How to Convert String to Array in JavaScript Effortlessly

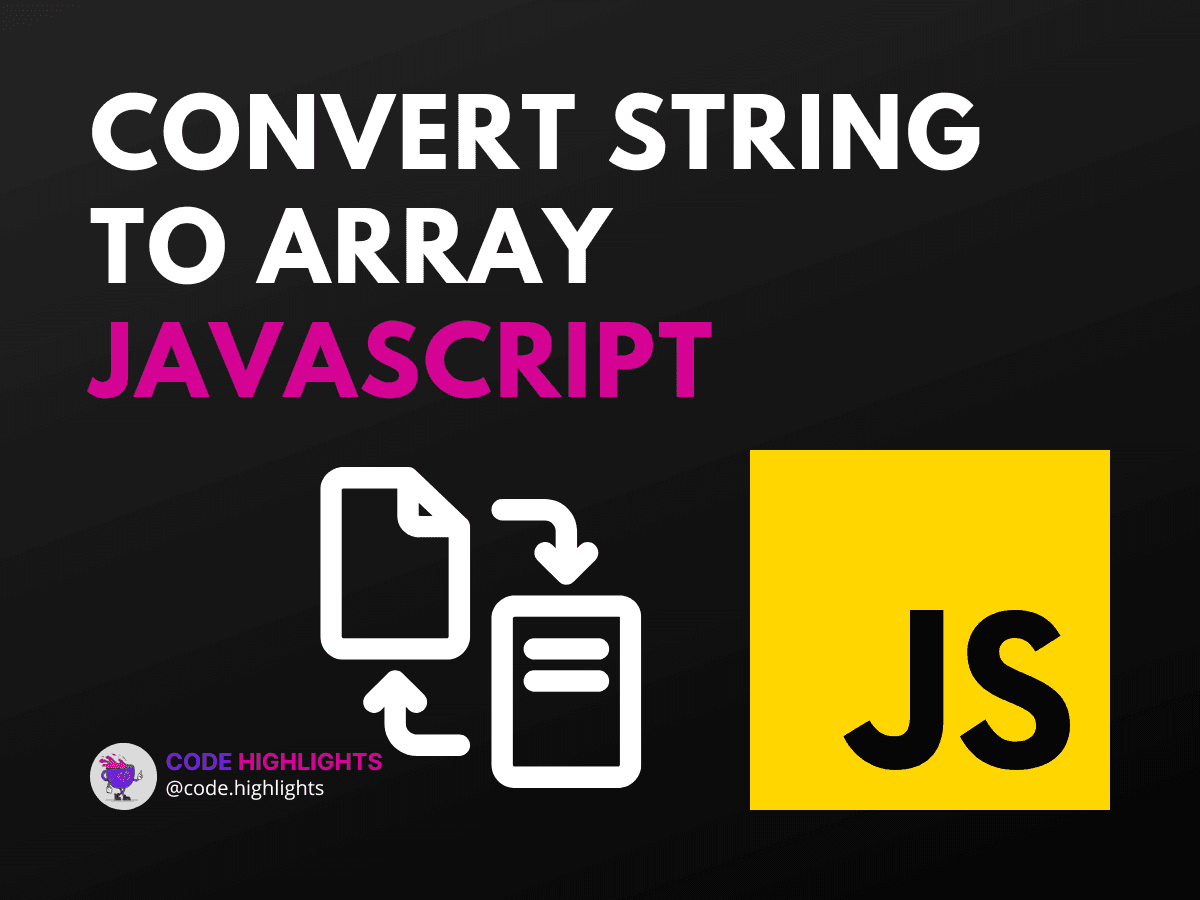
- Understanding String-to-Array Conversion
- The Split Method
- Converting with No Separator
- Special Cases: Handling Whitespace and Empty Strings
- Advanced Splitting: Using Regular Expressions
- Linking to Further Learning
- External Resources for Deeper Understanding
- Conclusion
JavaScript is an incredibly versatile language, and one of its many useful features is the ability to manipulate strings and arrays. Sometimes, you may need to convert a string into an array to perform certain operations. In this tutorial, we'll dive into the simple yet powerful methods to convert string to array in JavaScript
. Whether you're a beginner or an experienced developer, understanding this process is essential for handling text data effectively.
