Converting strings to numbers with vanilla JavaScript

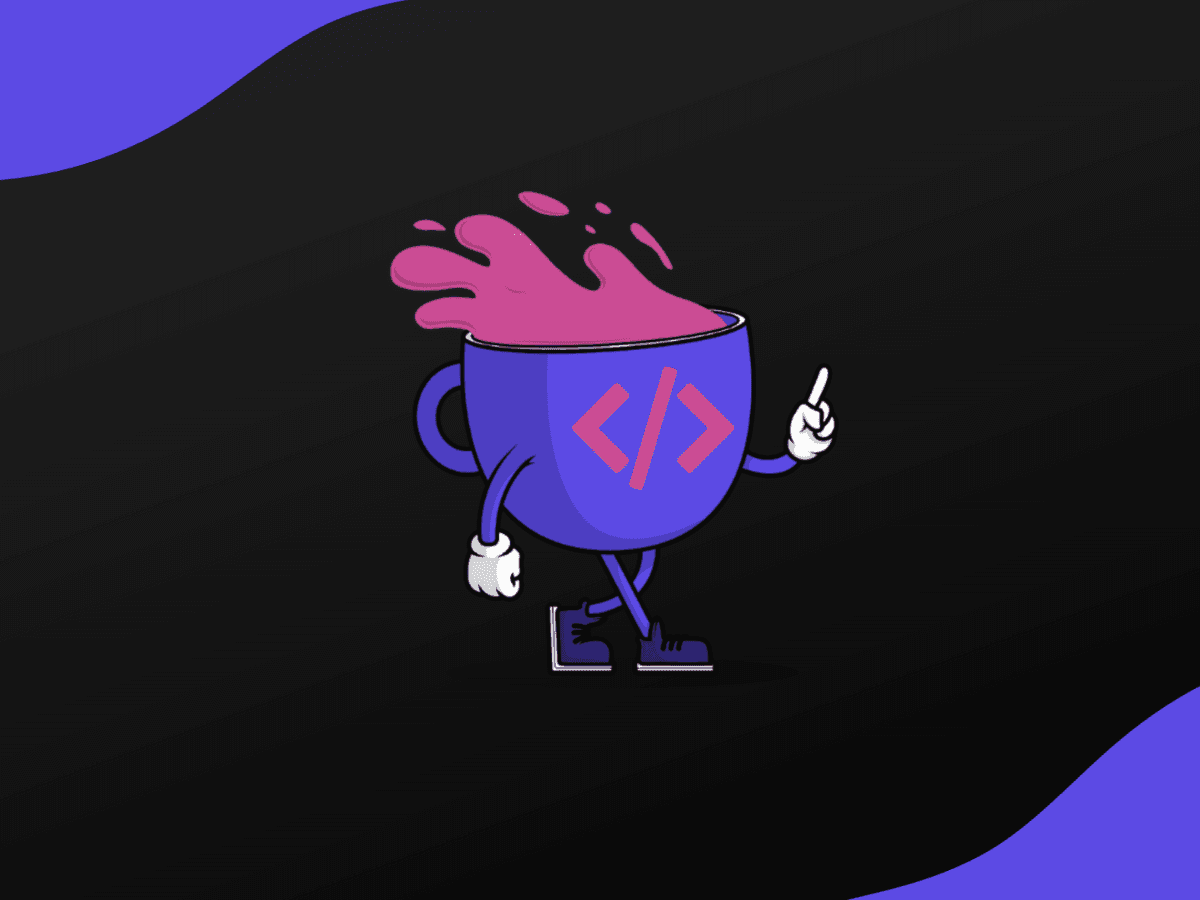
- Using parseInt()
- Using parseFloat()
- Using Number()
- Browser Compatibility
In JavaScript, it is common to find scenarios where we need to convert a string into a number.
Whether it's user input or data from an API, strings often need to be converted into numbers for mathematical operations or comparisons.
Today, we will explore three different ways to convert a string into a number using vanilla JavaScript.
Using parseInt()
The parseInt()
function is a popular method used to convert a string into an integer. It accepts two arguments; the string to convert and the radix, which should always be 10
for our purposes.
Here, parseInt()
ignores the 'px' and returns the integer 42. You can learn more about parseInt()
in our JavaScript course.
Using parseFloat()
The parseFloat()
function is used to convert a string into a point number (a number with decimal points). It's useful when dealing with values that aren't whole numbers.
parseFloat()
disregards the 'random' text and returns the floating-point number 3.14.
Using Number()
The Number()
function is another method to convert a string into a number. It can return an integer or a point number based on the input. However, if the string contains non-numeric characters, it returns NaN
(Not a Number).
1Number("123"); // returns 123
2Number("12.3"); // returns 12.3
3Number("3.14random"); // returns NaN
4Number("42px"); // returns NaN
If you're unsure about the format of the number, use parseInt()
or parseFloat()
. If you want the string to fail with NaN
if it has non-numerical characters, Number()
is a better choice.
Browser Compatibility
All these methods work in all modern browsers, including IE6 and up. To learn more about JavaScript, check out our JavaScript course. If you're new to web development, start with our introduction to web development course.
For further reading, Mozilla's JavaScript reference and W3School's JavaScript tutorial offer comprehensive guides on JavaScript's built-in functions.
In summary, converting a string to a number in JavaScript can be done using parseInt()
, parseFloat()
, or Number()
. The fastest way is typically using Number()
, but parseInt()
and parseFloat()
are safer choices when the format of the number is known.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
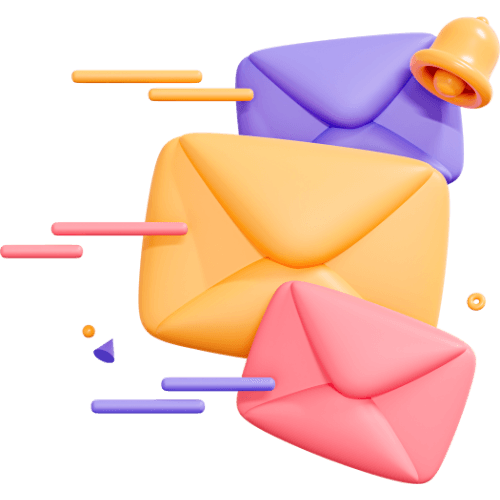
Related articles
9 Articles
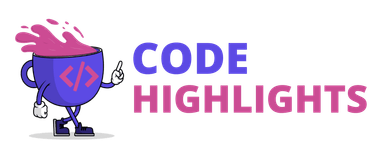
Copyright © Code Highlights 2025.