goto in JavaScript: Understanding Its Usage and Limitations

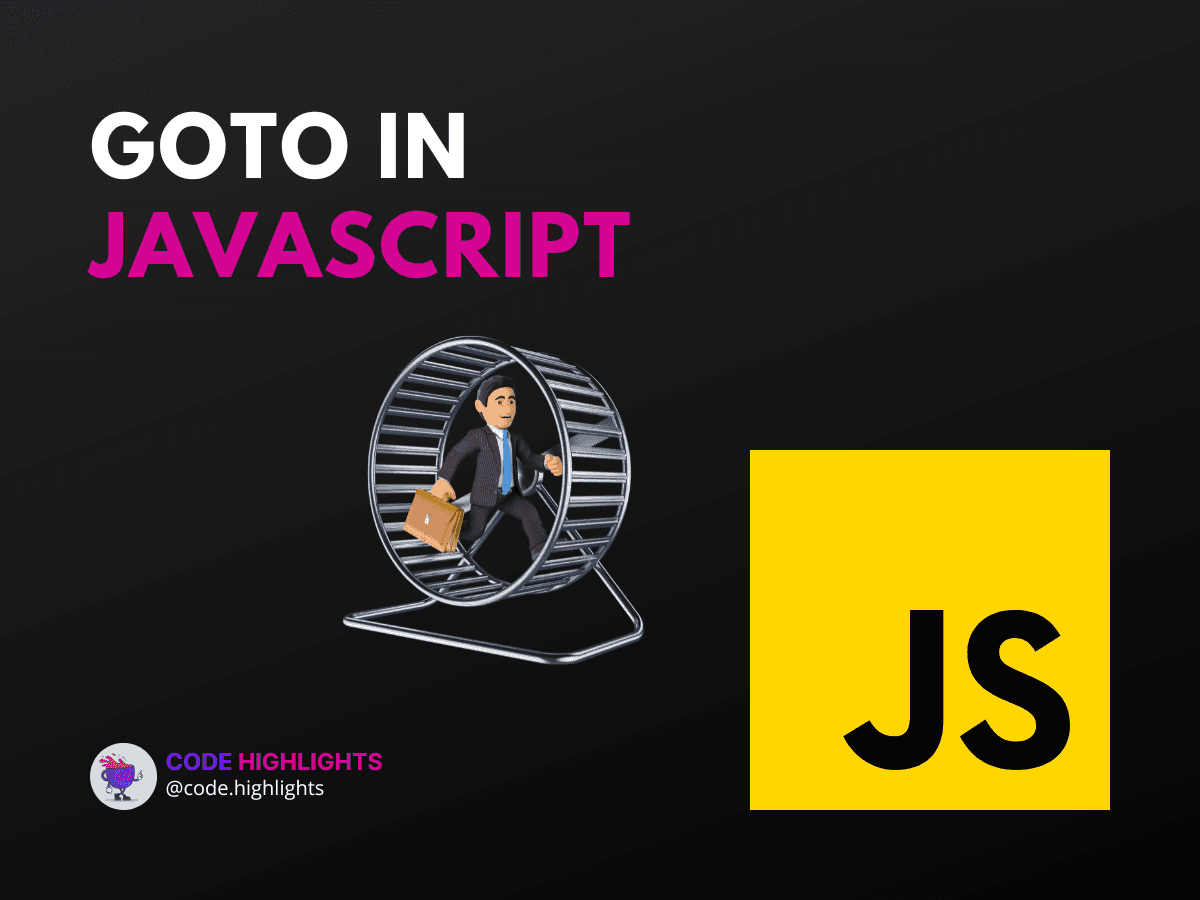
- Why is goto Not Used in JavaScript?
- How to Jump to a Line in JavaScript
- How Do You Go to a Line Number in JavaScript?
- Practical Alternatives to goto in JavaScript
- Conclusion
Have you ever found yourself wishing you could simply jump to a specific section of your code, similar to flipping to a bookmarked page in your favorite novel? If so, you may have wondered if JavaScript supports the goto
statement, a feature known in some programming languages for redirecting the flow of execution to a labeled section of code. In this tutorial, we'll dive into the concept of goto
in JavaScript, explore alternatives, and understand why it's not part of the language's toolbox.