Let's kick things off with a quick example:
This snippet shows how a string is turned into an array of characters. Now, let's explore the steps in more detail.
Understanding String-to-Array Conversion
Converting a string to an array may sound complex, but JavaScript provides straightforward methods to achieve this. You might wonder, "How do you convert a string to an array in JavaScript?" or "How to split a string into an array of characters in JavaScript?" The answer lies in the .split()
method, which we will cover extensively.
The Split Method
The .split()
method is your go-to tool for breaking down a string into an array. It takes a separator as an argument and slices the string wherever it finds this separator. If you don't provide any separator, the entire string becomes a single-element array. Here's how it works:
1let text = "apple, banana, cherry";
2let fruitsArray = text.split(", ");
3console.log(fruitsArray); // Output: ["apple", "banana", "cherry"]
In the above code, we used a comma followed by a space as the separator.
Converting with No Separator
Sometimes, you might want to convert into an array in JavaScript
where each character is an individual element. This is particularly useful when you need to manipulate or analyze each character separately:
1let singleChars = "abc";
2let charsArray = singleChars.split("");
3console.log(charsArray); // Output: ["a", "b", "c"]
Special Cases: Handling Whitespace and Empty Strings
What if your string contains whitespace or is empty? The .split()
method can handle these scenarios gracefully:
1let stringWithSpaces = " ";
2let emptyArray = stringWithSpaces.split(" ");
3console.log(emptyArray); // Output: ["", "", "", ""]
4
5let emptyString = "";
6let emptyArrayFromString = emptyString.split("");
7console.log(emptyArrayFromString); // Output: []
Advanced Splitting: Using Regular Expressions
For more complex splitting logic, regular expressions come into play. They allow you to specify patterns for the separator:
1let complexString = "One1Two2Three3";
2let numbersRemovedArray = complexString.split(/\d/);
3console.log(numbersRemovedArray); // Output: ["One", "Two", "Three"]
This regular expression (/\d/
) matches any digit, effectively removing numbers from the string.
Linking to Further Learning
If you're new to JavaScript or need to brush up on your skills, consider checking out this JavaScript course. For those of you looking to understand the foundational web technologies better, these courses on HTML fundamentals and CSS introduction are invaluable. And if you're just starting your journey into web development, don't miss this comprehensive introduction to web development.
External Resources for Deeper Understanding
To enrich your learning, here are some external resources:
- Mozilla Developer Network (MDN) on the split() method
- W3Schools JavaScript String Methods
- JavaScript.info on strings
Conclusion
Converting strings to arrays in JavaScript is a fundamental skill that opens up numerous possibilities for string manipulation and data processing. By using the .split()
method, you can tailor the conversion process to fit your specific needs, whether it's splitting by a delimiter or breaking down each character. Remember, practice is key to mastering this technique, so experiment with different strings and separators to see the method in action.
With the knowledge you've gained today, you're well-equipped to handle various challenges involving string and array manipulations in JavaScript. Keep coding and exploring, and you'll continue to uncover the power and flexibility of JavaScript!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
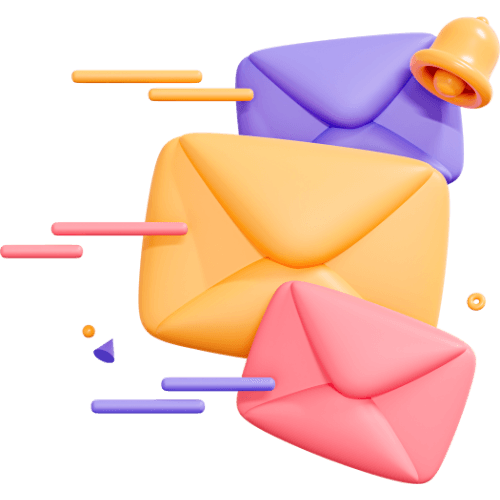
Related articles
9 Articles
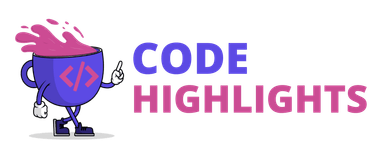
Copyright © Code Highlights 2025.