How to Use Curl in JavaScript for Effective API Requests

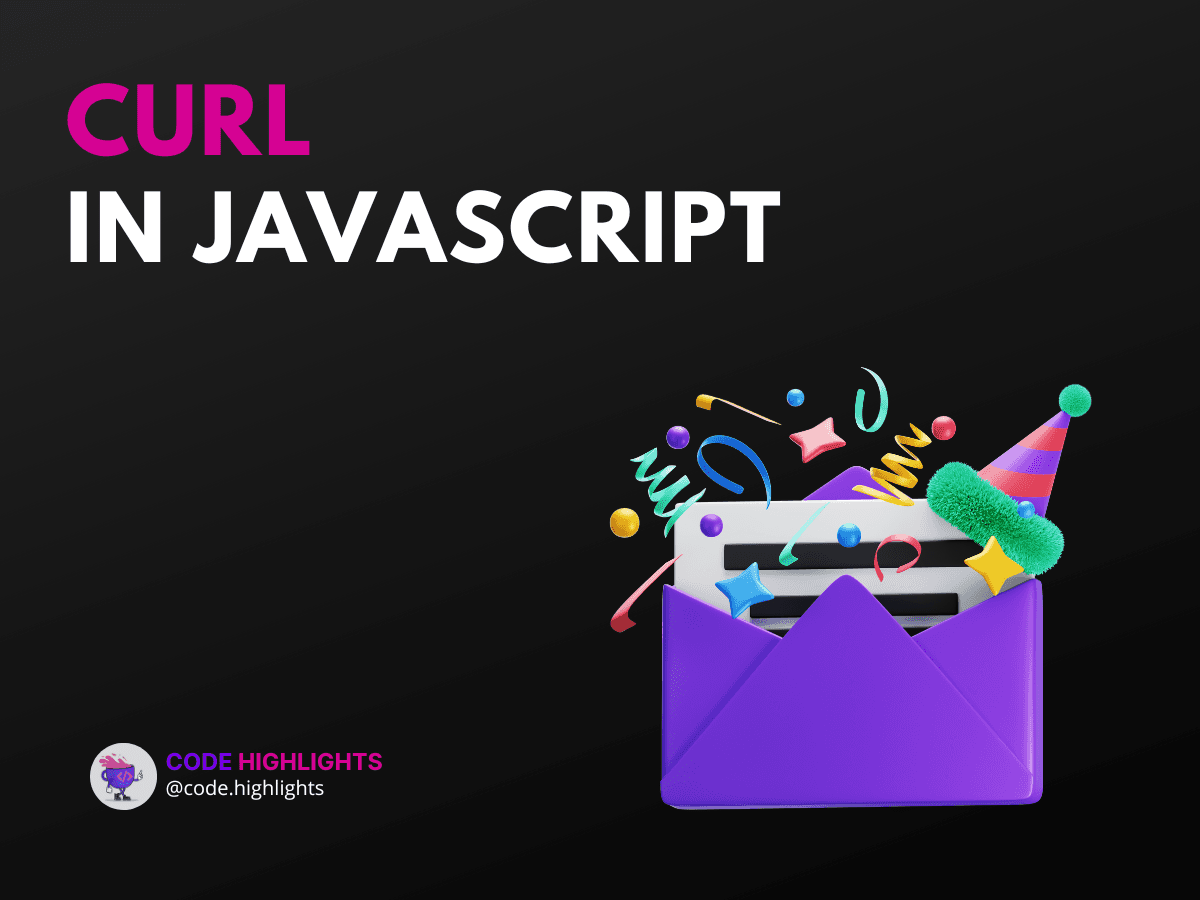
- Understanding the Syntax of cURL in JavaScript
- Parameters:
- Return Value:
- Example of a POST Request
- Unique Use Cases for cURL in JavaScript
- 1. Handling Different HTTP Methods
- 2. Adding Headers
- 3. Using Async/Await for Clarity
- Browser Compatibility
- Conclusion
When working with web applications, you often need to communicate with servers to send or retrieve data. This is where cURL comes into play. Although cURL is primarily a command-line tool, its concepts can be applied in JavaScript to make API requests. Understanding how to use cURL in JavaScript can help you interact with various web services effectively.
Here's a quick example to get started:
1fetch('https://api.example.com/data')
2 .then(response => response.json())
3 .then(data => console.log(data))
4 .catch(error => console.error('Error:', error));
In this snippet, we use the fetch
function to make a GET request to an API endpoint. The response is then converted to JSON format, and any errors are caught and logged.
