How to Get Current Month in JavaScript for Accurate Dates

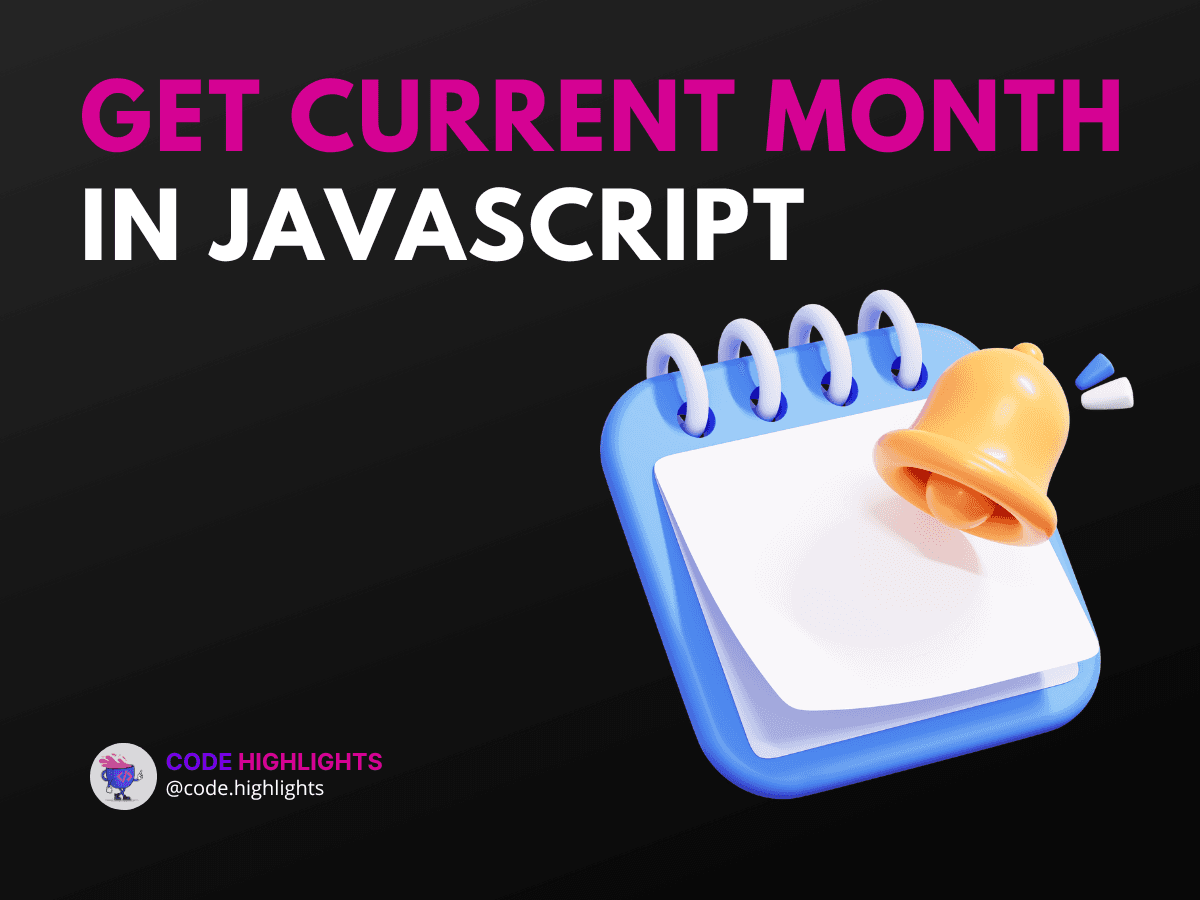
- Quick Code Example
- Understanding the Syntax
- Parameters
- Return Value
- Variations
- Example 1: Getting the Current Month Number
- Code Snippet
- Example 2: Displaying the Month Name
- Code Snippet
- Example 3: Using Moment.js to Get the Current Month
- Code Snippet
- Compatibility with Major Browsers
- Summary
JavaScript is a powerful language that helps developers create dynamic web applications. One common task is to get the current month in JavaScript, which is essential for date-related functionalities. Whether you're building a calendar app or simply displaying the date, knowing how to retrieve the current month can enhance your project. In this tutorial, we’ll explore various ways to achieve this, including using built-in methods and libraries.
