JavaScript eval() Method

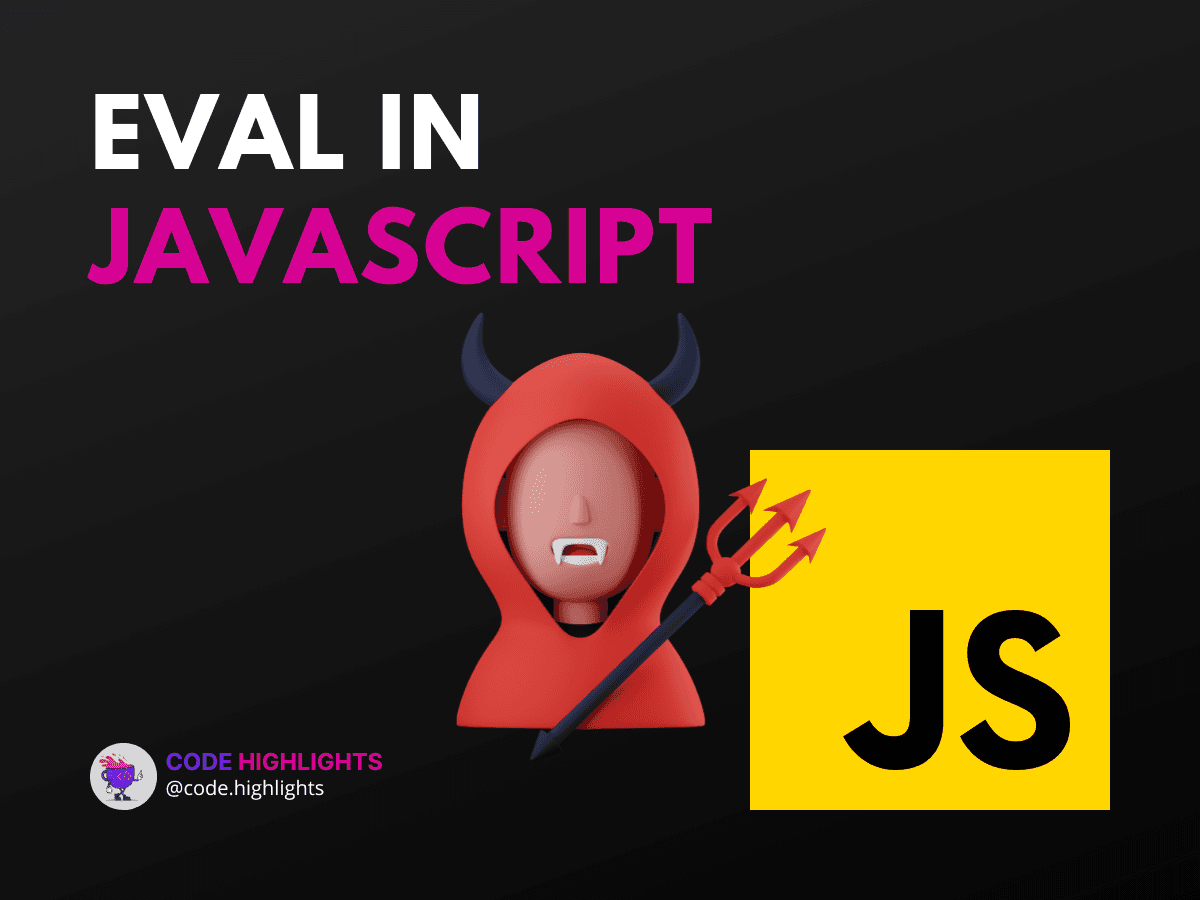
- What is eval() in JavaScript?
- Is JavaScript eval safe?
- What replaced eval in JavaScript?
- Is eval faster than new Function?
- Conclusion
JavaScript eval() Method has stirred debate over its use for years. Let's dive into what eval()
is, explore its risks, discuss its alternatives, and understand where it stands in modern JavaScript development.
In this simple example, we've used eval()
to execute a string expression that evaluates to 4. But there's much more to eval()
than just calculating expressions.
What is eval() in JavaScript?
The eval()
function in JavaScript takes a string argument and executes it as if it were code. This can be incredibly powerful, as it allows for dynamic execution of JavaScript code. However, with great power comes great responsibility.
Here, eval()
dynamically calculates the sum of the numbers in the string stored in the formula
variable. This might seem handy, but let's explore why developers are advised to use this function cautiously.
Is JavaScript eval safe?
Safety is a paramount concern when using eval()
in JavaScript. The primary issue with eval()
is that it can run any JavaScript code, which includes potentially harmful scripts. If eval()
is used to execute user-provided data, it could lead to security vulnerabilities, such as code injection attacks.
1// An example of a potentially dangerous use of eval()
2let userInput = '2; alert("This is unsafe!")';
3eval(userInput); // This will execute the alert, an unintended side effect.
In this example, the eval()
call executes an alert that was not intended to be part of the operation, demonstrating how user input can lead to unexpected consequences.
What replaced eval in JavaScript?
Given the security concerns, alternatives to eval()
have become more prevalent. For parsing JSON strings, JSON.parse()
is now widely recommended. It's safer and more predictable:
1let jsonStr = '{"name": "Alice", "age": 25}';
2let jsonObj = JSON.parse(jsonStr);
3console.log(jsonObj.name); // Outputs: Alice
For dynamic function creation, new Function()
is an alternative that offers similar functionality without some of the risks associated with eval()
.
While new Function()
is not a direct replacement for all eval()
use cases, it serves as a safer option when creating functions from strings.
Is eval faster than new Function?
Performance is another consideration. Historically, eval()
has been slower because it does not allow for optimizations that JavaScript engines apply to pre-parsed code. The new Function()
syntax, while still not as performant as static code, allows for some optimizations since the JavaScript engine knows it's dealing with a function.
Conclusion
The eval()
function in JavaScript is a powerful yet controversial feature. It's important to understand its risks and to know the safer alternatives available. For those looking to deepen their understanding of JavaScript, consider exploring courses like Learn JavaScript, HTML Fundamentals Course, Introduction to CSS, and Introduction to Web Development.
Remember that while eval()
can be a quick solution for certain problems, the potential security risks often outweigh the benefits. As a responsible developer, always prioritize the safety and integrity of your applications by choosing the right tools for the job.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
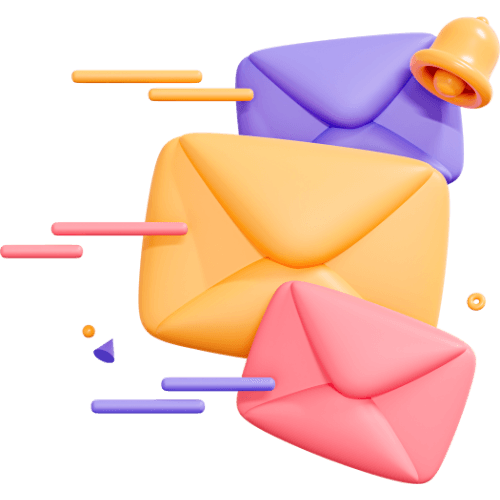
Related articles
9 Articles
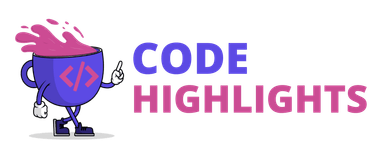
Copyright © Code Highlights 2025.