JavaScript Events and Event Handlers

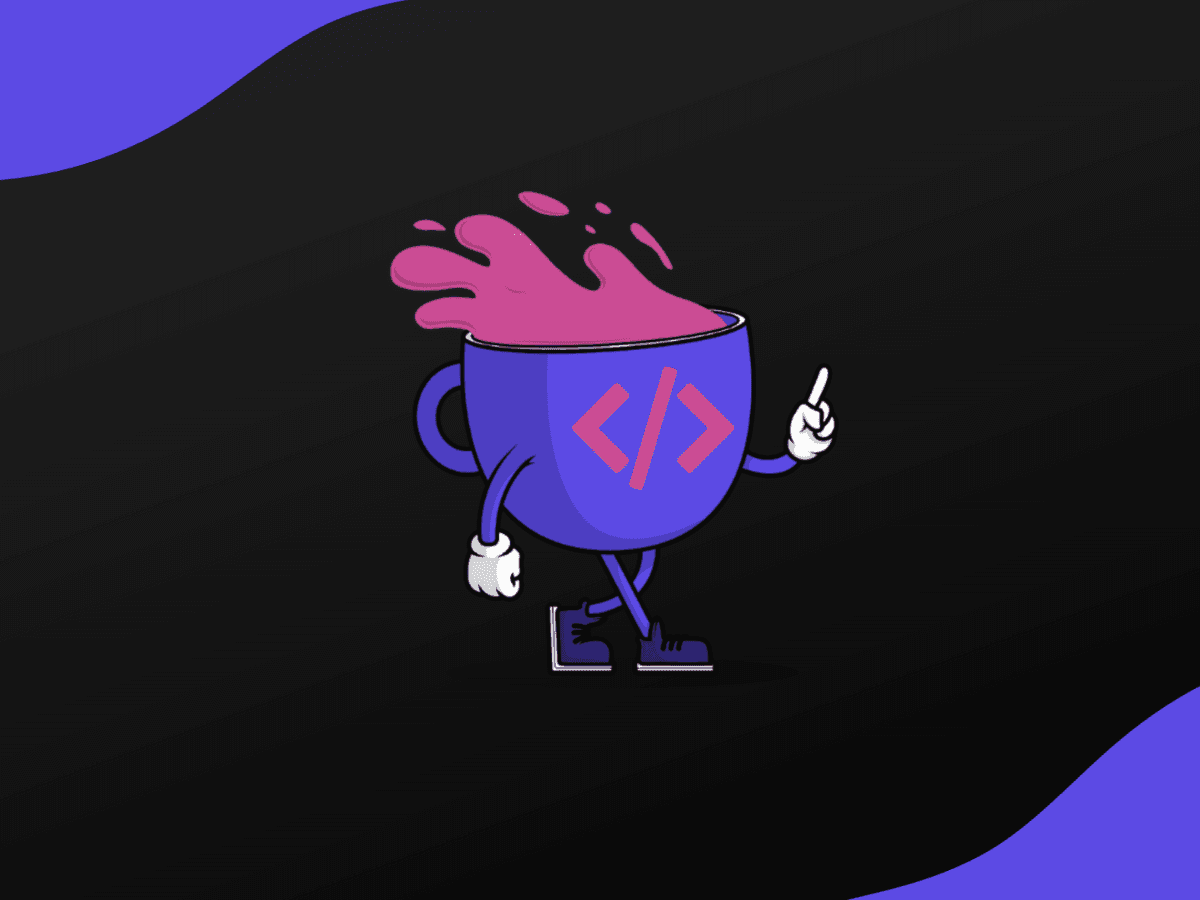
- Understanding JavaScript Events
- Types of JavaScript Events
- Using Event Handlers
- Best Practices for Using Events and Event Handlers
- Conclusion
JavaScript is a popular programming language that is used to build interactive and dynamic web applications. One of the key features of JavaScript is its ability to respond to user events such as clicks, scrolls and keyboard inputs. In this article, we will explore how JavaScript events work and how you can use event handlers to enhance user interaction on your website.
Understanding JavaScript Events
An event is any action that takes place on a web page, such as a mouse click, a key press or a scroll. JavaScript events are triggered when a user performs one of these actions and can be used to modify the content of the page or trigger an action, such as displaying a popup or playing a sound.
Here is an example of how to create a basic click event in JavaScript:
1document.getElementById("myButton").addEventListener("click", function() {
2 alert("Button Clicked!");
3});
In the example above, we use the addEventListener()
method to add a click event to the "myButton" element on the web page. When the button is clicked, the function defined in the second argument of addEventListener()
is executed and an alert box with the message “Button Clicked!” is displayed.
Types of JavaScript Events
JavaScript supports a wide range of events, each of which can be used to trigger different actions on a web page. Some commonly used events include:
click
: Triggered when the user clicks an element on the web page.submit
: Triggered when the user submits a form.keydown
: Triggered when the user presses a key.load
: Triggered when the page finishes loading.
You can find a complete list of JavaScript events here.
Using Event Handlers
Event handlers are JavaScript functions that are executed when an event is triggered. You can use event handlers to perform a wide range of actions, such as changing the content of a web page, validating user input, or playing multimedia content.
Here is an example of how to use an event handler to change the content of a web page:
1document.getElementById("myButton").addEventListener("click", function() {
2 document.getElementById("myText").innerHTML = "Hello World!";
3});
In this example, when the user clicks the "myButton" element, the contents of the "myText" element on the web page are changed to “Hello World!” using the innerHTML
property.
Best Practices for Using Events and Event Handlers
To ensure the best user experience, it’s important to use events and event handlers in a way that is efficient and easy to understand. Here are some best practices to keep in mind:
- Use descriptive event names that are easy to understand.
- Avoid using inline event handlers in HTML, as this can make code difficult to read and maintain.
- Use the
addEventListener()
method to add event handlers programmatically in JavaScript. - Consider using a JavaScript library, such as jQuery or React, to simplify event handling and improve code readability.
Conclusion
JavaScript events and event handlers are powerful tools that can be used to create engaging and interactive web applications. By understanding how events work and following best practices for their use, you can enhance user interaction on your website and create an enjoyable user experience.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
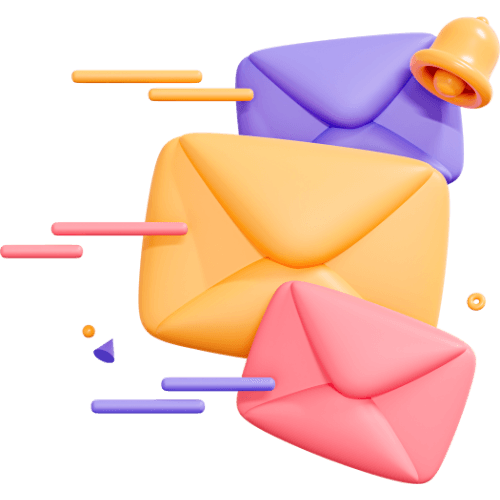
Related articles
9 Articles
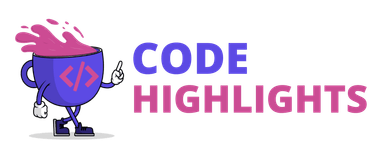
Copyright © Code Highlights 2025.