5 Quick Ways to Get Month Name from Date JavaScript

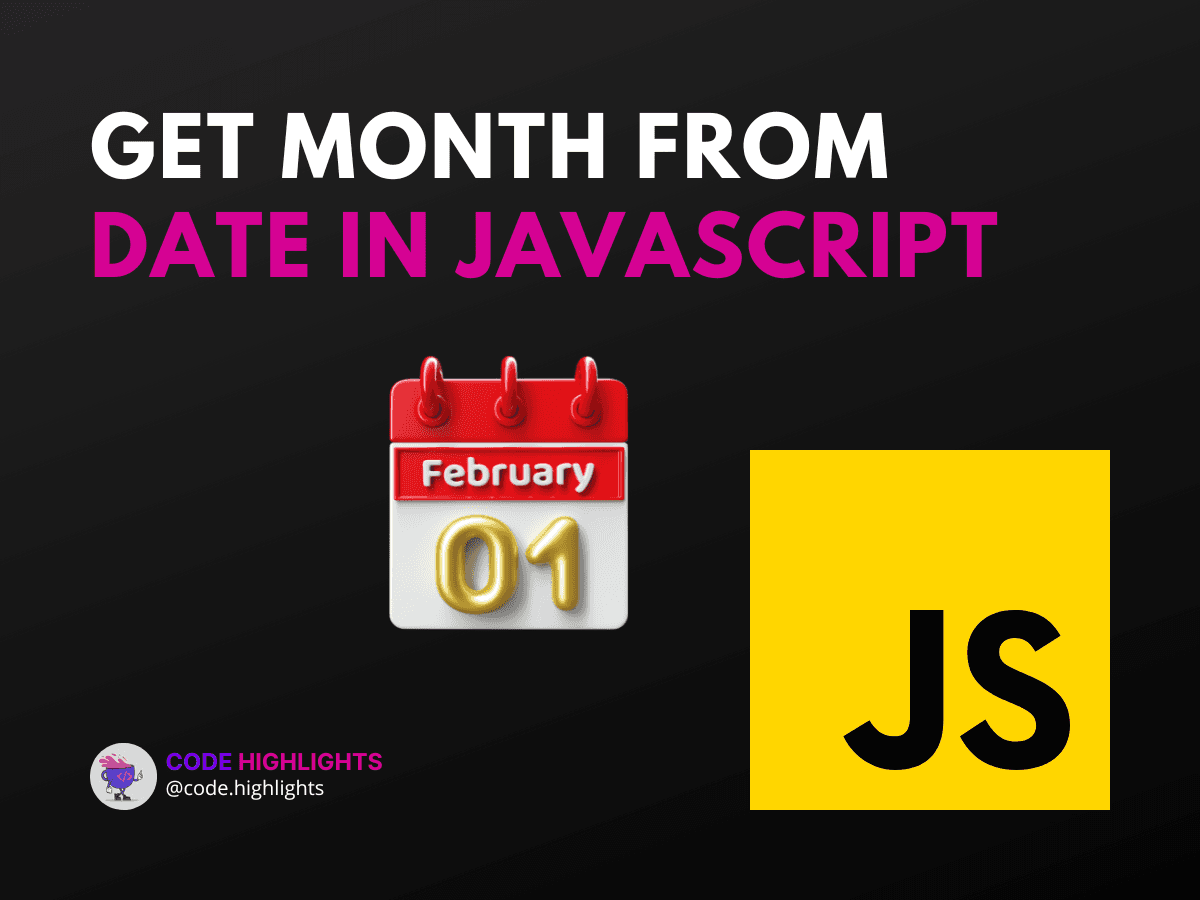
- Method 1: Using toLocaleString()
- Method 2: Array of Month Names
- Method 3: Using Intl.DateTimeFormat()
- Method 4: Using Moment.js
- Method 5: Using Date.prototype.toDateString()
JavaScript is a versatile language that enables web developers to create dynamic and interactive web pages. One common task you might encounter is extracting the month name from a date object. Whether you're building a calendar, scheduling events, or simply displaying dates in a more readable format, knowing how to get the month name from a date is an essential skill. In this tutorial, we'll explore five quick methods to achieve this using JavaScript.
