First Class Citizen JavaScript: 5 Techniques to Elevate Your Code

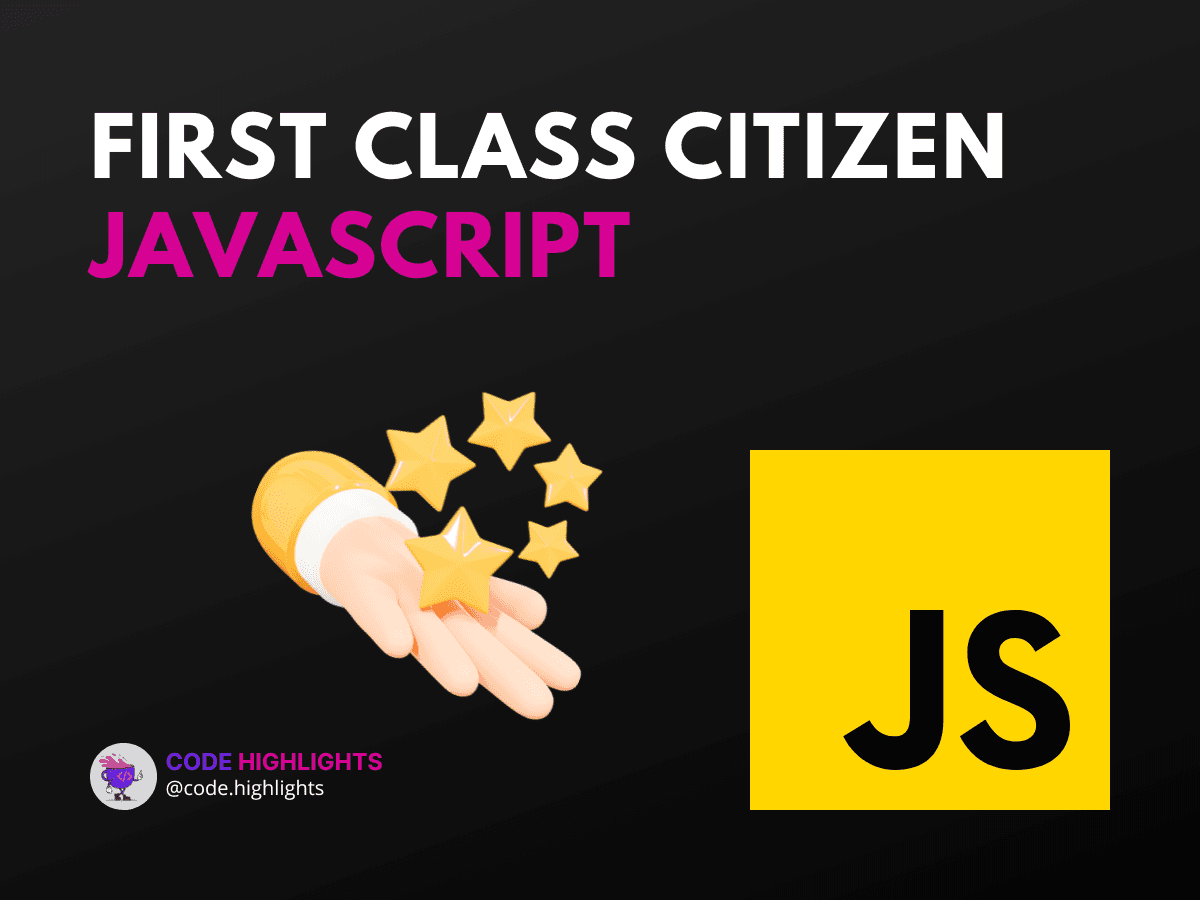
- What is a First-Class Citizen in JavaScript?
- Functions as First-Class Citizens
- Techniques to Utilize First-Class Functions
- Is HTTP a First-Class Citizen in Node.js?
- Are All Entities First-Class Citizens in JavaScript?
Welcome to the world of JavaScript, where the term "first class citizen" is more than just a fancy phrase—it's a fundamental concept that can transform the way you write and understand code. In this tutorial, we'll dive into what makes an entity in JavaScript a first-class citizen and why it matters. By the end, you'll be equipped with five powerful techniques to leverage this concept and elevate your coding skills.
Before we begin, let's look at a snippet of JavaScript code to get our feet wet:
1function greet(name) {
2 return `Hello, ${name}!`;
3}
4
5const greeting = greet('Alice');
6console.log(greeting); // Output: Hello, Alice!
