How to Check If a String is a Number JavaScript: 4 Quick Tips

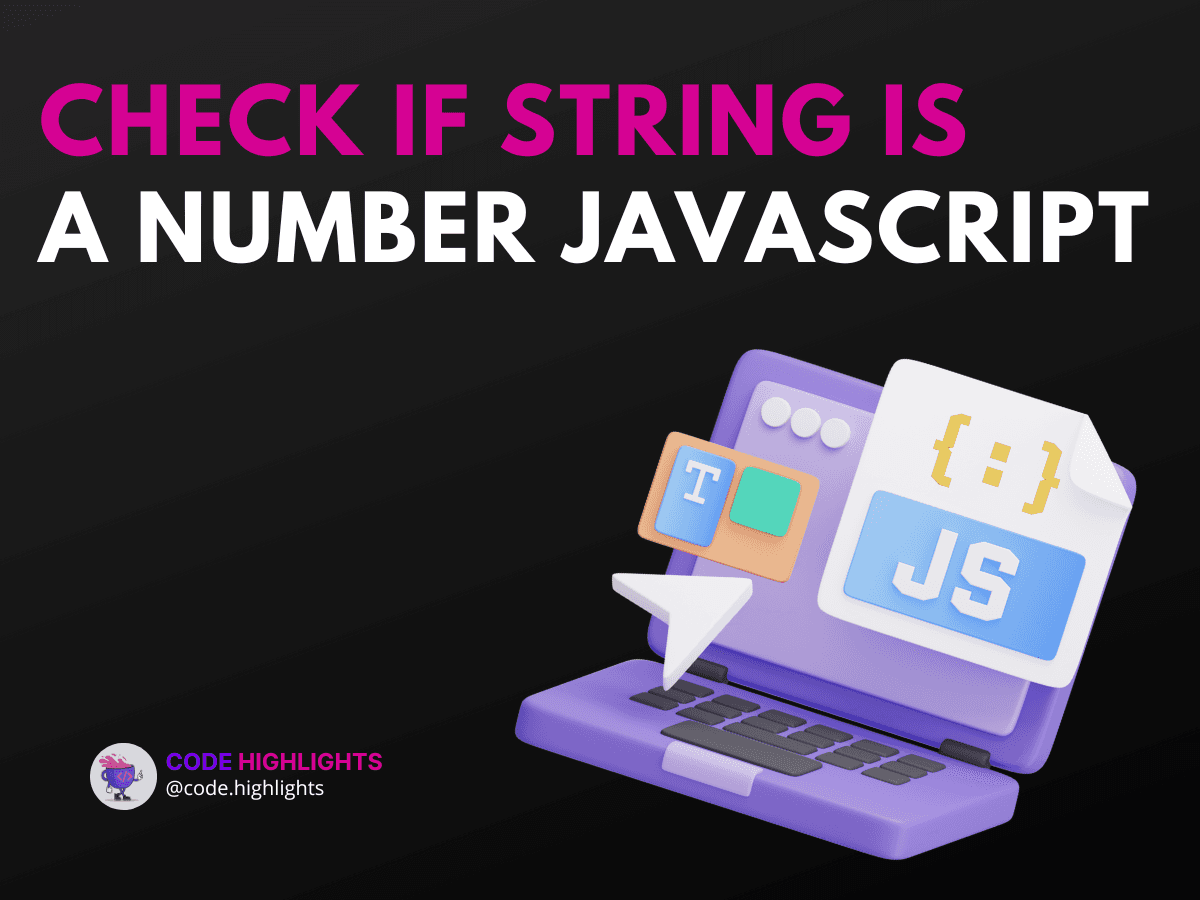
- Quick Code Example
- Understanding the Syntax
- Tip 1: Using isNaN()
- Tip 2: Using Number()
- Tip 3: Using parseFloat()
- Tip 4: Checking if a String Starts with a Number
- Browser Compatibility
- Summary
Checking if a string is a number in JavaScript is a common task, especially when working with user input. Sometimes, you might need to validate data before using it in calculations or saving it to a database. In this tutorial, we'll explore four quick tips to help you determine if a string represents a number. Let’s dive in!
