Why Use Anonymous Functions in JavaScript? Top 3 Reasons!

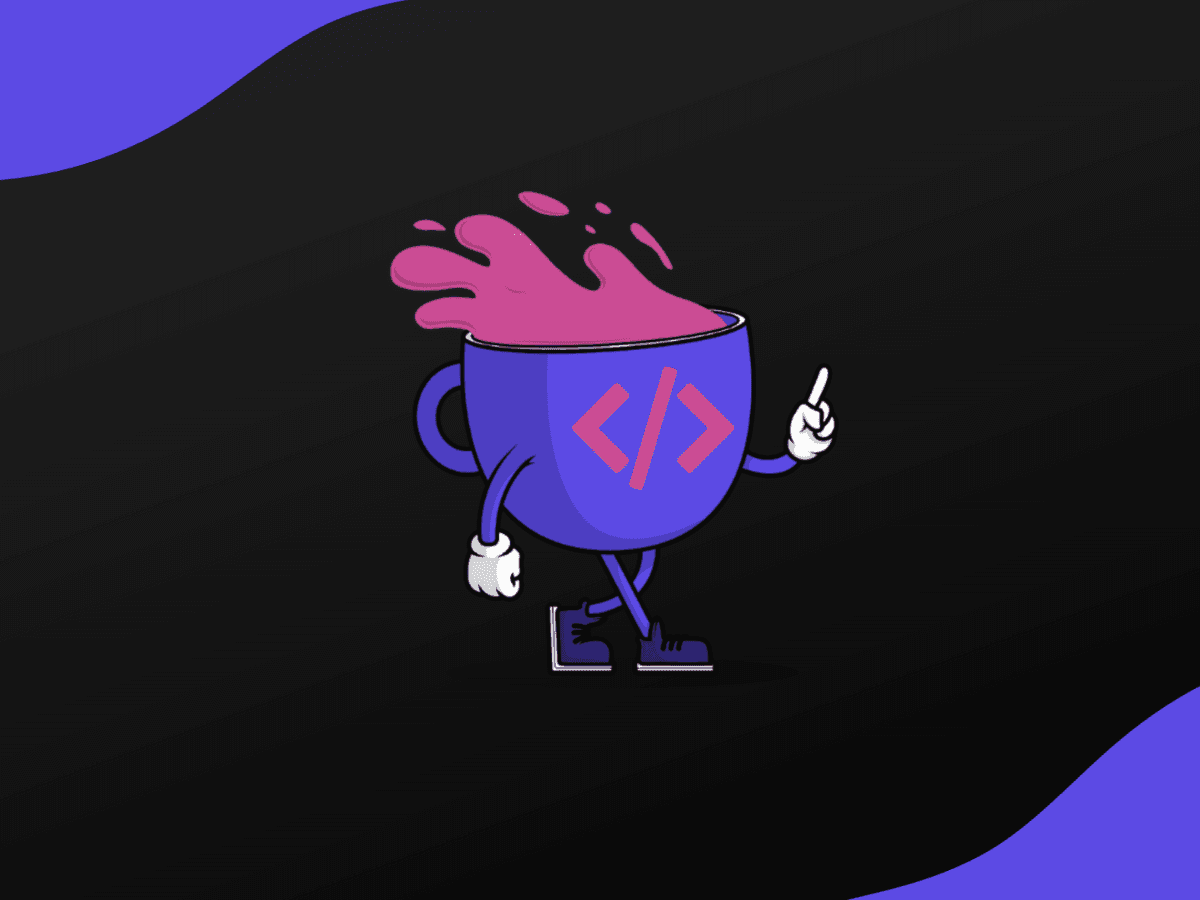
- Defining an Anonymous Function
- Why Anonymous Function in JavaScript?
- Named vs Anonymous Function in JavaScript
- Arrow Functions: The => in JavaScript
- Use Cases for Anonymous Functions in JavaScript
- Further Reading
In JavaScript, functions are objects and can be defined in several ways. One such way is through the use of anonymous functions. An anonymous function is a function without a name. It's often used as an argument to other functions or as an immediately invoked function execution (IIFE). Let's explore more about anonymous functions and their use cases.
Defining an Anonymous Function
1var anonFunc = function() {
2 console.log("This is an anonymous function");
3}
4anonFunc(); // Will log `This is an anonymous function`
In the above example, anonFunc
is a variable that refers to our anonymous function.
Why Anonymous Function in JavaScript?
Anonymous functions are widely used in JavaScript for several reasons. They help to encapsulate code and prevent polluting the global namespace. They're also commonly used in functional programming concepts like closures and callback functions.
Named vs Anonymous Function in JavaScript
A named function has a name when it's defined, while an anonymous function doesn't. Named functions have the advantage of being self-documenting and easier to debug due to their name property.
1function namedFunc() {
2 console.log("This is a named function");
3}
4namedFunc(); // Will log `This is a named function`
Arrow Functions: The =>
in JavaScript
Arrow functions, introduced in ES6, provide a new way of writing concise functions. The =>
symbol is used to define them.
1var arrowFunc = () => {
2 console.log("This is an arrow function");
3}
4arrowFunc(); // Will log `This is an arrow function`
Arrow functions are always anonymous and they handle this
differently than traditional functions.
Use Cases for Anonymous Functions in JavaScript
Anonymous functions are frequently used in event handling, asynchronous programming, and callbacks. They can also be used in IIFEs to create a new scope, protecting variables from the global scope.
1(function() {
2 var x = 20;
3 console.log(x); // Will log `20`
4})();
5console.log(x); // Will log `undefined`
Further Reading
To dive deeper into JavaScript functions, consider the following resources:
- Mozilla Developer Network's guide on functions
- Eloquent JavaScript's chapter on functions
- JavaScript.info's article on arrow functions
Mastering JavaScript functions, including the anonymous ones, is crucial for any web development journey. Keep practicing and exploring different JavaScript concepts to enhance your skills.
Related courses
1 Course
Stay Ahead with Code highlights
Join our community of forward-thinkers and innovators. Subscribe to get the latest updates on courses, exclusive insights, and tips from industry experts directly to your inbox.
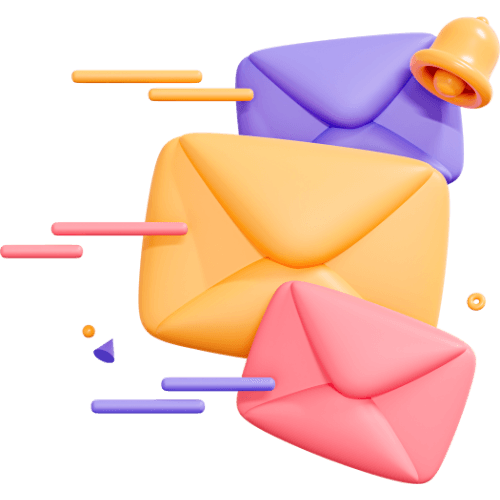
Related articles
9 Articles
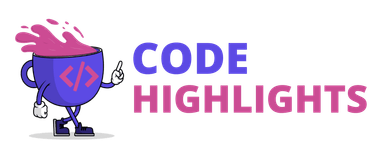
Copyright © Code Highlights 2025.