In this example, we're defining a function greet
and then using it just like any other value—we store it in a variable, pass it around, and so on. This is possible because, in JavaScript, functions are first-class citizens.
What is a First-Class Citizen in JavaScript?
In JavaScript, a first-class citizen is an entity that can be passed around in the program like any other value. This includes being stored in variables, passed as arguments to functions, returned from functions, and assigned to object properties.
Functions as First-Class Citizens
When we talk about first-class citizens in JavaScript, we often refer to functions. This is because JavaScript treats functions as objects, which means they can be manipulated and passed around just like any other object or primitive value.
For instance, if you've ever used callback functions or higher-order functions, you've taken advantage of the fact that functions are first-class citizens. Let's explore why this is such a game-changer for your code.
Techniques to Utilize First-Class Functions
- Callback Functions: By passing functions as arguments to other functions, you can create highly customizable and dynamic code. Callbacks are essential in asynchronous operations, such as event handling or fetching data from a server.
1function processData(data, callback) {
2 // Imagine we process the data here
3 callback(data);
4}
5
6processData({ id: 1, name: 'Bob' }, result => console.log(result));
- Higher-Order Functions: These are functions that either take other functions as arguments or return them as results. Higher-order functions are pillars of functional programming and can help you write clean, modular code.
1function createMultiplier(multiplier) {
2 return function(number) {
3 return number * multiplier;
4 };
5}
6
7const double = createMultiplier(2);
8console.log(double(5)); // Output: 10
-
Function Composition: You can combine simple functions to build more complex ones. This technique allows you to compose new behavior by chaining functions together.
-
Currying: Transform a function that takes multiple arguments into a sequence of functions, each with a single argument. This technique can make your functions more flexible and reusable.
-
Closures: Functions can have "memories" by enclosing their surrounding state. Closures allow you to keep state in a functional context, which can be incredibly powerful when used correctly.
Is HTTP a First-Class Citizen in Node.js?
While functions are the primary focus when discussing first-class citizens in JavaScript, it's worth noting that Node.js treats HTTP as a first-class module. This means that HTTP-related functions are readily available and integral to Node.js, enabling you to build web servers and clients with ease.
Are All Entities First-Class Citizens in JavaScript?
Not all entities in JavaScript are considered first-class citizens. For example, while functions certainly fit the bill, other constructs like classes or HTML elements have limitations on how they can be passed around or manipulated.
To learn more about JavaScript and its capabilities, consider enrolling in this comprehensive JavaScript course. If you're also interested in building web pages, the HTML fundamentals course and the introduction to CSS will provide you with the necessary knowledge to start creating stunning websites. And to tie it all together, the introduction to web development course can help you understand how these technologies work together.
In conclusion, understanding and utilizing the concept of first-class citizens in JavaScript can significantly improve your coding practices. By treating functions as values and leveraging the techniques we've discussed, you can write more expressive, flexible, and maintainable code.
Don't forget to check out reputable sources like MDN Web Docs and JavaScript.info for further reading and deep dives into JavaScript concepts.
Remember, practice makes perfect. So, try implementing these techniques in your next project and watch your JavaScript skills soar to new heights!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
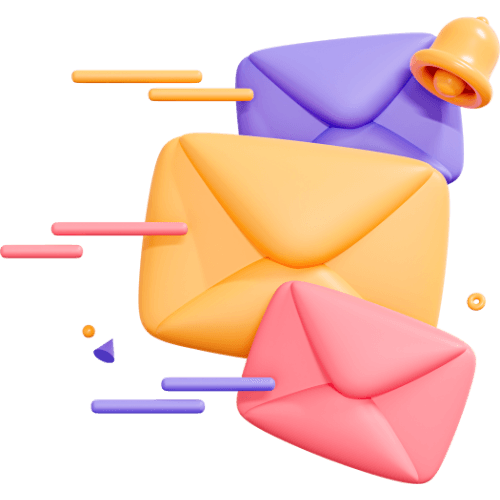
Related articles
9 Articles
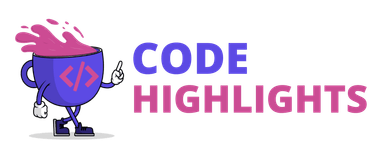
Copyright © Code Highlights 2024.