How to Use FindIndex JavaScript for Efficient Coding

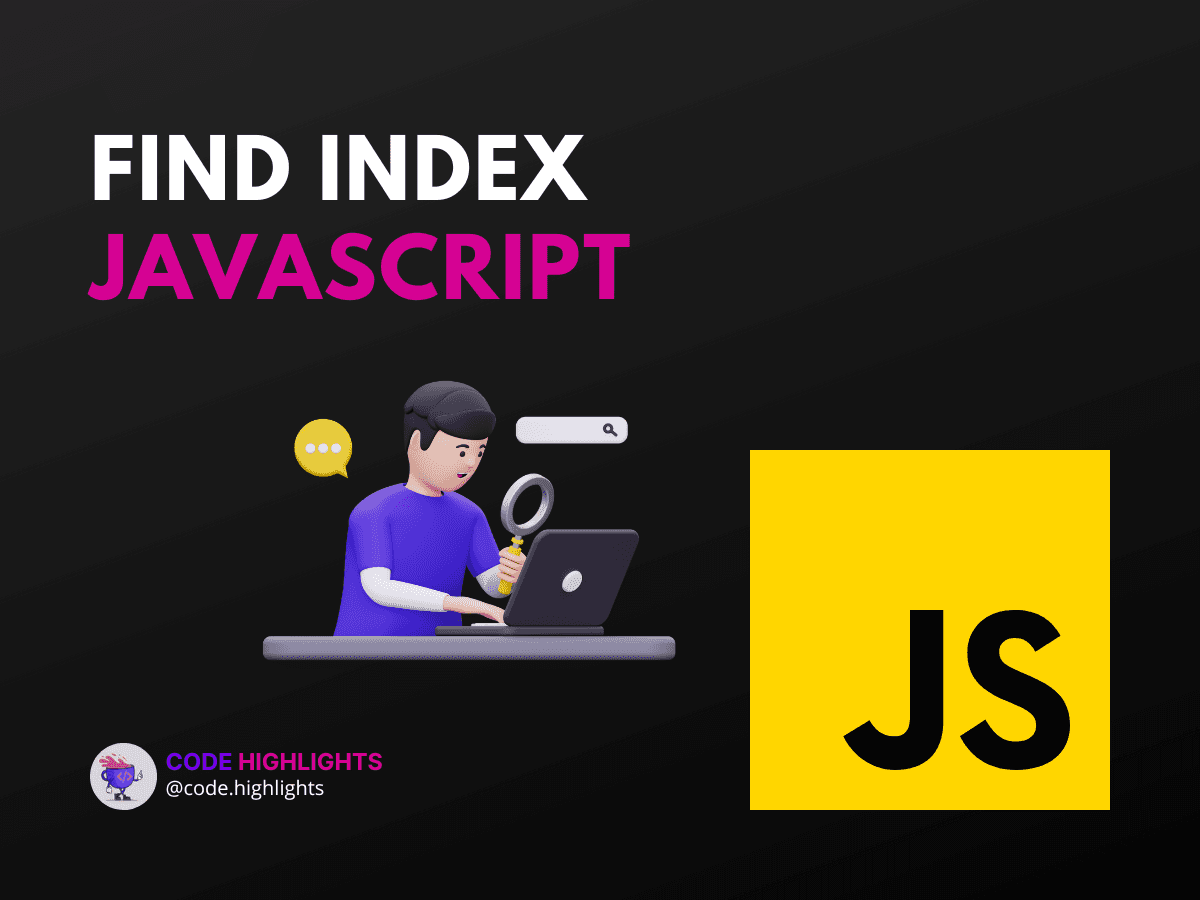
- Understanding Array Indexes in JavaScript
- Utilizing the FindIndex Method
- Step-by-Step Example
- Best Practices for Using FindIndex
- External Resources
JavaScript is a powerful language that enables web developers to create dynamic and interactive websites. One of the fundamental concepts in JavaScript is working with arrays, and a method that stands out for its utility is findIndex
. This method helps you locate the position of an item within an array based on a condition you specify. In this tutorial, we'll explore how to use findIndex
in JavaScript to write more efficient and readable code.
1// Example of findIndex usage
2const fruits = ['apple', 'banana', 'cantaloupe', 'blueberry', 'grapefruit'];
3const index = fruits.findIndex(fruit => fruit === 'blueberry');
4console.log(index); // Output: 3
By the end of this guide, you'll understand how findIndex
operates and how to implement it in your coding projects. If you're new to JavaScript, consider brushing up on the basics with this introductory course.
Understanding Array Indexes in JavaScript
Before diving into findIndex
, let's briefly address "Was ist der Array Index?" or "What is the array index?" in English. An array index is the numerical position of an item within an array, starting from zero. So, in an array of fruits, if 'apple' is the first element, its index is 0. Understanding indexes is crucial for manipulating arrays effectively.
Utilizing the FindIndex Method
The findIndex
method in JavaScript is used to get the index of the first element in an array that satisfies a provided testing function. If no elements satisfy the testing function, it returns -1, indicating that no element has passed the test.
Here's a step-by-step guide to using findIndex
:
- Define an array.
- Create a testing function that returns a boolean value.
- Call
findIndex
on the array, passing the testing function as an argument. - Handle the returned index accordingly.
Step-by-Step Example
1const numbers = [5, 12, 8, 130, 44];
2
3// Define a testing function
4const isLargeNumber = (element) => element > 13;
5
6// Use findIndex with the testing function
7const largeNumberIndex = numbers.findIndex(isLargeNumber);
8
9console.log(largeNumberIndex); // Output: 3
In this example, findIndex
looks for the first number greater than 13 and returns its index.
To further your understanding of JavaScript arrays, you might want to check out this HTML fundamentals course, which includes array manipulation using HTML interfaces, or this course on CSS introduction to style your array data.
Best Practices for Using FindIndex
When using findindex javascript
methods, there are several best practices to keep in mind:
- Use Arrow Functions: They provide a concise way to write the testing function.
- Check for
-1
: Always check iffindIndex
returns-1
to handle cases where no element matches the condition. - Optimize Your Testing Function: Since
findIndex
stops searching once it finds the first match, ensure your condition is well-optimized for performance.
To see findIndex
in the context of a full application, consider enrolling in an introduction to web development course.
External Resources
To deepen your knowledge, here are a few reputable external resources:
By now, you should have a solid understanding of how to use findIndex
in JavaScript to improve your coding efficiency. Remember to practice what you've learned with different arrays and conditions to become proficient in using this helpful method. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
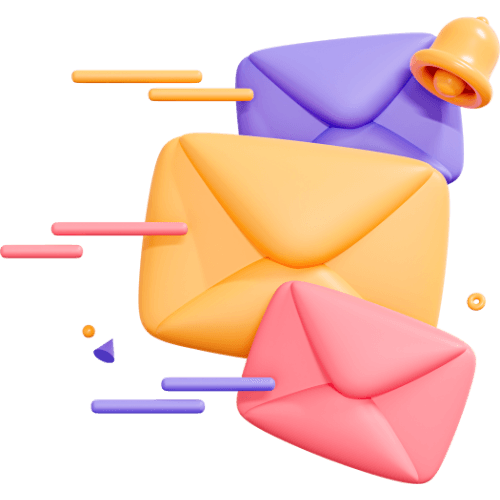
Related articles
9 Articles
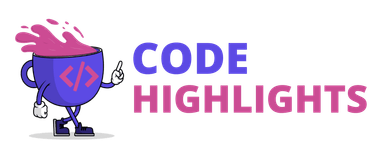
Copyright © Code Highlights 2025.