7 Simple Ways to Insert Character into String JavaScript

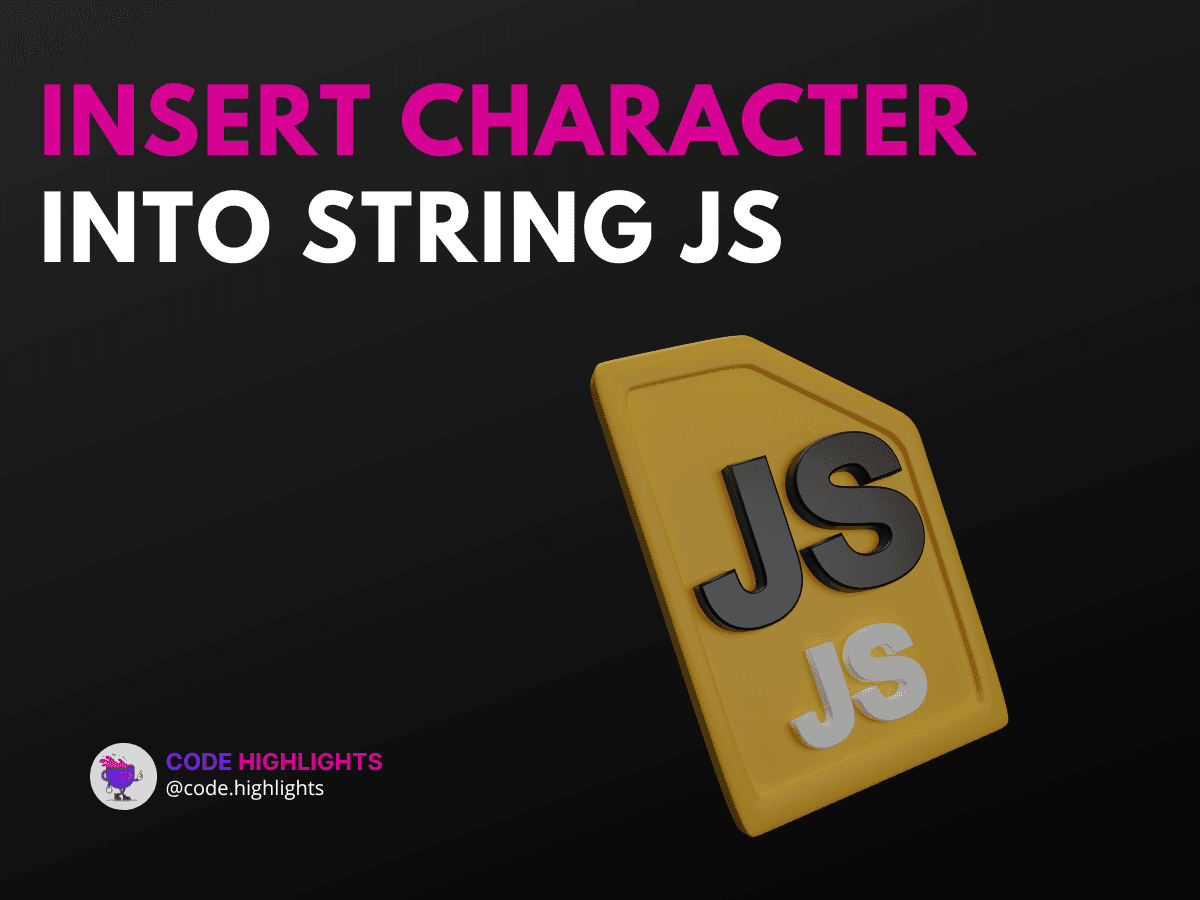
- Understanding String Syntax
- 1. Using slice()
- 2. Using substring()
- 3. Using Template Literals
- 4. Using concat()
- 5. Using Array Methods
- 6. Using Regular Expressions
- 7. Using replace()
- Compatibility with Major Browsers
- Conclusion
Inserting characters into a string in JavaScript can be quite handy. Whether you’re adding a letter, a number, or even a special character, knowing how to do this opens up many possibilities for your code. Let’s dive into some simple methods to insert characters into strings efficiently!