Understanding the Syntax of cURL in JavaScript
In JavaScript, the equivalent of cURL for making HTTP requests is typically the fetch
API or libraries like Axios. The basic syntax for using fetch
looks like this:
Parameters:
- url: A string representing the URL to which the request is sent.
- options: An optional object that includes method type (GET, POST, etc.), headers, body, and other configurations.
Return Value:
The fetch
function returns a Promise that resolves to the Response object representing the response to the request.
Example of a POST Request
Here’s how to send a POST request using fetch
:
1fetch('https://api.example.com/data', {
2 method: 'POST',
3 headers: {
4 'Content-Type': 'application/json'
5 },
6 body: JSON.stringify({ name: 'John', age: 30 })
7})
8.then(response => response.json())
9.then(data => console.log(data))
10.catch(error => console.error('Error:', error));
In this example, we're sending JSON data to the server. The body
contains the data we want to send.
Unique Use Cases for cURL in JavaScript
1. Handling Different HTTP Methods
Using different HTTP methods is crucial when interacting with APIs. Here’s an example of a DELETE request:
1fetch('https://api.example.com/data/1', {
2 method: 'DELETE'
3})
4.then(response => {
5 if (response.ok) {
6 console.log('Data deleted successfully');
7 } else {
8 console.error('Error deleting data');
9 }
10})
11.catch(error => console.error('Error:', error));
This snippet demonstrates how to delete a resource from the server.
2. Adding Headers
Sometimes, you may need to include authentication tokens or other headers. Here's how to do it:
1fetch('https://api.example.com/protected-data', {
2 method: 'GET',
3 headers: {
4 'Authorization': 'Bearer YOUR_TOKEN_HERE'
5 }
6})
7.then(response => response.json())
8.then(data => console.log(data))
9.catch(error => console.error('Error:', error));
In this case, we pass an authorization token in the headers to access protected resources.
3. Using Async/Await for Clarity
Using async/await can make your code cleaner. Here’s an example:
1async function fetchData() {
2 try {
3 const response = await fetch('https://api.example.com/data');
4 const data = await response.json();
5 console.log(data);
6 } catch (error) {
7 console.error('Error:', error);
8 }
9}
10
11fetchData();
This approach allows you to write asynchronous code that looks synchronous, improving readability.
Browser Compatibility
The fetch
API is widely supported in modern browsers, including Chrome, Firefox, and Edge. However, older browsers like Internet Explorer might not support it. For broader compatibility, consider using a library like Axios, which works across more environments.
Conclusion
In this tutorial, we explored how to use cURL concepts in JavaScript for effective API requests. We discussed the syntax, parameters, and return values of the fetch
API. Additionally, we provided several code examples demonstrating different use cases, such as handling various HTTP methods and adding headers.
Understanding how to implement cURL in JavaScript is essential for any web developer. It allows you to interact with APIs seamlessly, making your applications more dynamic and responsive. If you're interested in learning more about web development, check out our HTML Fundamentals Course and Introduction to Web Development.
For further reading, you can explore MDN Web Docs on Fetch, Axios Documentation, and RESTful API Design.
By mastering these techniques, you'll be well-equipped to handle API interactions in your projects!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
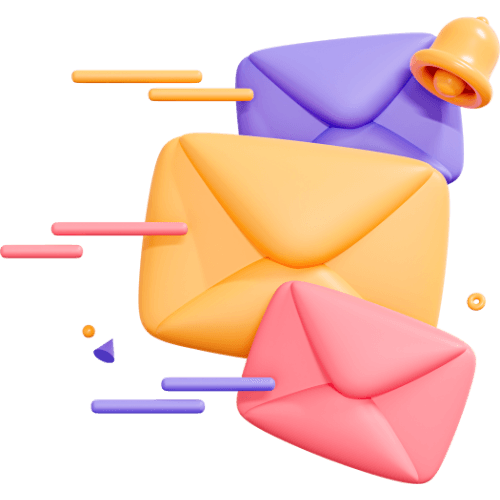
Related articles
9 Articles
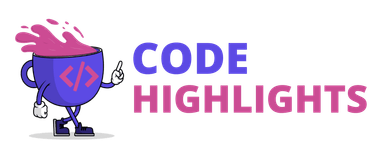
Copyright © Code Highlights 2025.