Quick Code Example
Before diving into details, here’s a quick example to get you started:
1const currentMonth = new Date().getMonth() + 1; // Adding 1 because months are zero-indexed
2console.log(currentMonth);
This code snippet will display the current month as a number between 1 (January) and 12 (December).
Understanding the Syntax
To get the current month in JavaScript, you primarily use the Date
object. The syntax looks like this:
Parameters
- No parameters are required for
getMonth()
.
Return Value
- This method returns an integer from 0 to 11, where 0 represents January and 11 represents December.
Variations
You may often want to display the month in a user-friendly way. Here’s how to do that:
1const monthNames = ["January", "February", "March", "April", "May", "June", "July", "August", "September", "October", "November", "December"];
2const currentMonthName = monthNames[new Date().getMonth()];
3console.log(currentMonthName);
Example 1: Getting the Current Month Number
Code Snippet
1const monthNumber = new Date().getMonth() + 1;
2console.log(`Current Month Number: ${monthNumber}`);
This example demonstrates how to retrieve the current month as a number. Remember, you need to add 1 since getMonth()
starts counting from zero.
Example 2: Displaying the Month Name
Code Snippet
1const monthNames = ["Jan", "Feb", "Mar", "Apr", "May", "Jun", "Jul", "Aug", "Sep", "Oct", "Nov", "Dec"];
2const currentMonthName = monthNames[new Date().getMonth()];
3console.log(`Current Month: ${currentMonthName}`);
In this case, we create an array of abbreviated month names and use it to display the current month in a more readable format.
Example 3: Using Moment.js to Get the Current Month
Code Snippet
1// Make sure to include Moment.js in your project
2const currentMonth = moment().format('MMMM');
3console.log(`Current Month using Moment.js: ${currentMonth}`);
If you prefer using libraries, Moment.js makes it easy to work with dates and times. The above code retrieves the full name of the current month.
Compatibility with Major Browsers
The methods discussed are compatible with all major web browsers, including Chrome, Firefox, Safari, and Edge. However, if you decide to use libraries like Moment.js, ensure that they are included properly in your project.
Summary
In this tutorial, we covered how to get the current month in JavaScript. We explored multiple methods, including using the built-in Date
object and the Moment.js library. Understanding how to retrieve the current month is crucial for many applications, especially those dealing with dates. For further learning, check out our courses on HTML Fundamentals, CSS Introduction, and JavaScript.
By mastering these techniques, you'll be better equipped to handle date-related tasks in your projects. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
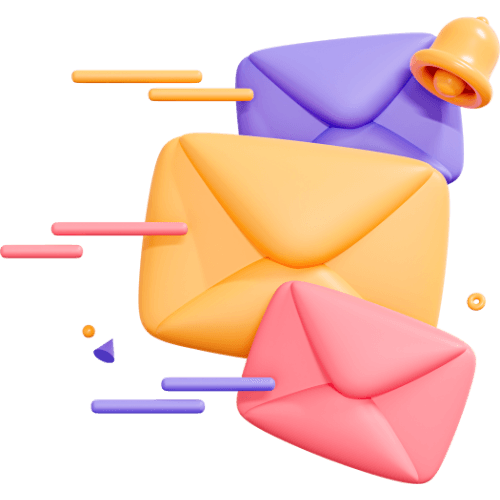
Related articles
9 Articles
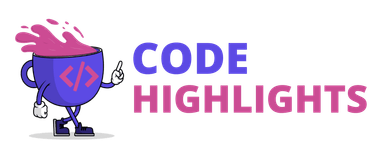
Copyright © Code Highlights 2025.