7 Simple Ways to Javascript Add Key to Object

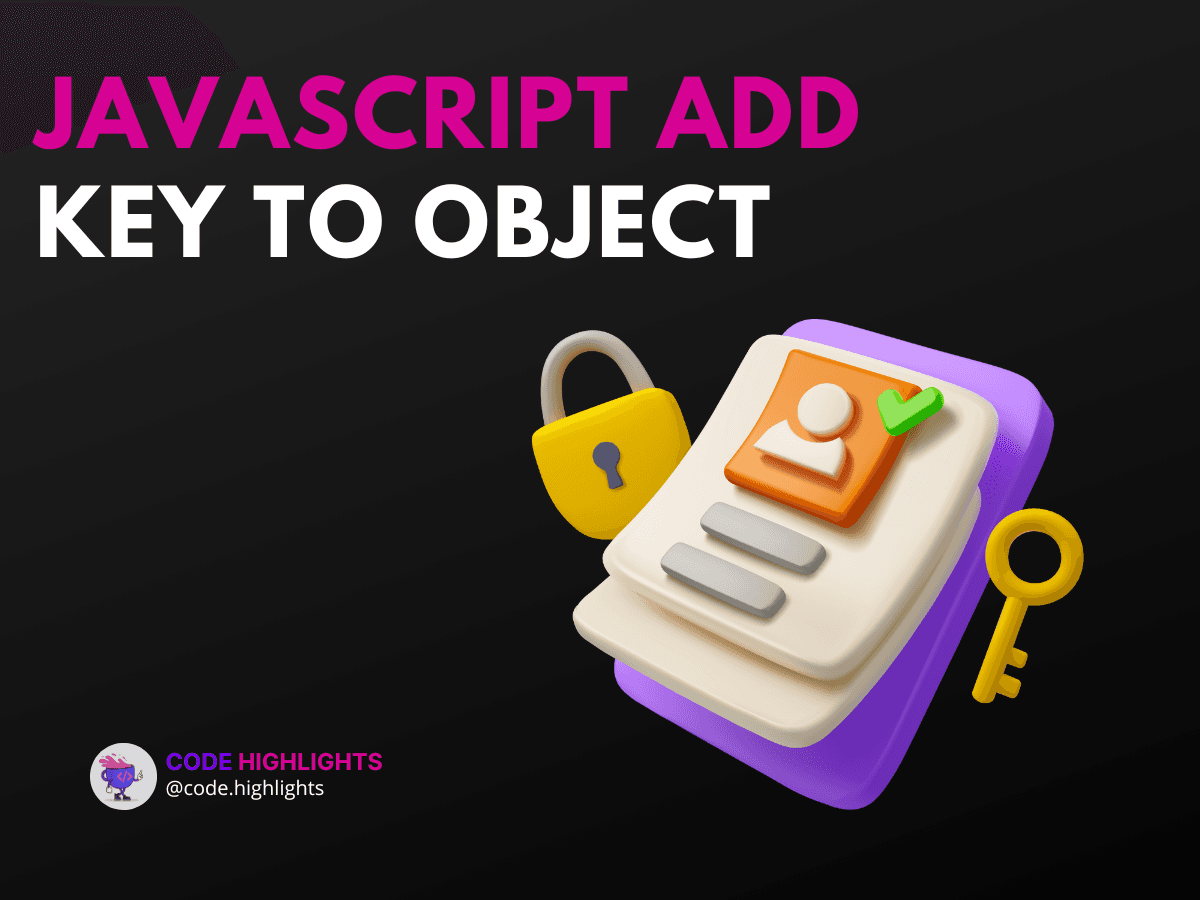
- 1. Using Dot Notation
- Explanation
- 2. Using Bracket Notation
- Explanation
- 3. Using Object.assign()
- Explanation
- 4. Using the Spread Operator
- Explanation
- 5. Using the Object.defineProperty() Method
- Explanation
- 6. Using the Object.keys() Method
- Explanation
- 7. Using JSON.parse() and JSON.stringify()
- Explanation
- Browser Compatibility
- Summary
JavaScript objects are essential for storing data in key-value pairs. Sometimes, you might need to add new keys to an existing object. This can be useful when you want to update information or expand the object's capabilities. In this tutorial, we will explore seven simple ways to JavaScript add key to object.
Here’s a quick example to get us started:
1let person = { name: "Alice", age: 25 };
2person.city = "New York"; // Adding a new key
3console.log(person); // { name: "Alice", age: 25, city: "New York" }
1. Using Dot Notation
The simplest way to add a key is by using dot notation. This method is straightforward and easy to read.
1let car = { brand: "Toyota", model: "Camry" };
2car.year = 2021; // Adding a new key
3console.log(car); // { brand: "Toyota", model: "Camry", year: 2021 }
Explanation
- Syntax:
objectName.keyName = value;
- Parameters:
objectName
: The object you are modifying.keyName
: The name of the new key.value
: The value you want to assign to the new key.
