How to Master img src JavaScript Function for Dynamic Images

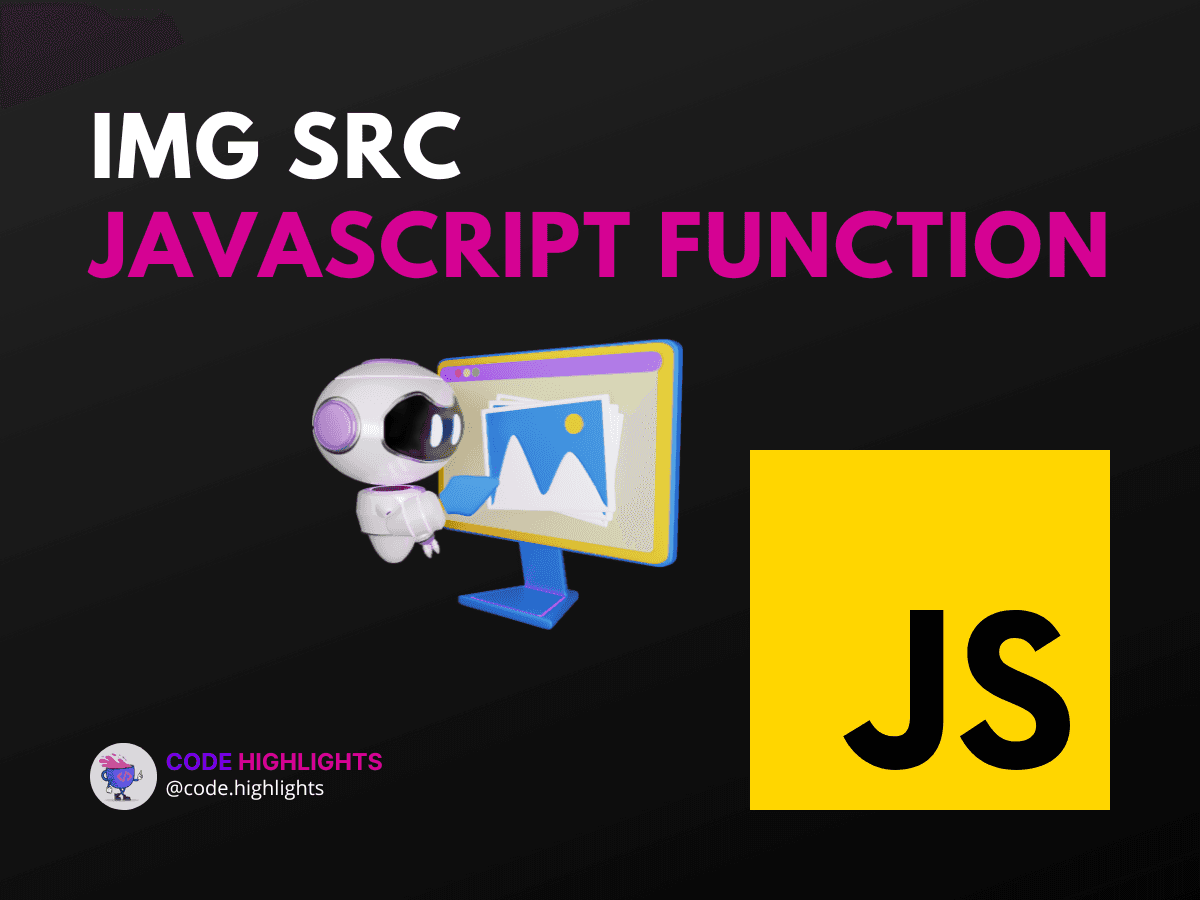
- Understanding the img src JavaScript Function
- Calling img src in JavaScript
- Using JavaScript Variables in img src
- The Role of src Function in JavaScript
- Practical Examples
- Conclusion
When developing web pages, images play a pivotal role in design and user engagement. But what happens when you need to dynamically control images using JavaScript? In this tutorial, we'll explore the img src
JavaScript function, which is essential for manipulating image sources on the fly. Whether you're a budding developer or looking to sharpen your skills, get ready to add a powerful tool to your coding arsenal.