1// Hypothetical example of how one might expect goto to work (not valid in JavaScript)
2// goto label;
3// ... code ...
4// label: console.log("Jumped to here!");
Now, before we jump into the nitty-gritty, let's address a common question: Can you use goto
in JavaScript? The short answer is no. JavaScript does not include a goto
statement due to the complexity and maintainability issues it can introduce. But don't worry! We'll cover how to effectively manage code flow without it.
Why is goto
Not Used in JavaScript?
The goto
statement, while present in some older programming languages, is intentionally excluded from JavaScript. This design decision helps to prevent spaghetti code—a term used to describe tangled, complex, and difficult-to-follow code. Instead, JavaScript encourages structured and clear paths of execution through functions, loops, and conditional statements.
How to Jump to a Line in JavaScript
Although you can't use goto
to jump to a line in JavaScript, you can achieve similar control flow with functions and error handling. Let's explore a practical example:
1function processUserData(userData) {
2 if (!userData) {
3 throw new Error("No user data provided");
4 }
5 // Process user data here
6 console.log("User data processed");
7}
8
9try {
10 processUserData(null);
11} catch (error) {
12 console.log(error.message); // Output: No user data provided
13 // Handle the error or jump to another part of the code
14}
In this example, we simulate a jump by throwing an error when a condition is met and catching it to handle the situation accordingly.
How Do You Go to a Line Number in JavaScript?
Since JavaScript doesn't support line numbers as jump targets, you might wonder how to navigate through your code efficiently. The answer lies in organizing your code into smaller, reusable functions. This way, you can call a function to "jump" to a block of code.
1function goToSection(section) {
2 // Logic to execute the code block related to the section
3 console.log(`Navigating to section: ${section}`);
4}
5
6goToSection('contactForm');
By breaking down your code into functions, you maintain readability and control over the execution flow.
Practical Alternatives to goto
in JavaScript
While you can't use goto
in JavaScript, there are several structured alternatives that can help you manage the flow of your application:
- Functions: Encapsulate code into functions for reuse and clarity.
- Loops: Use
for
,while
, ordo...while
loops to repeat sections of code. - Conditional Statements: Employ
if
,else if
,else
, andswitch
to execute code based on conditions. - Exception Handling: Utilize
try
,catch
,finally
, andthrow
to handle errors and perform jumps in a controlled manner.
Conclusion
While goto
might seem like a convenient way to control the flow of your program, JavaScript provides more structured and maintainable ways to achieve the same results. By using functions, loops, conditional statements, and exception handling, you can write clean, readable, and efficient code.
For those looking to expand their JavaScript knowledge, consider checking out our Learn JavaScript Course. If you're new to web development altogether, our Introduction to Web Development Course is a great place to start. And for those interested in the building blocks of web pages, our HTML Fundamentals Course and Introduction to CSS Course will serve you well.
Remember, writing effective code is about understanding your tools and knowing when and how to use them. Keep learning, keep coding, and you'll find that even without goto
, JavaScript offers a powerful and flexible way to direct your program's flow.
Now it's your turn to put these concepts into practice. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
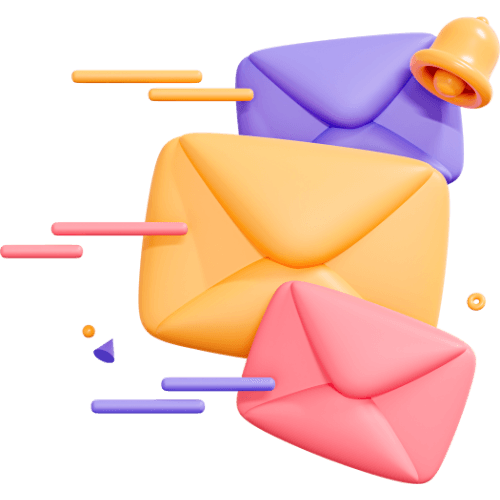
Related articles
125 Articles
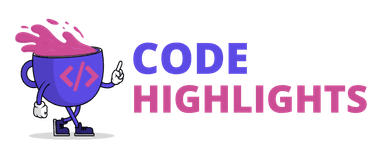
Copyright © Code Highlights 2024.