- Return Value: The updated object.
2. Using Bracket Notation
Bracket notation allows you to add keys using strings. This is especially useful when the key name includes spaces or special characters.
1let book = { title: "1984", author: "George Orwell" };
2book["published year"] = 1949; // Adding a new key
3console.log(book); // { title: "1984", author: "George Orwell", published year: 1949 }
Explanation
- Syntax:
objectName["keyName"] = value;
- Parameters: Same as above.
- Return Value: The updated object.
3. Using Object.assign()
Object.assign()
allows you to add multiple keys at once. It merges properties from one or more source objects into a target object.
1let user = { username: "john_doe" };
2Object.assign(user, { email: "john@example.com", age: 30 }); // Adding new keys
3console.log(user); // { username: "john_doe", email: "john@example.com", age: 30 }
Explanation
- Syntax:
Object.assign(target, ...sources);
- Parameters:
target
: The object to which you want to add new keys....sources
: One or more objects containing properties to add.
- Return Value: The target object.
4. Using the Spread Operator
The spread operator (...
) is a modern way to add keys to an object. It creates a new object with all existing keys plus the new ones.
1let device = { type: "laptop", brand: "Dell" };
2device = { ...device, model: "XPS 13" }; // Adding a new key
3console.log(device); // { type: "laptop", brand: "Dell", model: "XPS 13" }
Explanation
- Syntax:
let newObject = { ...existingObject, newKey: value };
- Parameters: Same as above.
- Return Value: A new object with added keys.
5. Using the Object.defineProperty() Method
This method allows you to add a key with specific property attributes, like making it non-enumerable.
1let settings = {};
2Object.defineProperty(settings, "theme", {
3 value: "dark",
4 writable: true,
5 enumerable: true,
6 configurable: true
7}); // Adding a new key
8console.log(settings); // { theme: "dark" }
Explanation
- Syntax:
Object.defineProperty(object, key, descriptor);
- Parameters:
object
: The object to modify.key
: The key name to add.descriptor
: An object that defines the properties of the key.
- Return Value: The modified object.
6. Using the Object.keys() Method
You can also add keys based on existing keys by looping through them with Object.keys()
.
1let student = { name: "John", grade: "A" };
2Object.keys(student).forEach(key => {
3 student[key + "_info"] = `Info about ${student[key]}`; // Adding new keys
4});
5console.log(student); // { name: "John", grade: "A", name_info: "Info about John", grade_info: "Info about A" }
Explanation
- Syntax:
Object.keys(object).forEach(callback);
- Parameters:
object
: The object to iterate over.callback
: A function to execute for each key.
- Return Value: The original object.
7. Using JSON.parse() and JSON.stringify()
This method can be used to create a deep copy of an object and add new keys simultaneously.
1let original = { name: "Emma" };
2let newObject = JSON.parse(JSON.stringify(original));
3newObject.age = 28; // Adding a new key
4console.log(newObject); // { name: "Emma", age: 28 }
Explanation
- Syntax:
JSON.parse(JSON.stringify(object));
- Parameters:
object
: The object to copy.
- Return Value: A new object that can be modified independently.
Browser Compatibility
Most modern browsers, including Chrome, Firefox, and Safari, support all these methods for adding keys to objects. However, older versions of Internet Explorer may not support some ES6 features like the spread operator.
Summary
In this tutorial, we explored seven simple ways to JavaScript add key to object. We covered methods like dot notation, bracket notation, Object.assign()
, the spread operator, Object.defineProperty()
, and more. Each method has its unique use cases, and understanding them will help you manage your objects more effectively.
For more in-depth learning, check out our courses on JavaScript, HTML Fundamentals, and CSS Introduction. If you're just starting with web development, consider our Introduction to Web Development course.
Feel free to dive deeper into each method and explore how they can enhance your coding skills!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
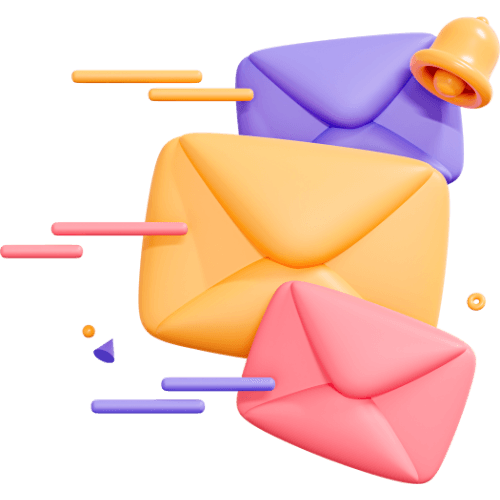
Related articles
9 Articles
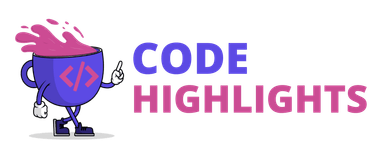
Copyright © Code Highlights 2025.