What is the instanceof JavaScript operator?

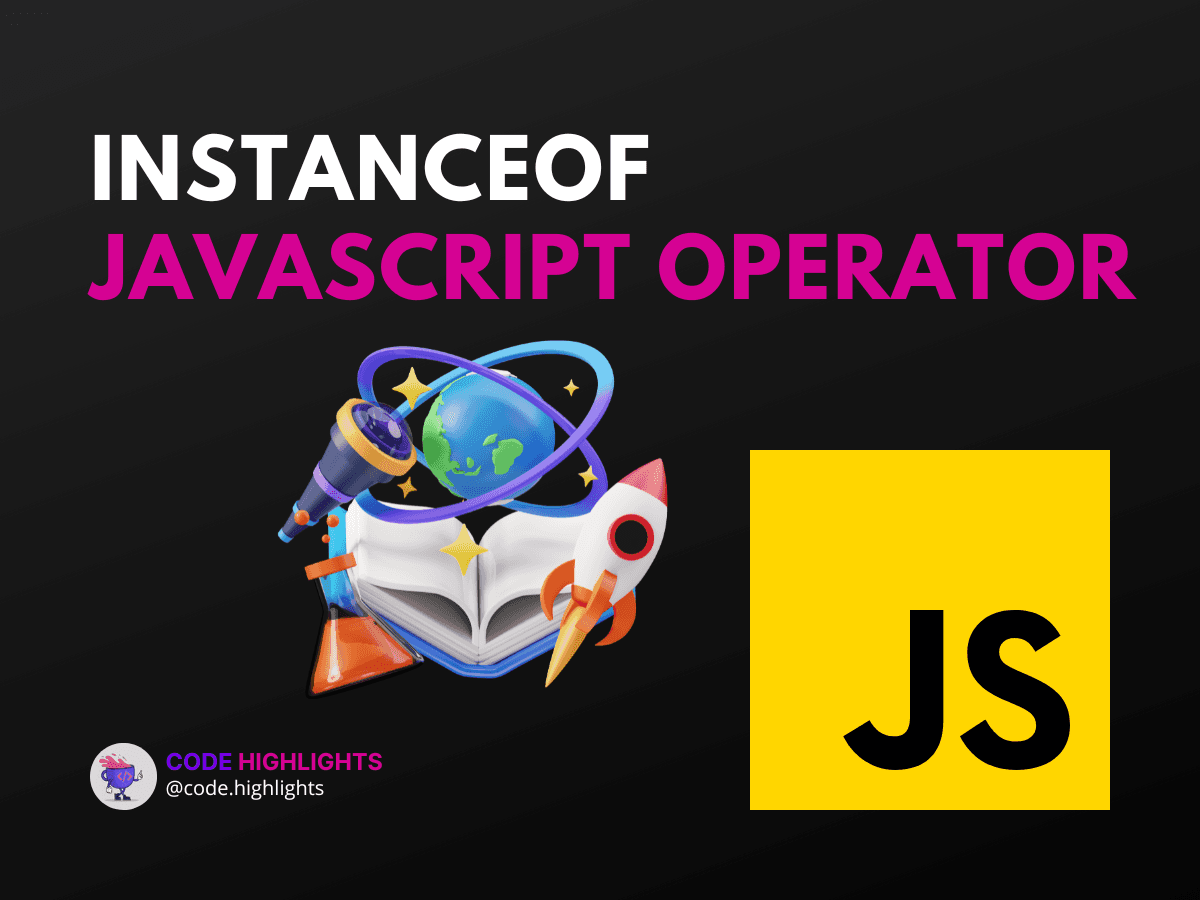
- Understanding instanceof in JavaScript
- typeof vs. instanceof
- What Does "Instance" Mean in JavaScript?
