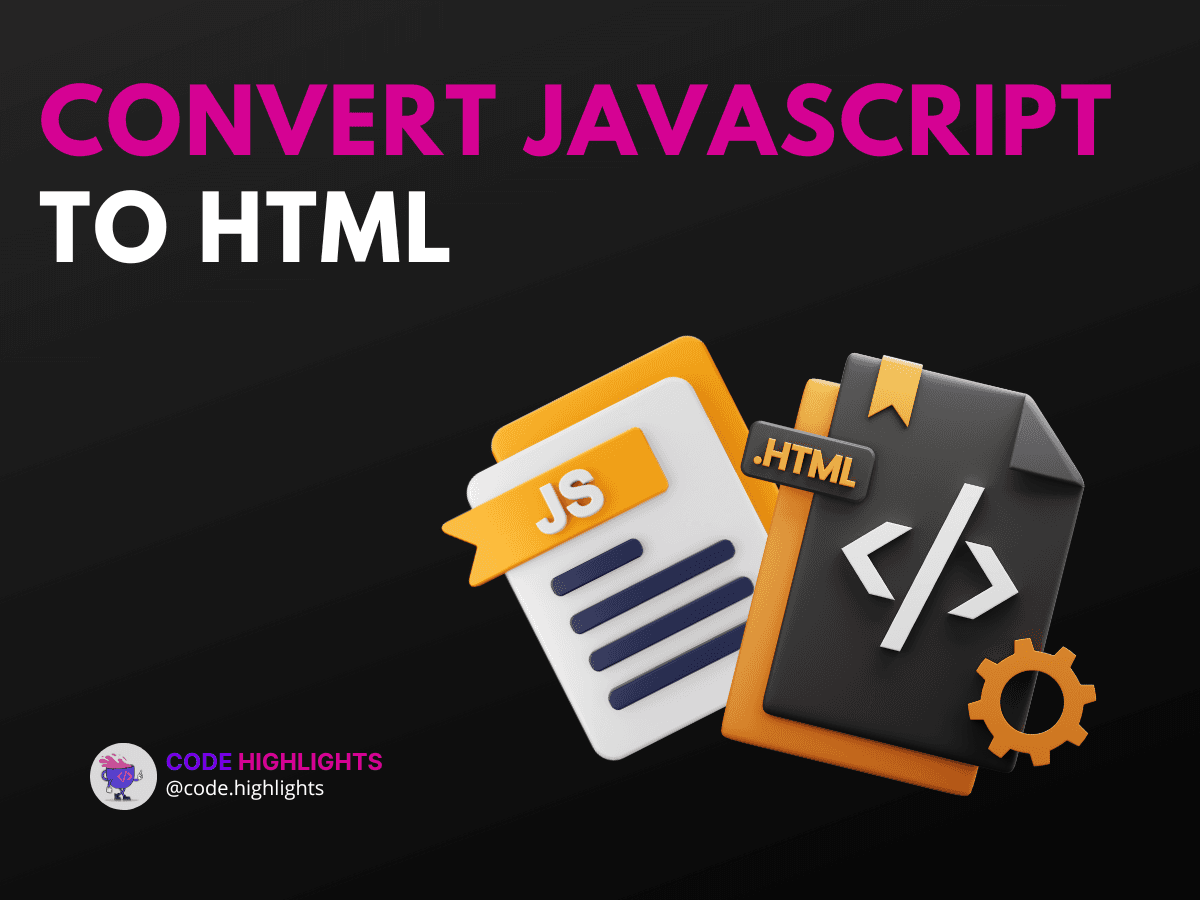
- Quick Code Example
- Understanding the Syntax
- Parameters:
- Return Values:
- Variations:
- Example 1: Linking External JavaScript
- Title: Linking an External Script
- Example 2: Inline JavaScript
- Title: Using Inline JavaScript
- Example 3: Creating a JavaScript Form in HTML
- Title: JavaScript Form Creation
- Compatibility with Major Web Browsers
- Summary
Are you looking to improve your website's performance? One effective way is to convert JavaScript to HTML. This process can help your site load faster, enhancing user experience. In this guide, we will explore how to integrate JavaScript into HTML seamlessly, along with practical examples and tips.
