What Every Developer Must Know About For Loop in JavaScript Array

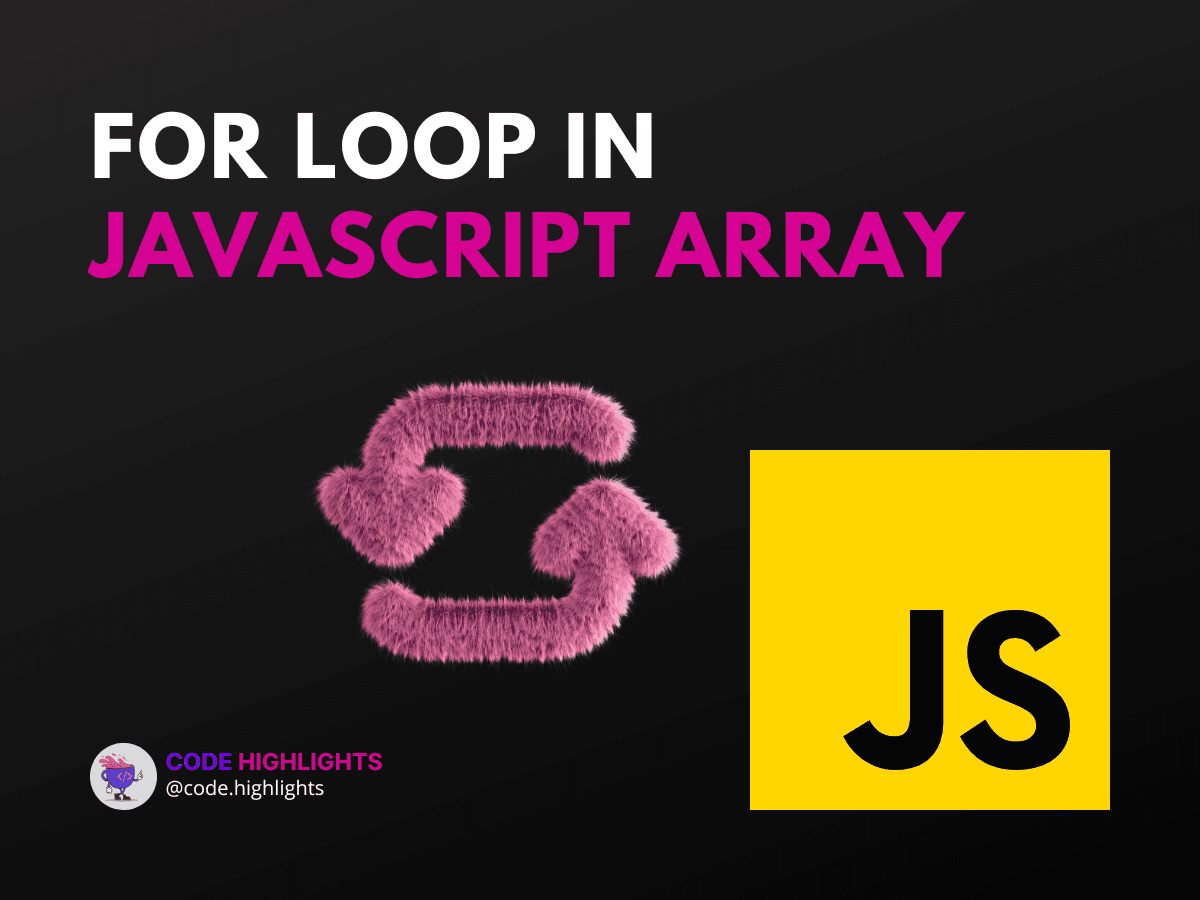
- Understanding the For Loop
- How to Apply a Loop to an Array
- How to Iterate Through an Array
When you start diving into the world of JavaScript, one of the fundamental concepts you'll encounter is looping through arrays. Arrays are like treasure chests in coding; they store a collection of items like numbers, strings, or even other arrays. But how do you unlock the treasures within? Enter the for loop
, a versatile and powerful tool for iterating over these collections. Let's embark on a journey to understand how to use a for loop in JavaScript array
.
1let fruits = ['Apple', 'Banana', 'Cherry'];
2for (let i = 0; i < fruits.length; i++) {
3 console.log(fruits[i]);
4}
In the code snippet above, we're introduced to the syntax of a for loop
that cycles through an array of fruits, logging each one to the console. But there's much more to it than just cycling through elements. Read on to discover the ins and outs of this essential programming technique.
Understanding the For Loop
A for loop
is a control structure used to repeat a block of code a certain number of times, which is particularly useful when dealing with arrays. To answer the question, "Can you use a for in loop on an array JavaScript?" — Absolutely! The traditional for loop
, as shown in our introductory example, is tailor-made for arrays.
How to Apply a Loop to an Array
Applying a for loop
to an array is straightforward:
- Start by declaring the loop with the
for
keyword. - Initialize a counter variable, usually
i
, to track the current index. - Set up a condition that runs until the counter reaches the array length.
- Increment the counter after every loop iteration.
Here's how you can get array values using a for loop
in JavaScript:
1let colors = ['Red', 'Green', 'Blue'];
2for (let i = 0; i < colors.length; i++) {
3 console.log(colors[i]); // Output each color
4}
This loop will iterate through the colors
array and log each color to the console.
How to Iterate Through an Array
Iterating through an array means accessing each element one by one. While the traditional for loop
is common, JavaScript also offers other methods to iterate through arrays, such as the forEach
method and the for...of
loop. If you're interested in expanding your JavaScript knowledge, consider checking out a comprehensive JavaScript course.
For those who are also learning the building blocks of web pages, understanding HTML is crucial. You can begin your journey with an HTML fundamentals course. And don't forget about styling; make your websites visually appealing by learning CSS through an introduction to CSS. Once you're comfortable with these technologies, deepen your understanding with an introduction to web development course.
To ensure you're getting accurate information, always refer to reputable sources. The Mozilla Developer Network (MDN), for example, provides an in-depth look at the for
statement in JavaScript.
Let's recap what we've covered so far:
- We answered whether you can use a
for in
loop on an array in JavaScript (yes, you can!). - We discussed how to apply a loop to an array and iterate through it using a
for loop
. - We provided an example of how to get array values using a
for loop
.
By now, you should have a solid understanding of using a for loop in JavaScript array
. Remember, practice makes perfect. So, go ahead and try writing some for loops
of your own to become more comfortable with this essential tool in your developer toolkit. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
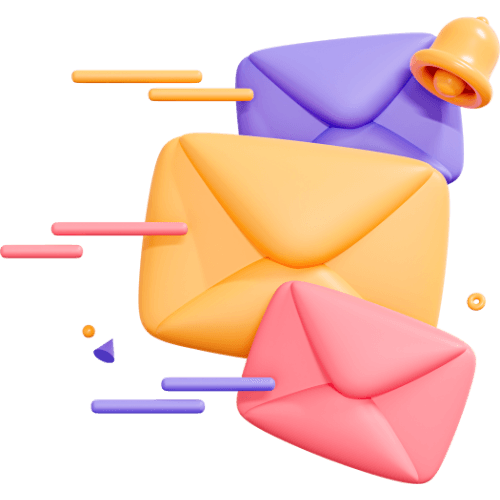
Related articles
9 Articles
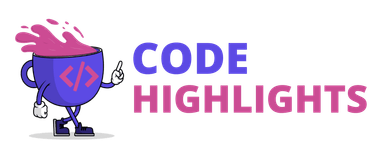
Copyright © Code Highlights 2025.