3 Quick Methods to Disable Button in JavaScript Today

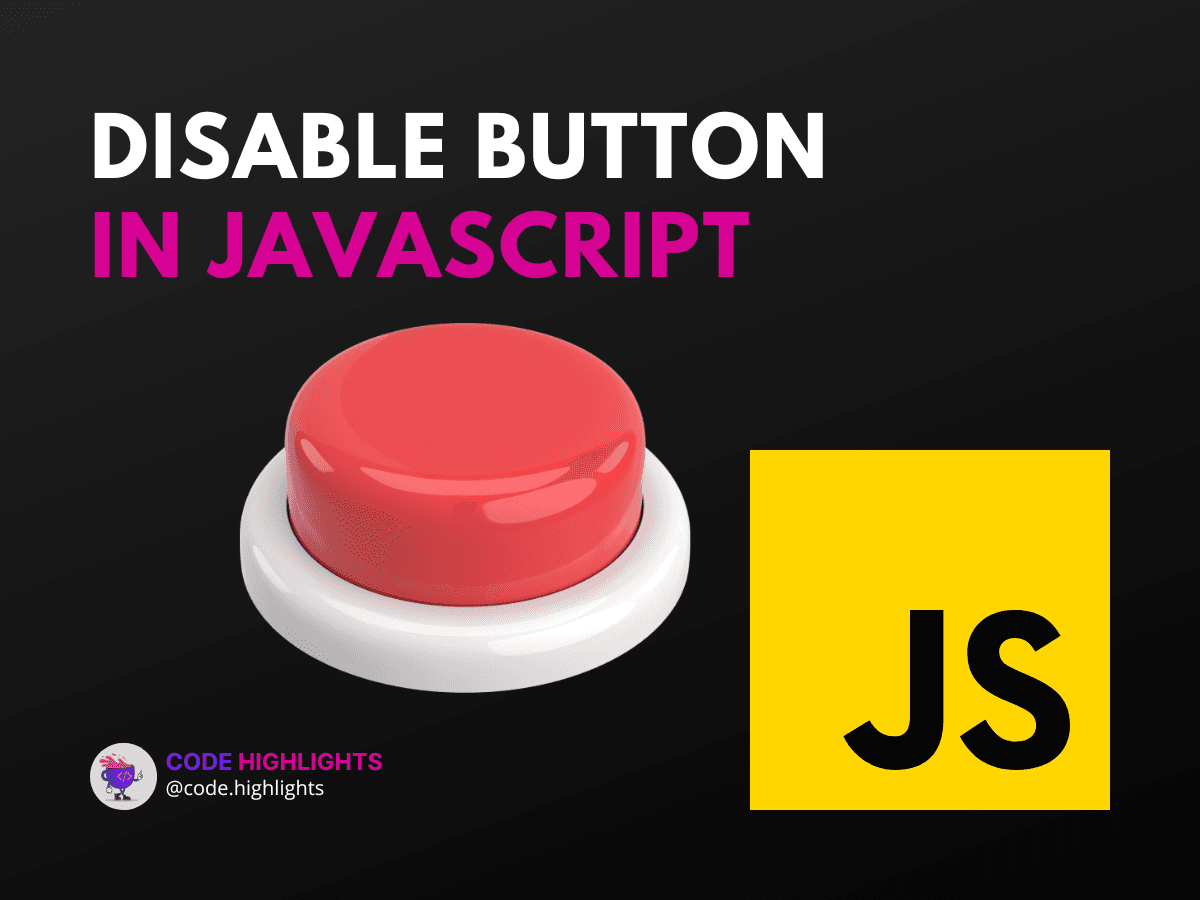
- Method 1: Using the disabled Attribute
- Method 2: Disabling Button Click Event
- Method 3: Disabling Buttons within a Table
- Conclusion
JavaScript is a powerful tool in the hands of web developers, allowing for dynamic user interactions on web pages. One common task is controlling the state of buttons. Imagine a scenario where you need to prevent users from clicking a button after they've submitted a form or while an operation is in progress. That's where disabling buttons comes into play. In this tutorial, we'll explore how to effectively disable a button in JavaScript, ensuring a smoother user experience.
Let's start with a basic example of a button in HTML:
1<button id="myButton">Click Me!</button>
Now, let's jump into the methods to disable this button using JavaScript.
Method 1: Using the disabled
Attribute
The simplest way to disable a button is by setting the disabled
attribute directly via JavaScript.
This line of code will make the button unclickable, answering your question, "How do I make a button disabled?" This method is straightforward and commonly used across various applications.
Learn JavaScript with our comprehensive course to understand more about manipulating DOM elements like buttons.
Method 2: Disabling Button Click Event
Sometimes, you might want to disable only the click event of a button without changing its appearance. Here's how you can do it:
1document.getElementById('myButton').addEventListener('click', function(event){
2 event.preventDefault();
3});
By using event.preventDefault()
, you ensure that the button's default action is not taken when it is clicked, effectively answering "How do you disable click in JavaScript?"
Method 3: Disabling Buttons within a Table
Disabling a button within a table requires a bit more specificity. Suppose you have the following HTML structure:
1<table>
2 <tr>
3 <td><button class="table-button">Table Button 1</button></td>
4 </tr>
5 <tr>
6 <td><button class="table-button">Table Button 2</button></td>
7 </tr>
8</table>
To disable a button in a table in JavaScript, you could use:
1var tableButtons = document.querySelectorAll('.table-button');
2for(var i = 0; i < tableButtons.length; i++) {
3 tableButtons[i].disabled = true;
4}
This script will disable all buttons with the class .table-button
, ensuring they are unclickable within your table.
Brush up on your HTML skills with our HTML Fundamentals Course to better understand how button elements work within different contexts.
Conclusion
With these three methods, you can control the behavior of buttons on your web pages, enhancing user experience by preventing unwanted actions. Remember, the key is to choose the method that best fits the context of your application.
For further reading, check out these articles:
- MDN Web Docs on the Button element
- W3Schools JavaScript Tutorial
- JavaScript.info - An Introduction to JavaScript
Expand your web development knowledge by exploring our Introduction to Web Development and Learn CSS Introduction courses.
By now, you should feel confident in your ability to disable a button in JavaScript. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
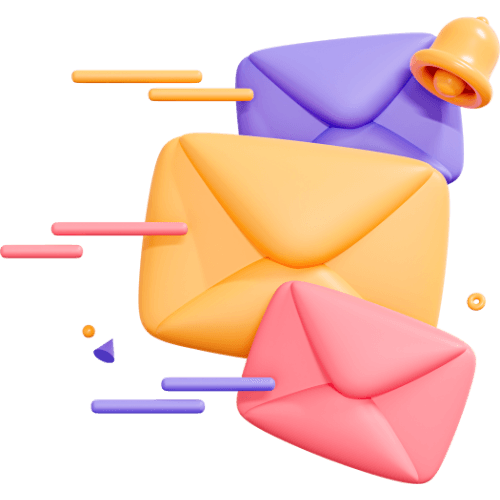
Related articles
9 Articles
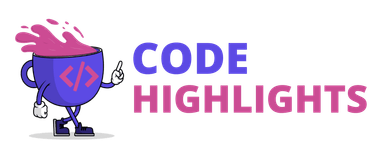
Copyright © Code Highlights 2024.