Ultimate Guide: Find Duplicate in Array JavaScript Efficiently

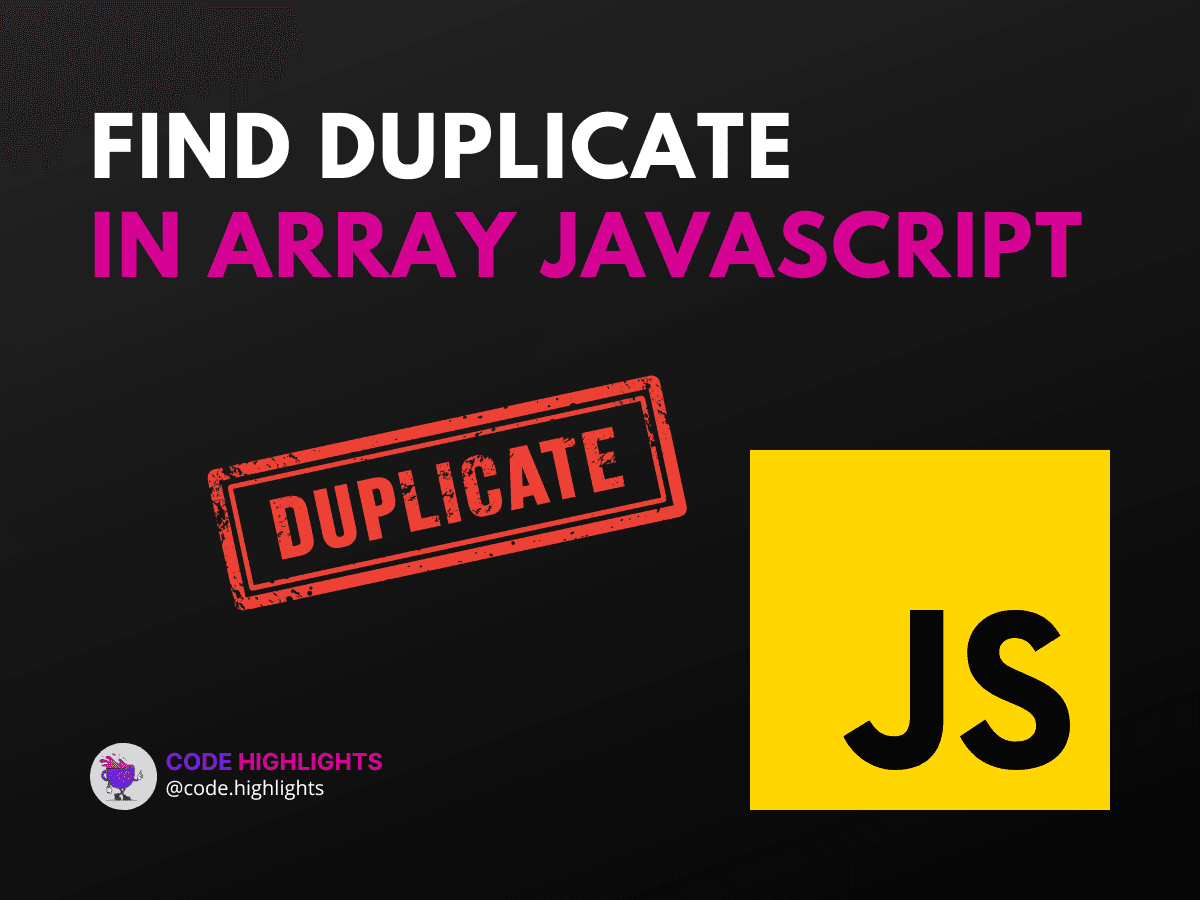
- Finding Duplicates: The Basics
- Using a Loop
- Utilizing a Hash Table
- Counting Duplicates in JavaScript
- Checking for Duplicates Before Insertion
- Conclusion and Further Learning
Discovering duplicates within an array is a common challenge that many developers face. It's a fundamental skill that can be applied in various scenarios, from data validation to algorithm design. In this tutorial, we'll explore how to find duplicate elements in an array using JavaScript, ensuring your code is both efficient and easy to understand.
Let's start with a simple example. Suppose we have an array of numbers, and we want to quickly identify any duplicates:
By the end of this guide, you'll be able to answer this question with confidence. If you're new to JavaScript or need a refresher, consider taking a look at our Learn JavaScript course.
