7 Easy Steps to Create Button Using JavaScript

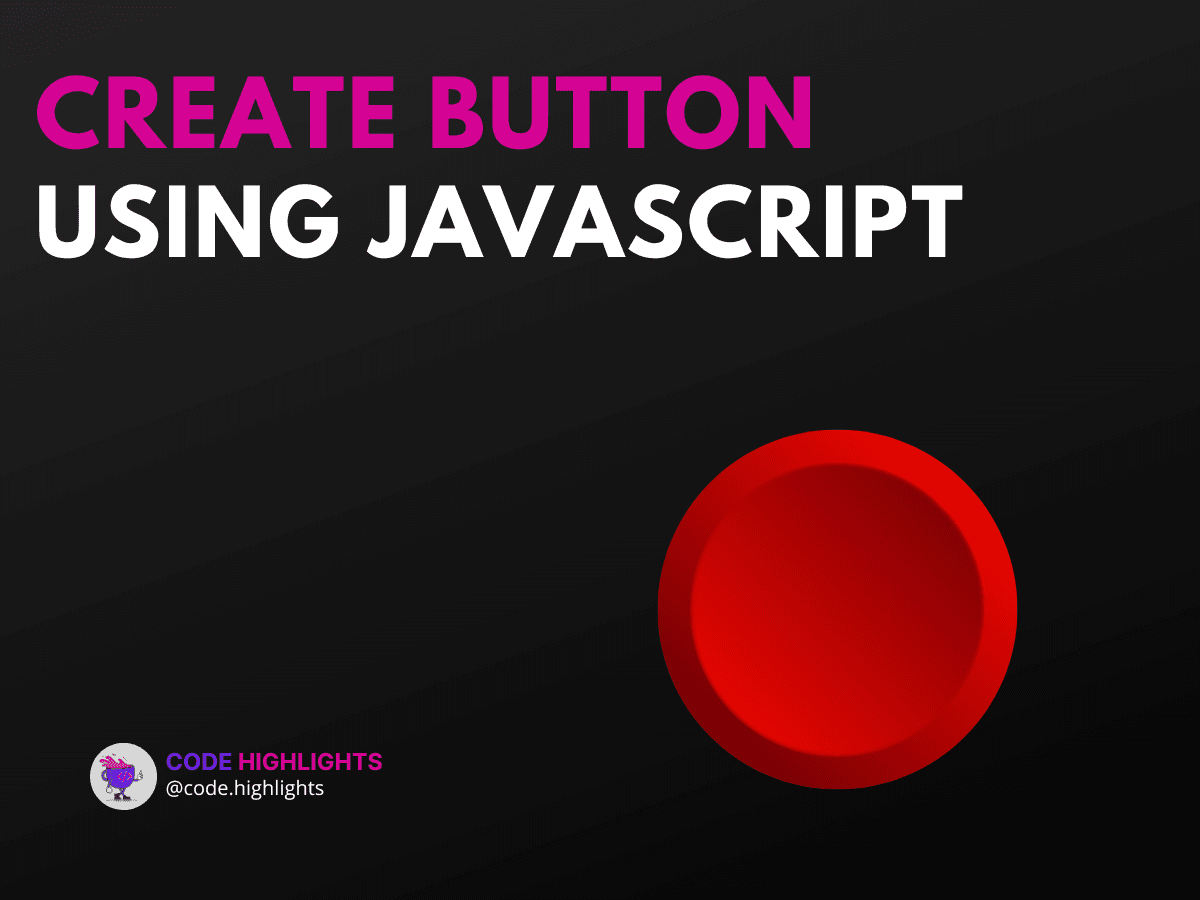
- Quick Code Example
- Step 1: Understanding the Button Element
- Parameters:
- Return Values:
- Step 2: Creating a Button in JavaScript
- Step 3: Adding Click Events
- Step 4: Styling Your Button
- Step 5: Creating a Link Button
- Step 6: Generating Multiple Buttons
- Step 7: Compatibility with Browsers
- Summary
Creating a button using JavaScript is a fun and straightforward process. Buttons are essential elements in web development, allowing users to interact with your webpage. In this tutorial, we will walk through seven easy steps to create a button using JavaScript. By the end, you will have a solid understanding of how to generate buttons and respond to user actions.
