7 Innovative Deck of Cards JavaScript Techniques for 2024

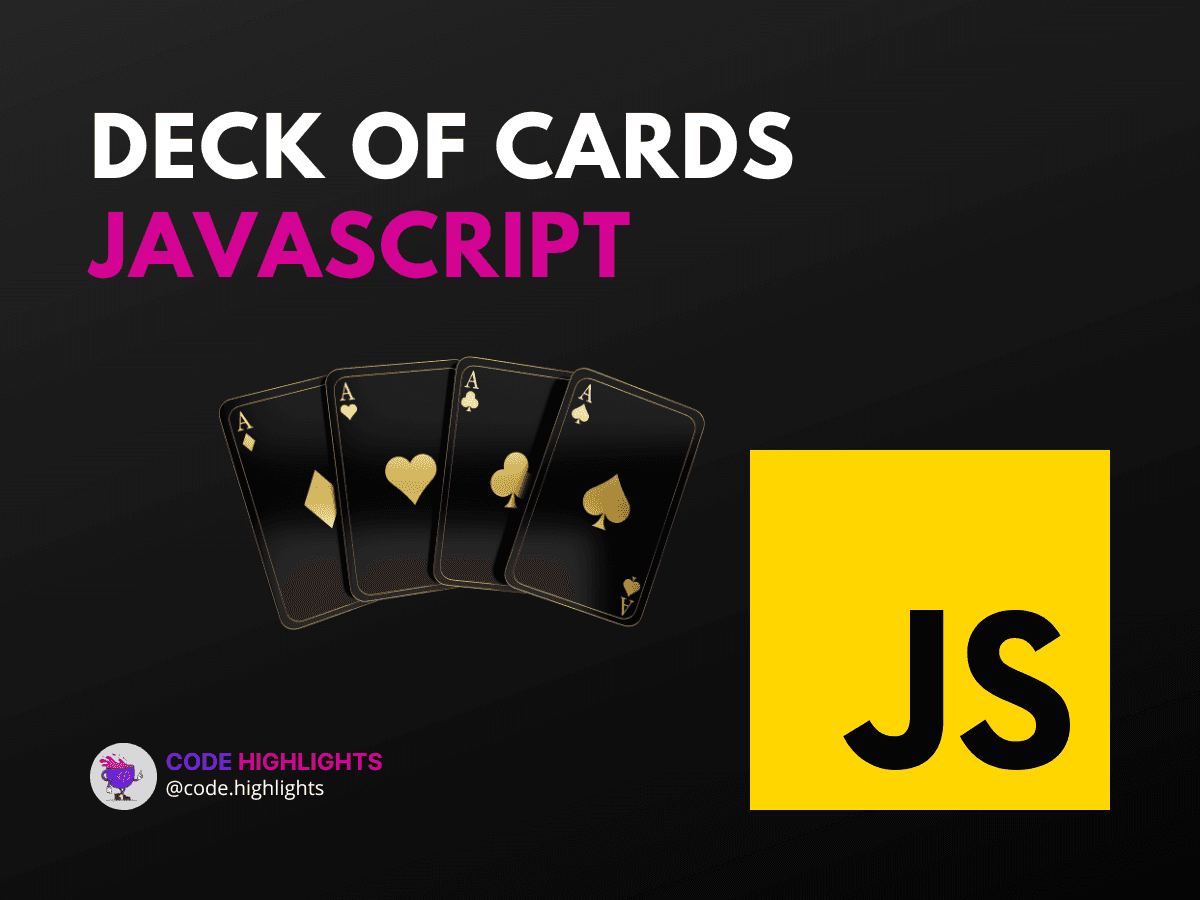
- Technique 1: Creating a Deck of Cards
- Technique 2: Shuffling the Deck
- Technique 3: Drawing Cards
- Technique 4: Sorting the Deck
- Technique 5: Filtering Cards
- Technique 6: Displaying the Deck
- Technique 7: Adding Gameplay Logic
Welcome to the fascinating world of coding with a deck of cards in JavaScript! Whether you're a seasoned developer or just starting out, mastering these innovative techniques will elevate your programming game in 2024. In this tutorial, we'll explore how to harness the power of JavaScript to create, shuffle, and manipulate a virtual deck of cards. So, let's deal you in on some coding fun!
Before we dive into the nitty-gritty, here's a sneak peek at JavaScript in action:
1const deck = [];
2const suits = ['Hearts', 'Diamonds', 'Clubs', 'Spades'];
3const values = ['Ace', '2', '3', '4', '5', '6', '7', '8', '9', '10', 'Jack', 'Queen', 'King'];
4
5// Initialize the deck
6suits.forEach(suit => {
7 values.forEach(value => {
8 deck.push({ value, suit });
9 });
10});
This snippet initializes a standard 52-card deck. Exciting, isn't it? Now, let's shuffle up and deal with our seven innovative techniques.