1let exampleDate = new Date();
2console.log(exampleDate.toLocaleString('default', { month: 'long' })); // Outputs the full month name
Method 1: Using toLocaleString()
The toLocaleString()
method is a powerful function that can convert a date to a string, using locale-specific conventions.
1function getMonthName(date) {
2 return date.toLocaleString('default', { month: 'long' });
3}
4
5let currentDate = new Date();
6console.log(getMonthName(currentDate)); // "April" if the current month is April
This method is straightforward and respects localization, which means it will provide the month name in the user's local language if needed.
Method 2: Array of Month Names
For those who prefer a more manual approach, you can define an array with the names of the months and use the getMonth()
method of the Date object.
1const monthNames = [
2 "January", "February", "March",
3 "April", "May", "June",
4 "July", "August", "September",
5 "October", "November", "December"
6];
7
8function getMonthNameFromDate(date) {
9 return monthNames[date.getMonth()];
10}
11
12console.log(getMonthNameFromDate(new Date())); // Returns the current month name
This method gives you control over the exact formatting of the month names and is not dependent on the user's locale.
Method 3: Using Intl.DateTimeFormat()
The Intl.DateTimeFormat()
constructor is part of the Internationalization API, which provides language-sensitive date and time formatting.
1function getMonthFullName(date) {
2 return new Intl.DateTimeFormat('en-US', { month: 'long' }).format(date);
3}
4
5console.log(getMonthFullName(new Date())); // "April"
This method is highly customizable and can be used for various locales, just like toLocaleString()
.
Method 4: Using Moment.js
Moment.js is a popular library for parsing, validating, manipulating, and formatting dates.
1// Assuming Moment.js is included in your project
2const moment = require('moment');
3
4function getMonthNameWithMoment(date) {
5 return moment(date).format('MMMM');
6}
7
8console.log(getMonthNameWithMoment(new Date())); // "April"
While Moment.js is a large library, it's very reliable and has extensive documentation to help you through more complex date manipulations.
Method 5: Using Date.prototype.toDateString()
The toDateString()
method returns the date portion of a Date object in English, which includes the month name.
1function extractMonthName(date) {
2 return date.toDateString().split(' ')[1];
3}
4
5console.log(extractMonthName(new Date())); // "Apr"
This method returns an abbreviated month name and is limited to English.
In conclusion, extracting the month name from a date in JavaScript can be done in multiple ways, depending on your project requirements and preferences. Whether you choose to use built-in JavaScript methods, external libraries like Moment.js, or implement your own solution with an array of month names, you now have the tools to display dates in a user-friendly manner.
While you're mastering JavaScript, don't forget to check out our Learn JavaScript course for more tips and tricks. If you're new to web development, our Introduction to Web Development course is a great place to start, and you can also brush up on your HTML and CSS skills with our HTML Fundamentals Course and CSS Introduction Course.
For more detailed information on JavaScript's Date
object and its methods, the Mozilla Developer Network is an invaluable resource. Additional information on internationalization can be found on ECMA International, and for those interested in Moment.js, their official documentation is thorough and easy to follow.
By choosing the right method for your needs, you'll be able to efficiently get the month name from a date in JavaScript, creating a more polished and professional user experience on your web applications.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
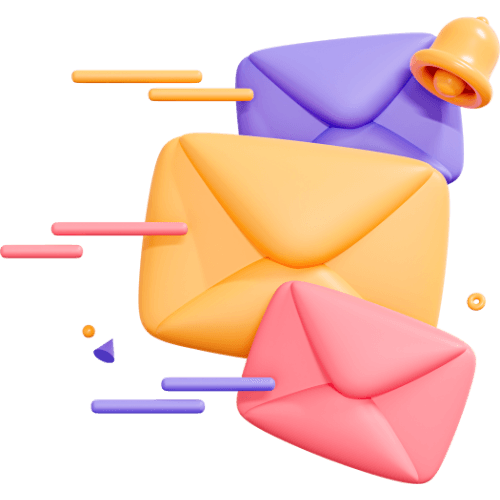
Related articles
9 Articles
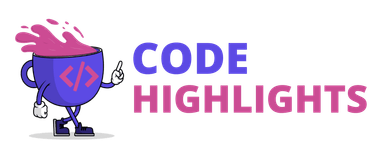
Copyright © Code Highlights 2025.