Quick Code Example
Here's a quick example to get you started. This code creates a button that changes the text when clicked:
1<!DOCTYPE html>
2<html lang="en">
3<head>
4 <meta charset="UTF-8">
5 <title>Button Example</title>
6</head>
7<body>
8 <button id="myButton">Click Me!</button>
9
10 <script>
11 document.getElementById("myButton").onclick = function() {
12 this.innerHTML = "You clicked me!";
13 };
14 </script>
15</body>
16</html>
Step 1: Understanding the Button Element
To create a button using JavaScript, we first need to know about the <button>
element in HTML. The basic syntax looks like this:
1<button type="button">Button Text</button>
Parameters:
type
: Defines the button's behavior (e.g., "button", "submit", "reset").Button Text
: The visible text on the button.
Return Values:
The button itself can trigger various events, such as clicks or hover actions.
Step 2: Creating a Button in JavaScript
Now, let’s create a button using JavaScript. You can use the document.createElement
method to make a button dynamically:
1let button = document.createElement("button");
2button.innerHTML = "New Button";
3document.body.appendChild(button);
This code snippet creates a new button and adds it to the webpage.
Step 3: Adding Click Events
To make your button interactive, you can add click events. Here’s how you can do that:
This code will show an alert when the button is clicked.
Step 4: Styling Your Button
You can also style your button using CSS. Here’s an example of how to change its appearance:
1button.style.backgroundColor = "blue";
2button.style.color = "white";
3button.style.padding = "10px 20px";
This will make your button look more appealing.
Step 5: Creating a Link Button
If you want your button to act like a link, you can do this by using the window.location
property:
This code will redirect the user to a new webpage when the button is clicked.
Step 6: Generating Multiple Buttons
You might want to create multiple buttons. You can do this with a loop:
1for (let i = 0; i < 3; i++) {
2 let btn = document.createElement("button");
3 btn.innerHTML = "Button " + (i + 1);
4 document.body.appendChild(btn);
5}
This will create three buttons with different texts.
Step 7: Compatibility with Browsers
JavaScript buttons are widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. You can confidently use JavaScript to create buttons for any modern web application.
Summary
In this tutorial, we covered how to create a button using JavaScript in seven easy steps. We learned about button elements, adding click events, styling, creating link buttons, and generating multiple buttons. Buttons are crucial for user interaction on websites, and knowing how to implement them enhances your web development skills. If you're interested in learning more about web development, check out our introduction to web development course or dive into HTML fundamentals.
Feel free to explore more about JavaScript in our learn JavaScript course, or check out our CSS introduction for styling tips!
Now you’re ready to create buttons that can improve user engagement on your website!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
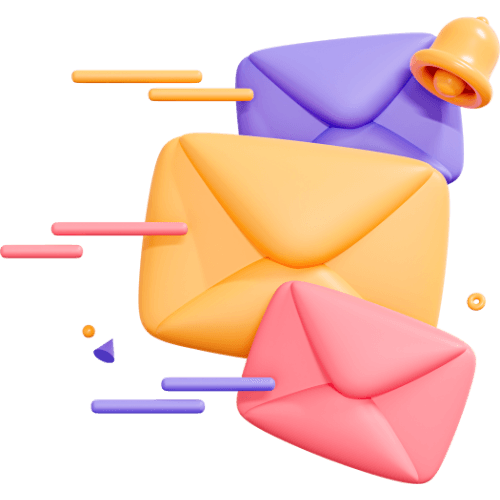
Related articles
9 Articles
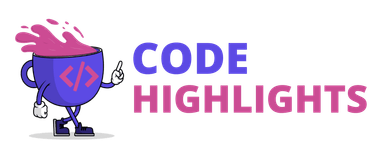
Copyright © Code Highlights 2025.