Quick Code Example
Here's a simple code snippet to get started:
This code checks if the variable input
is a number using the isNaN()
function.
Understanding the Syntax
In JavaScript, you can use various methods to check if a string is a number. Here are some key functions and their syntax:
- isNaN(value): Returns
true
if the value is NaN (Not a Number). - Number(value): Converts the value to a number.
- parseFloat(string): Parses a string and returns a floating-point number.
- parseInt(string): Parses a string and returns an integer.
Each of these functions has its own parameters and return values. For example, isNaN()
takes one parameter and returns a boolean.
Tip 1: Using isNaN()
To check if a string is a number, you can use the isNaN()
function. This function determines whether a value is NaN. Here's how you can use it:
1function checkIsNaN(input) {
2 return !isNaN(input);
3}
4
5console.log(checkIsNaN("456")); // true
6console.log(checkIsNaN("abc")); // false
This function returns true
if the input is a number.
Tip 2: Using Number()
Another method is to convert the string to a number using the Number()
function. If the conversion fails, it will result in NaN
.
1function checkNumber(input) {
2 return Number(input) === input;
3}
4
5console.log(checkNumber("789")); // true
6console.log(checkNumber("12abc")); // false
In this example, checkNumber
returns true
for numeric strings and false
otherwise.
Tip 3: Using parseFloat()
If you want to check for decimal numbers, parseFloat()
can be useful. It converts a string to a floating-point number.
1function checkFloat(input) {
2 return !isNaN(parseFloat(input)) && isFinite(input);
3}
4
5console.log(checkFloat("3.14")); // true
6console.log(checkFloat("abc")); // false
This function ensures that the input is a valid finite number.
Tip 4: Checking if a String Starts with a Number
Sometimes, you may want to check if a string starts with a number. You can use a regular expression for this purpose.
1function startsWithNumber(input) {
2 return /^[0-9]/.test(input);
3}
4
5console.log(startsWithNumber("123abc")); // true
6console.log(startsWithNumber("abc123")); // false
This function checks if the first character of the string is a digit.
Browser Compatibility
The methods discussed above are widely supported across all major web browsers, including Chrome, Firefox, Safari, and Edge. This means you can confidently use these techniques in your web applications without worrying about compatibility issues.
Summary
In this tutorial, we learned how to check if a string is a number in JavaScript using four different methods: isNaN()
, Number()
, parseFloat()
, and regular expressions. Each method has its unique use cases, so you can choose the one that best fits your needs. Remember, validating user input is crucial for building robust applications.
For more on JavaScript, consider checking out our JavaScript course or explore other topics like HTML fundamentals and CSS introduction. Happy coding!
For additional reading, you can refer to resources from MDN Web Docs, W3Schools, and JavaScript.info.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
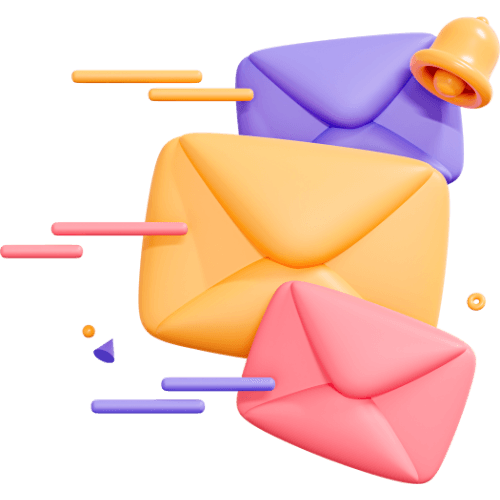
Related articles
9 Articles
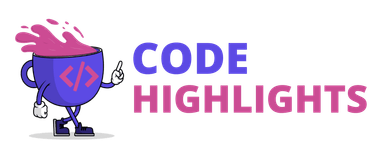
Copyright © Code Highlights 2024.