Before we dive into the nitty-gritty, let's look at a basic example of how an image is embedded into HTML using the src
attribute:
1<img src="path-to-your-image.jpg" alt="Descriptive Image Text">
Now, imagine you want to change this image source dynamically using JavaScript. That's exactly what we're about to learn!
Understanding the img src
JavaScript Function
JavaScript provides us with several ways to manipulate the src
attribute of an image element. Let's start by addressing a common question: How do you pass an image src
in a JavaScript function?
Here's a simple example:
In the code above, we have defined a function called setImageSource
that takes one parameter, imagePath
. This function finds an image element with the ID dynamicImage
and sets its src
attribute to the imagePath
provided.
Calling img src
in JavaScript
To call the img src
in JavaScript, you simply need to invoke the function you've created with the desired image path:
This line of code will change the source of the image with the ID dynamicImage
to new-image-path.jpg
.
Using JavaScript Variables in img src
What if you want to use a JavaScript variable in img src
? Here's how you can do that:
By storing the image path in a variable, you can easily pass it around and use it to change the image source dynamically.
The Role of src
Function in JavaScript
While there isn't a built-in src
function in JavaScript, the concept refers to the process of setting the src
attribute of an image element via JavaScript. This enables developers to create interactive and dynamic user experiences. For example, changing an image based on user actions or updating images without reloading the page.
Practical Examples
Let's put our knowledge into practice with some examples.
-
Changing an Image on Button Click
Imagine you have a gallery of images, and you want to change the displayed image when a user clicks a button. Here's how you could set it up:
1<img id="dynamicImage" src="default-image.jpg" alt="Gallery Image">2<button onclick="setImageSource('next-image.jpg')">Next Image</button>With this setup, clicking the button calls our
setImageSource
function and updates the image instantly. -
Rotating Through Images Automatically
You might want to create a slideshow where images change automatically after a certain interval. This can be done using JavaScript's
setInterval
function:
Conclusion
You now know how to master the img src
JavaScript function to control images dynamically. Whether you're looking to enhance your portfolio or build more interactive websites, these techniques are fundamental to modern web development.
Continue to expand your JavaScript knowledge with courses like Learn JavaScript, or solidify your foundational skills with HTML Fundamentals Course, Learn CSS Introduction, and Introduction to Web Development.
For further reading and best practices, consider these external resources:
- Mozilla Developer Network's guide on images
- W3Schools' tutorial on JavaScript image rollover
- CSS-Tricks article on responsive images
By incorporating dynamic image handling in your projects, you can create more engaging and responsive sites that captivate your audience. Keep experimenting with what you've learned, and happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
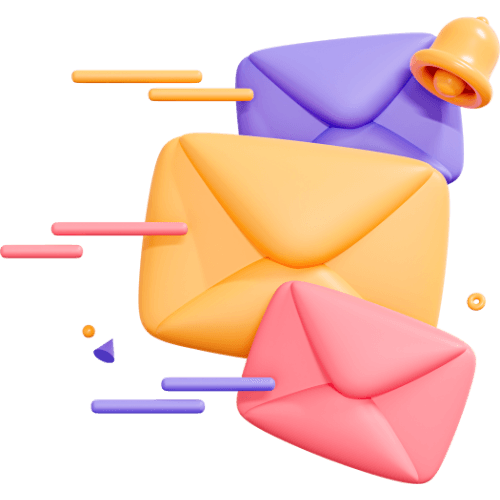
Related articles
9 Articles
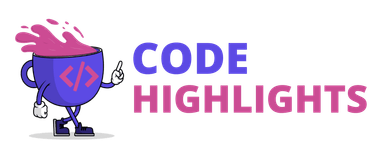
Copyright © Code Highlights 2025.