- Checking if an Instance is an Object in JavaScript
- Practical Example: Using instanceof with Custom Objects
- Conclusion
JavaScript is a language teeming with concepts that can seem daunting at first glance. One such concept is the instanceof
operator, a tool in your JavaScript utility belt that's invaluable for understanding and validating the types of objects during runtime. Before we dive into the nitty-gritty, let's look at a simple code snippet:
1class Car {
2 constructor(brand) {
3 this.brand = brand;
4 }
5}
6
7let myCar = new Car("Ford");
8console.log(myCar instanceof Car); // true
This introductory example demonstrates instanceof
in action, checking if myCar
is an instance of the Car
class. Now, let's buckle up and explore the depths of instanceof
in JavaScript.
Understanding instanceof
in JavaScript
The instanceof
operator in JavaScript is used to check whether a specific object is an instance of a class or a constructor function. It helps in determining the prototype chain of an object, which is crucial when dealing with inheritance in JavaScript.
For instance, consider you've just started learning JavaScript through this interactive course. You'll soon discover that JavaScript objects inherit properties and methods from a prototype. The instanceof
operator allows you to verify this relationship between an object and its prototype.
typeof
vs. instanceof
While both typeof
and instanceof
are used for type checking, they serve different purposes. The typeof
operator returns a string indicating the type of the unevaluated operand. It's great for primitive data types like string
, number
, or boolean
. On the other hand, instanceof
checks the prototype chain of an object, making it more suitable for custom objects and complex data types.
Here's how you can distinguish them:
In the above example, typeof
correctly identifies num
as a number, but instanceof
returns false because num
is not an instance of the Number
object.
What Does "Instance" Mean in JavaScript?
In JavaScript, an "instance" refers to a concrete occurrence of any object that has been created using a constructor function or a class. For example:
Here, dateInstance
is an instance of the Date
class.
Checking if an Instance is an Object in JavaScript
In JavaScript, almost everything is an object, including instances created by constructors. To check if an instance is an object, you can use the instanceof
operator or simply check its type:
1console.log(dateInstance instanceof Object); // true
2console.log(typeof dateInstance === 'object'); // true
Both checks confirm that dateInstance
is indeed an object.
Practical Example: Using instanceof
with Custom Objects
Let's create a custom object and see instanceof
at work:
1function Animal(name) {
2 this.name = name;
3}
4let dog = new Animal("Buddy");
5
6console.log(dog instanceof Animal); // true
Here, dog
is verified as an instance of the Animal
constructor.
Conclusion
The instanceof
operator is a powerful feature in JavaScript that aids in type-checking and understanding object inheritance. By using it alongside other JavaScript fundamentals, such as those covered in HTML, CSS, and Web Development, you can craft robust and dynamic web applications.
For further reading on JavaScript's instanceof
and object-oriented programming, check out these external resources:
- MDN Web Docs on instanceof
- Understanding JavaScript Constructors
- JavaScript Inheritance and the Prototype Chain
Remember, practice makes perfect. Experiment with instanceof
to get a feel for its behavior and quirks. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
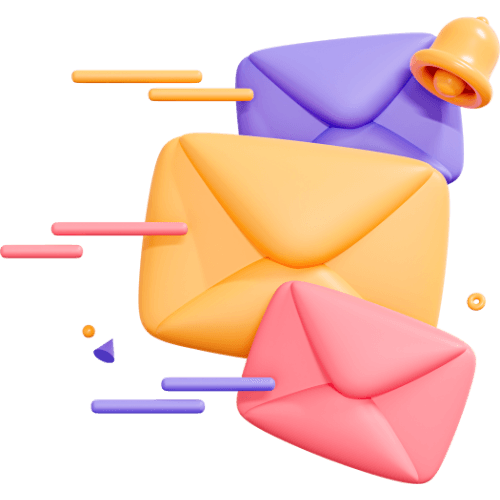
Related articles
9 Articles
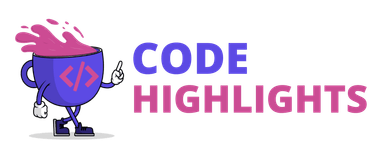
Copyright © Code Highlights 2024.