How to Solve Javascript Anagram Puzzles Efficiently

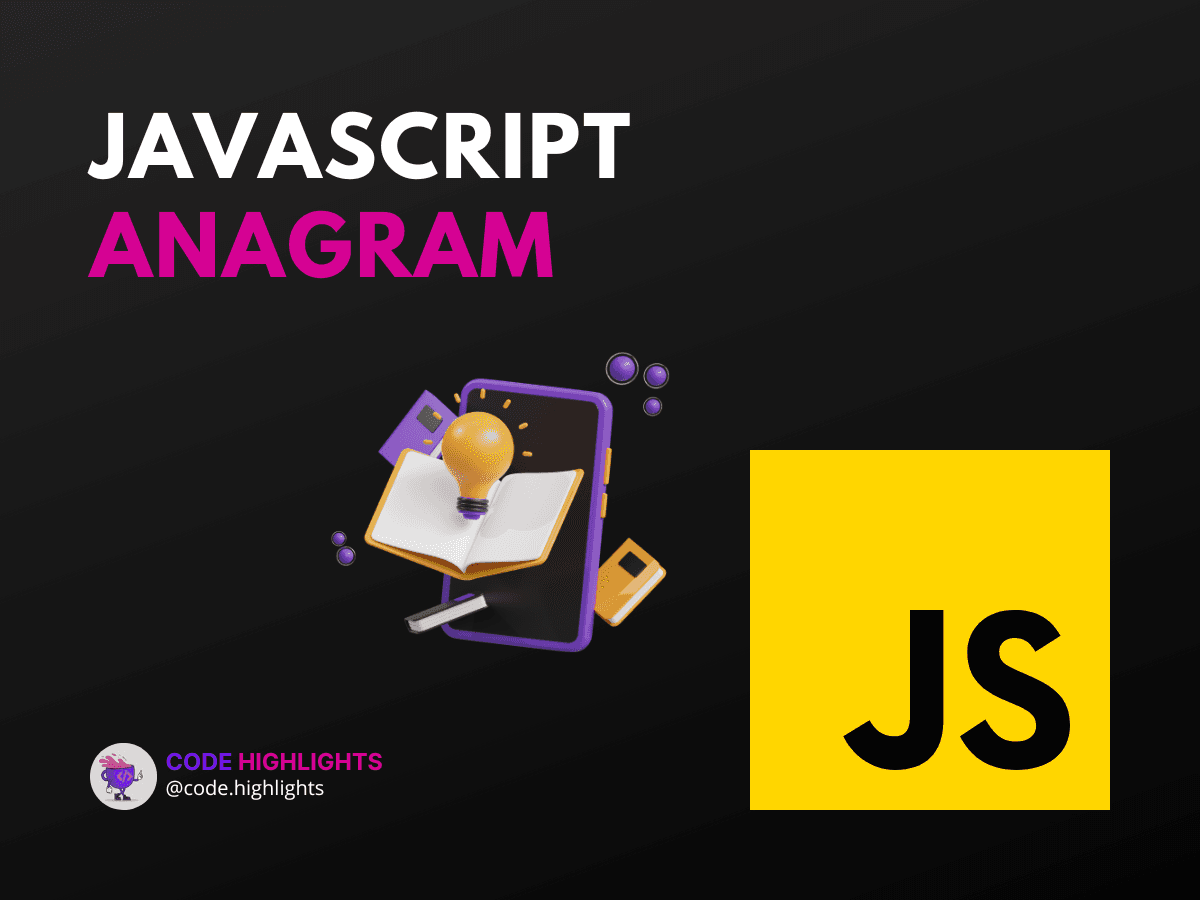
- Step 1: Normalize the Input
- Step 2: Sorting the Characters
- Step 3: Comparing the Strings
- Step 4: Testing Your Function
Anagrams are a timeless puzzle that challenge our ability to recognize patterns and rearrange letters to uncover hidden words. In the digital age, we can harness the power of programming to solve these puzzles, and JavaScript is an excellent tool for this task. This tutorial will guide you through creating an efficient algorithm to tackle any JavaScript anagram challenge.
Before diving into the code, let's briefly understand what an anagram is. An anagram is a word or phrase formed by rearranging the letters of a different word or phrase, typically using all the original letters exactly once. For example, "listen" is an anagram of "silent".
1function isAnagram(str1, str2) {
2 return formatStr(str1) === formatStr(str2);
3}
4
5function formatStr(str) {
6 return str
7 .replace(/[^\w]/g, '')
8 .toLowerCase()
9 .split('')
10 .sort()
11 .join('');
12}
13
14console.log(isAnagram('listen', 'silent')); // true
In the code snippet above, you see a simple introduction to how we might compare two strings to check if they are anagrams in JavaScript. Now, let's break down the steps to create a more comprehensive solution.
Step 1: Normalize the Input
First, we need to ensure that our input strings are in a consistent format. This involves removing any non-alphanumeric characters and converting all letters to lowercase.
Step 2: Sorting the Characters
After normalization, the next step is to sort the characters alphabetically. This will allow us to compare two strings directly to see if they are anagrams.
Step 3: Comparing the Strings
With both strings normalized and sorted, we can now compare them to determine if they are anagrams.
1function areAnagrams(string1, string2) {
2 return sortCharacters(string1) === sortCharacters(string2);
3}
Step 4: Testing Your Function
It's always good practice to test your functions with various inputs to ensure they work as expected.
1console.log(areAnagrams('listen', 'silent')); // true
2console.log(areAnagrams('hello', 'bye')); // false
Through these steps, you've learned the basics of creating a JavaScript function to solve anagram puzzles. If you're looking to deepen your knowledge of JavaScript, consider exploring our JavaScript course.
For those interested in web development, understanding how to manipulate strings is crucial. You might find our HTML fundamentals course and introduction to CSS helpful in building a strong foundation. And for a broader overview, our introduction to web development course can set you on the right path.
To further enhance your understanding of JavaScript anagrams, check out these external resources:
- Mozilla Developer Network (MDN) JavaScript Guide
- JavaScript Algorithms and Data Structures
- W3Schools JavaScript Tutorial
Remember, practice makes perfect. Keep experimenting with different algorithms and optimizing your code for better performance. Happy coding!
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
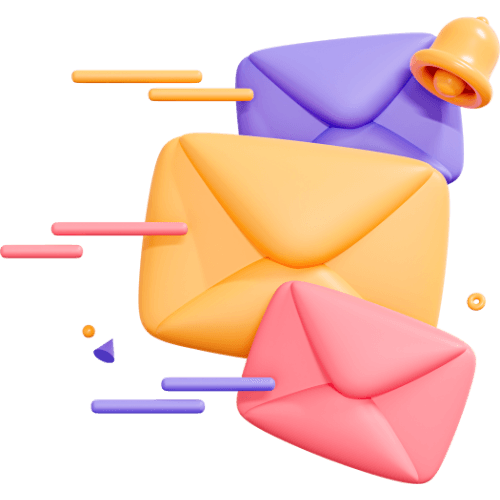
Related articles
9 Articles
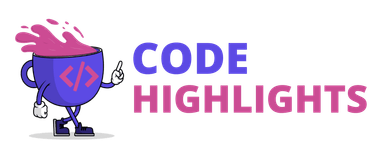
Copyright © Code Highlights 2025.