4 Easy Ways to Iterate Over Arrays in JavaScript

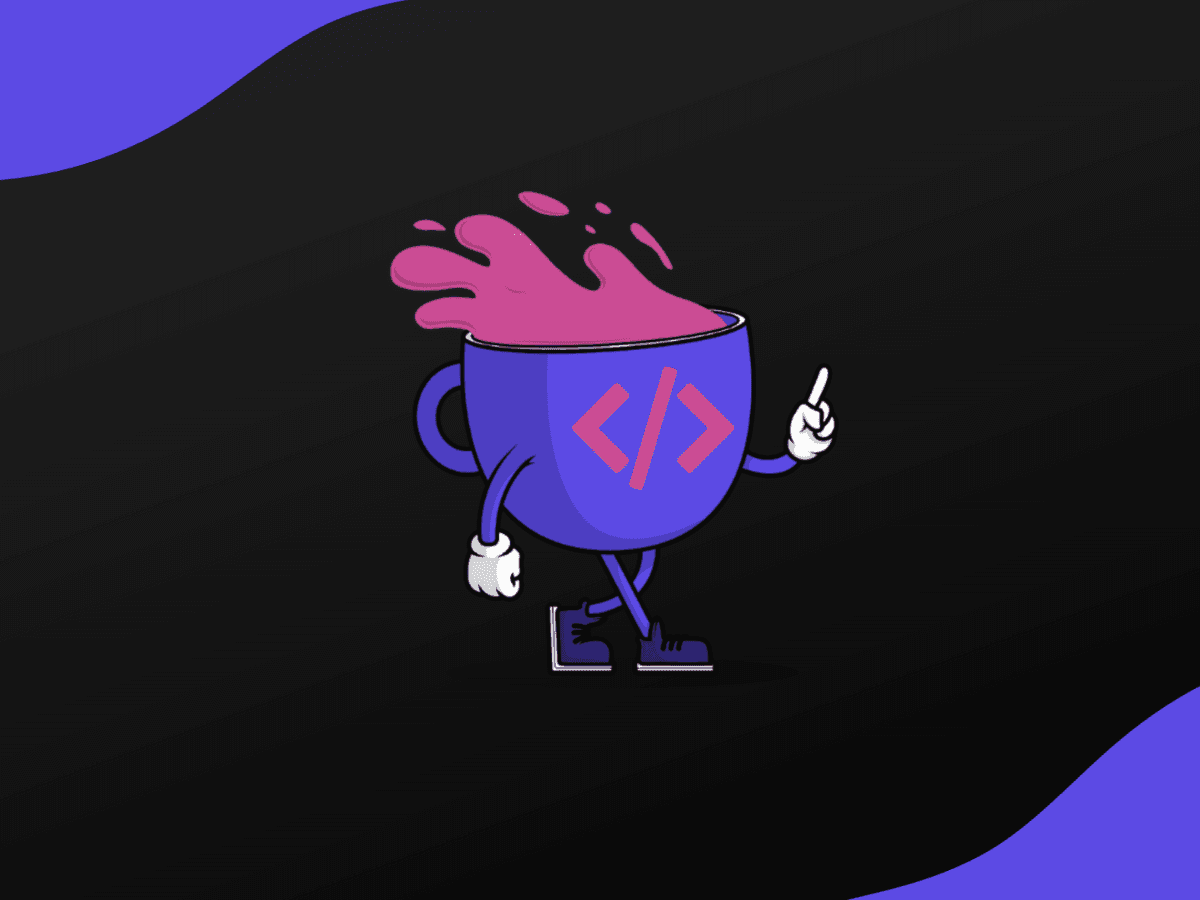
- for Loop
- forEach() Method
- for...of Loop
- map() Method
- Performance Considerations
In JavaScript, arrays are a type of object used for storing multiple values in a single variable. Each value in the array is an element. You can iterate, or loop, over an array in JavaScript in several ways. This tutorial will explore some of the most common methods.
for
Loop
A for
loop is a control flow statement, which allows code to be executed repeatedly. To iterate over an array with a for
loop, you can use the array's length property.
This will log each number on its own line, from 1
to 5
.
forEach()
Method
The forEach()
method executes a provided function once for each array element. This is a more modern, functional programming style and is featured prominently in our JavaScript course.
This will log each string on its own line, from apple
to cherry
.
for...of
Loop
The for...of
statement creates a loop iterating over iterable objects, including: built-in String, Array, array-like objects and more. It combines the best parts of the for
and forEach()
.
This will log each number on its own line, from 10
to 40
.
map()
Method
The map()
method creates a new array with the results of calling a provided function on every element in the calling array.
1var arr = [1, 2, 3, 4, 5];
2var newArr = arr.map(function(element) {
3return element * 2;
4});
5console.log(newArr);
This will log the new array [2, 4, 6, 8, 10]
.
Performance Considerations
The for
loop is the fastest way to iterate over an array in JavaScript, followed by forEach()
, for...of
, and finally map()
. However, the differences are often negligible, and readability or convenience might often dictate your choice.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
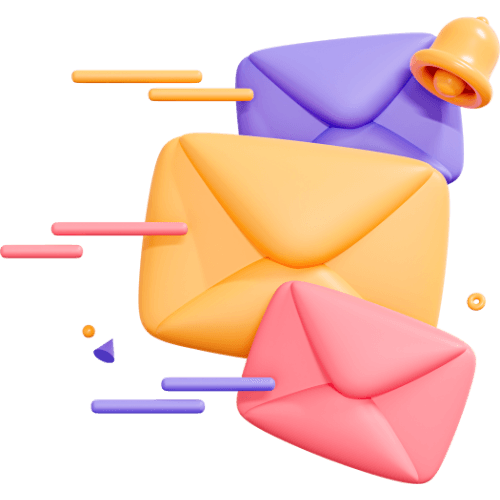
Related articles
9 Articles
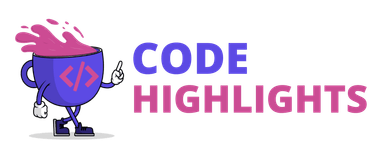
Copyright © Code Highlights 2025.