Finding Duplicates: The Basics
When you're looking to find duplicate in array JavaScript, there are several methods to consider. Each approach has its trade-offs regarding performance and readability.
Using a Loop
One of the most straightforward ways to find duplicates is by using a nested loop:
1function findDuplicates(array) {
2 let duplicates = [];
3 for (let i = 0; i < array.length; i++) {
4 for (let j = i + 1; j < array.length; j++) {
5 if (array[i] === array[j]) {
6 duplicates.push(array[i]);
7 break;
8 }
9 }
10 }
11 return duplicates;
12}
13
14console.log(findDuplicates(numbers)); // Output: [2, 4]
This method is simple but not the most efficient, especially for large arrays. It compares each element with every other element, resulting in a time complexity of O(n^2).
Utilizing a Hash Table
To improve efficiency, we can use a hash table (in JavaScript, an object or a Map
) to keep track of seen elements:
1function findDuplicatesEfficient(array) {
2 let seen = new Map();
3 let duplicates = [];
4
5 for (let value of array) {
6 if (seen.has(value)) {
7 duplicates.push(value);
8 } else {
9 seen.set(value, true);
10 }
11 }
12 return duplicates;
13}
14
15console.log(findDuplicatesEfficient(numbers)); // Output: [2, 4]
The findDuplicatesEfficient
function reduces the time complexity to O(n) by avoiding nested loops. This is a significant improvement, especially noticeable with larger datasets.
Counting Duplicates in JavaScript
Sometimes, you might not only want to find repeated elements in an array but also count how many times each element appears. Here's how you can achieve this:
1function countDuplicates(array) {
2 let counts = new Map();
3
4 for (let value of array) {
5 let count = counts.get(value) || 0;
6 counts.set(value, count + 1);
7 }
8
9 return Array.from(counts).filter(([value, count]) => count > 1);
10}
11
12console.log(countDuplicates(numbers)); // Output: [[2, 2], [4, 2]]
The countDuplicates
function provides us with pairs of numbers and their respective counts, but only for those that are duplicates.
Checking for Duplicates Before Insertion
What if you want to check if an array is duplicated before adding a new element? This preemptive check can prevent duplicates from occurring in the first place:
1function addUnique(array, element) {
2 if (array.includes(element)) {
3 console.log(`Duplicate found: ${element}`);
4 } else {
5 array.push(element);
6 }
7}
8
9addUnique(numbers, 8); // Adds the element since it's unique
10addUnique(numbers, 2); // Logs a message because 2 is a duplicate
The includes
method is a convenient way to check for the presence of an element in an array.
Conclusion and Further Learning
Finding and handling duplicates in an array is a common task that can be optimized for better performance. In this tutorial, we've covered several methods to find duplicate in array JavaScript, count them, and check for duplicates before insertion.
For those who want to deepen their understanding of JavaScript, HTML, and CSS, our courses on HTML Fundamentals, Learn CSS Introduction, and Introduction to Web Development are great resources.
Remember, practice makes perfect. Implement these techniques in your projects, and you'll master array manipulation in no time!
For further reading and best practices in JavaScript, consider these reputable sources:
Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
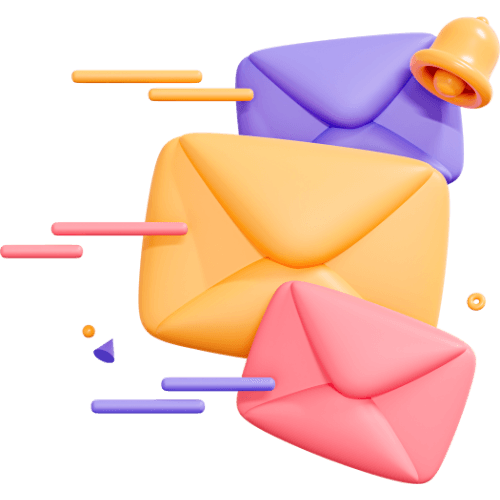
Related articles
9 Articles
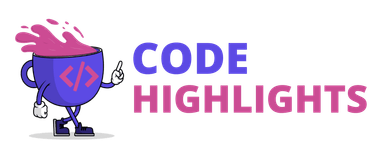
Copyright © Code Highlights 2025.