Quick Code Example
Before diving deeper, here's a simple example to show how JavaScript can be included in HTML:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Simple Example</title>
6 <script>
7 function greet() {
8 alert("Hello, World!");
9 }
10 </script>
11</head>
12<body>
13 <button onclick="greet()">Click Me!</button>
14</body>
15</html>
This code creates a button that, when clicked, displays a greeting message.
Understanding the Syntax
When you convert JavaScript to HTML, you typically use the <script>
tag. Here’s the basic syntax:
1<script src="path/to/your/javascript.js"></script>
Parameters:
src
: The path to your JavaScript file.
Return Values:
- The script runs and executes functions defined in it.
Variations:
You can place the <script>
tag in different parts of your HTML document:
- In the
<head>
section for scripts that need to load first. - At the end of the
<body>
for scripts that can run after the content loads.
Example 1: Linking External JavaScript
Title: Linking an External Script
To link an external JavaScript file, use the following code:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>External Script Example</title>
6 <script src="script.js"></script>
7</head>
8<body>
9 <h1>Welcome!</h1>
10</body>
11</html>
In this example, script.js
contains your JavaScript code. This keeps your HTML clean and organized.
Example 2: Inline JavaScript
Title: Using Inline JavaScript
You can also use JavaScript directly in your HTML like this:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Inline Script Example</title>
6</head>
7<body>
8 <h1>Inline JavaScript</h1>
9 <script>
10 document.write("This text is added with JavaScript!");
11 </script>
12</body>
13</html>
Here, the JavaScript code runs immediately and adds text to the page.
Example 3: Creating a JavaScript Form in HTML
Title: JavaScript Form Creation
You can create a form that uses JavaScript to validate input before submission:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Form Example</title>
6 <script>
7 function validateForm() {
8 const name = document.forms["myForm"]["name"].value;
9 if (name === "") {
10 alert("Name must be filled out");
11 return false;
12 }
13 }
14 </script>
15</head>
16<body>
17 <form name="myForm" onsubmit="return validateForm()">
18 Name: <input type="text" name="name"><br>
19 <input type="submit" value="Submit">
20 </form>
21</body>
22</html>
This form checks if the name field is empty before submitting.
Compatibility with Major Web Browsers
JavaScript is widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. However, it's essential to test your code to ensure it works consistently across different platforms.
Summary
In this guide, we explored how to convert JavaScript to HTML effectively. We learned how to link external scripts, use inline JavaScript, and create forms that validate user input. By implementing these techniques, you can enhance your website's performance and user experience.
For more in-depth learning, consider checking out our courses on JavaScript, HTML Fundamentals, and CSS Introduction.
By understanding how to integrate JavaScript into your HTML, you can create more dynamic and interactive web pages. Happy coding!
For further reading, you can refer to resources from Mozilla Developer Network or W3Schools JavaScript Tutorial.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
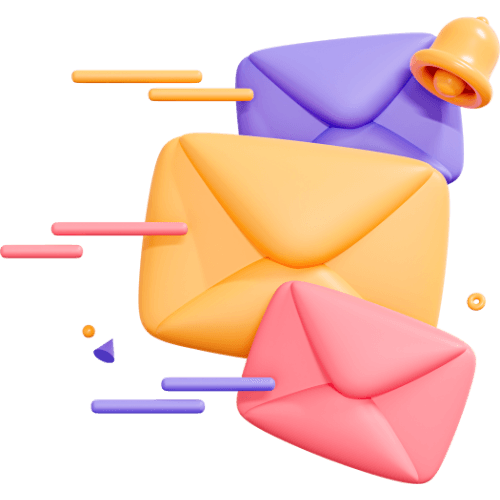
Related articles
9 Articles
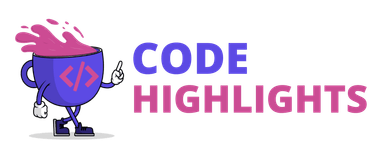
Copyright © Code Highlights 2025.