Here’s a quick example to get started:
1let str = "Hello World!";
2let newStr = str.slice(0, 5) + " JavaScript" + str.slice(5);
3console.log(newStr); // Output: Hello JavaScript World!
Understanding String Syntax
In JavaScript, strings are sequences of characters. To manipulate strings, we often use built-in methods. Some key points to remember include:
- Parameters: Strings can be manipulated using various parameters like indices.
- Return Values: Most methods return a new string rather than modifying the original.
For more detailed learning, check out our HTML Fundamentals Course for a solid foundation in web development.
1. Using slice()
The slice()
method can help you insert characters by cutting and joining parts of the string.
1let str = "Goodbye!";
2let newStr = str.slice(0, 3) + " Java" + str.slice(3);
3console.log(newStr); // Output: GooJava dbye!
2. Using substring()
Similar to slice()
, the substring()
method extracts parts of a string.
1let str = "Hello!";
2let newStr = str.substring(0, 3) + " Wonderful" + str.substring(3);
3console.log(newStr); // Output: HelWonderful lo!
3. Using Template Literals
Template literals allow for easier string manipulation with backticks.
1let str = "Coding is fun!";
2let newStr = `${str.slice(0, 7)}awesome ${str.slice(7)}`;
3console.log(newStr); // Output: Coding awesome is fun!
4. Using concat()
The concat()
method joins two or more strings together.
1let str = "I love";
2let newStr = str.concat(" JavaScript!");
3console.log(newStr); // Output: I love JavaScript!
5. Using Array Methods
You can convert a string to an array, modify it, and then join it back.
1let str = "Hello!";
2let arr = str.split('');
3arr.splice(3, 0, ' Java');
4let newStr = arr.join('');
5console.log(newStr); // Output: HelJava lo!
6. Using Regular Expressions
Regular expressions can also help insert characters at specific positions.
1let str = "123456789";
2let newStr = str.replace(/(\d{3})(\d+)/, '$1-');
3console.log(newStr); // Output: 123-456789
7. Using replace()
The replace()
method can replace occurrences of a substring, letting you add characters.
1let str = "Hello!";
2let newStr = str.replace("!", " JavaScript!");
3console.log(newStr); // Output: Hello JavaScript!
Compatibility with Major Browsers
All the methods discussed above are compatible with major web browsers like Chrome, Firefox, Safari, and Edge. This ensures that your string manipulation will work consistently across different platforms.
Conclusion
Inserting a character into a string in JavaScript is straightforward with the right methods. From using slice()
and substring()
to more advanced techniques like regular expressions, you have many options. These skills are essential for effective programming and can enhance your projects.
For further learning, consider exploring our Introduction to Web Development course to deepen your understanding of JavaScript and other web technologies.
By mastering how to insert characters into strings, you can improve your coding skills and create more dynamic applications. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
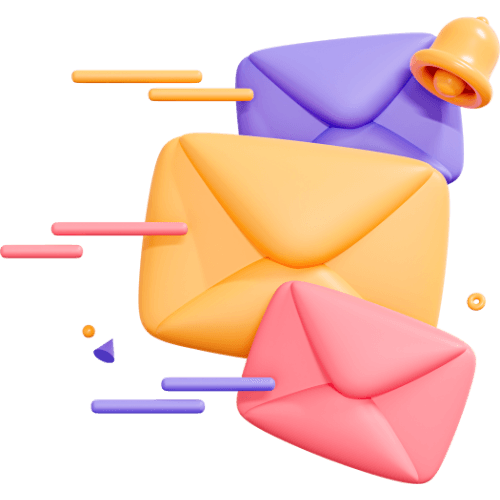
Related articles
9 Articles
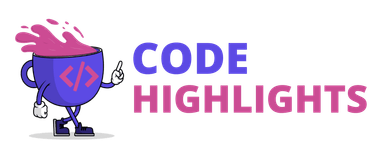
Copyright © Code Highlights 2025.