Technique 1: Creating a Deck of Cards
Creating a deck of cards is the foundation of any card game. Here's how to do it in JavaScript:
1function createDeck() {
2 return suits.flatMap(suit => values.map(value => ({ value, suit })));
3}
4const myDeck = createDeck();
This function uses flatMap
and map
to generate the deck. You've just created a new deck of cards with just a few lines of code!
Technique 2: Shuffling the Deck
A shuffled deck is crucial for any card game. Let's use the Fisher-Yates algorithm:
1function shuffleDeck(deck) {
2 for (let i = deck.length - 1; i > 0; i--) {
3 const j = Math.floor(Math.random() * (i + 1));
4 [deck[i], deck[j]] = [deck[j], deck[i]];
5 }
6 return deck;
7}
8shuffleDeck(myDeck);
This function shuffles the cards in place, ensuring a random order every time.
Technique 3: Drawing Cards
Drawing cards from the deck is a common operation. Here's a simple way to do it:
The pop
method removes the last element from the deck and returns it as the drawn card.
Technique 4: Sorting the Deck
Sometimes, you might want to sort the deck by suit or value. Here's a technique using sort
:
1function sortDeck(deck) {
2 return deck.sort((cardA, cardB) => {
3 if (cardA.suit === cardB.suit) {
4 return values.indexOf(cardA.value) - values.indexOf(cardB.value);
5 }
6 return suits.indexOf(cardA.suit) - suits.indexOf(cardB.suit);
7 });
8}
9sortDeck(myDeck);
This function sorts the deck first by suit, then by value within each suit.
Technique 5: Filtering Cards
To find specific cards, use the filter
method:
1function filterCards(deck, suit) {
2 return deck.filter(card => card.suit === suit);
3}
4const spades = filterCards(myDeck, 'Spades');
This function returns all cards of the specified suit.
Technique 6: Displaying the Deck
To display the deck on a webpage, you could use HTML and CSS. Here's a quick example:
1function displayDeck(deck) {
2 const deckElement = document.createElement('div');
3 deck.forEach(card => {
4 const cardElement = document.createElement('div');
5 cardElement.className = 'card';
6 cardElement.innerText = `${card.value} of ${card.suit}`;
7 deckElement.appendChild(cardElement);
8 });
9 document.body.appendChild(deckElement);
10}
11displayDeck(myDeck);
For more on HTML and CSS, check out this HTML fundamentals course and this introduction to CSS.
Technique 7: Adding Gameplay Logic
Finally, to create a game, you need to add logic. This involves setting conditions for winning, losing, and scoring. For an introduction to web development, including game logic, visit Introduction to Web Development.
We've just gone through seven innovative ways to work with a deck of cards in JavaScript. If you're interested in diving deeper into JavaScript, consider this JavaScript course.
Remember, practice makes perfect. Keep experimenting with these techniques, and soon you'll be able to build your own engaging card games. Happy coding!
This tutorial is just the beginning of what you can achieve with JavaScript. For further reading and to stay current with best practices, refer to reputable sources such as Mozilla Developer Network (MDN), W3Schools, and Stack Overflow. These resources offer a wealth of information for developers of all skill levels.
Remember to keep your code clean, comment often, and always be learning. The world of JavaScript is vast and ever-evolving—there's always something new to discover!
Happy shuffling!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
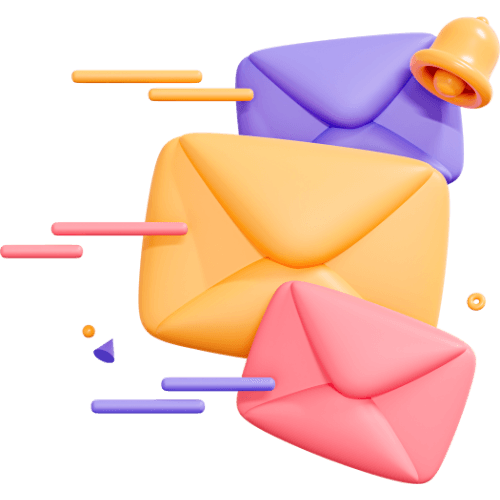
Related articles
9 Articles
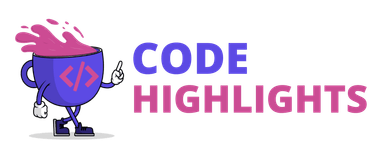
Copyright © Code Highlights 2024.