JavaScript Absolute Value - Math.abs()

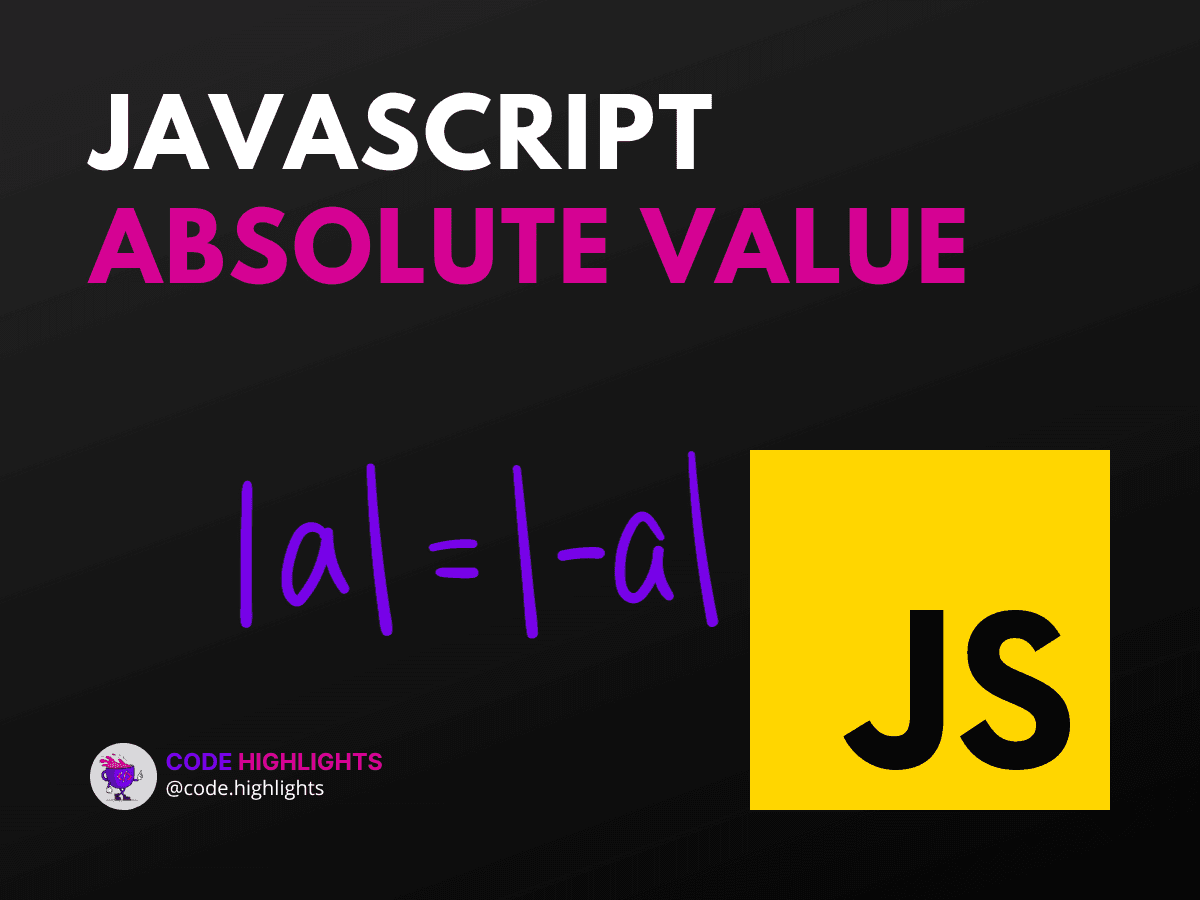
- Understanding Absolute Value in JavaScript
- How to Do Absolute Value in JavaScript?
- How to Do Absolute Value in Code?
- How Do You Find the Absolute Value of a Negative Number in JavaScript?
- What Is the Absolute Value of an Array in JavaScript?
- Practical Applications of Math.abs()
- Conclusion
Are you ready to dive into the world of JavaScript and demystify the concept of absolute values? Whether you're a budding programmer or someone looking to brush up on your coding skills, understanding the Math.abs()
method in JavaScript is essential. This powerful function can transform negative numbers into positive ones with ease, making it a handy tool in various coding scenarios.
In this tutorial, we'll explore how to harness the Math.abs()
method to calculate absolute values, ensuring that you're well-equipped to tackle any challenges that come your way. If you're eager to learn more about JavaScript, consider checking out this comprehensive course. Moreover, if you're new to web development, you might find this introductory course useful to get started.
Understanding Absolute Value in JavaScript
The term "absolute value" refers to the non-negative value of a number without regard to its sign. In other words, it's the distance of a number from zero on the number line, regardless of direction. This concept is not only prevalent in mathematics but also in programming.
How to Do Absolute Value in JavaScript?
JavaScript provides the Math.abs()
method for this very purpose. It's a built-in function that returns the absolute value of a given number. Here's a simple example:
How to Do Absolute Value in Code?
Using the Math.abs()
method is straightforward. You just need to pass the number as an argument to the function:
How Do You Find the Absolute Value of a Negative Number in JavaScript?
To find the absolute value of a negative number, simply use the Math.abs()
method with the negative number as its argument:
What Is the Absolute Value of an Array in JavaScript?
If you have an array of numbers and you want to convert all of them to their absolute values, you can use the map()
function combined with Math.abs()
like so:
1let numberArray = [-1, -2, -3, -4];
2let absoluteArray = numberArray.map(Math.abs);
3console.log(absoluteArray); // Output: [1, 2, 3, 4]
Practical Applications of Math.abs()
The Math.abs()
method is incredibly versatile. It's often used in algorithms that require distance calculations or when ensuring that a calculation yields a positive result. For instance, in financial applications, the absolute difference between two currency values can be crucial.
Conclusion
By now, you should feel comfortable using the Math.abs()
method to compute absolute values in JavaScript. This function is both simple and powerful, making it a valuable addition to your coding toolkit. As you continue your journey in web development, don't forget to strengthen your foundation with courses on HTML and CSS.
For further reading and best practices, check out these external resources:
- Mozilla Developer Network's Math.abs() documentation
- W3Schools JavaScript Math Reference
- JavaScript Info on Numbers
Keep practicing, and soon enough, you'll be crafting code that handles absolute values with finesse. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
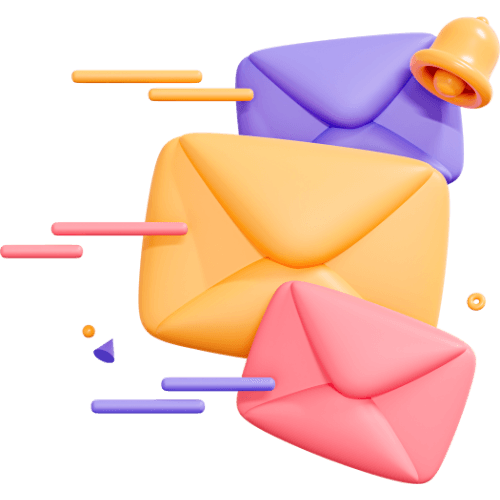
Related articles
9 Articles
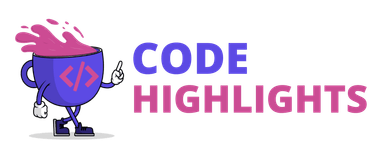
Copyright © Code Highlights 2025.